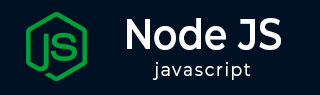
- Node.js 教程
- Node.js - 主页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js - 全局对象
- Node.js - 实用模块
- Node.js - Web 模块
- Node.js - Express 框架
- Node.js - RESTFul API
- Node.js - 扩展应用程序
- Node.js - 打包
- Node.js - 内置模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用的资源
- Node.js - 讨论
Node.js - 响应对象
res对象表示 Express 应用程序收到 HTTP 请求时发送的 HTTP 响应。
响应对象属性
以下是与响应对象关联的几个属性的列表。
先生。 | 属性及说明 |
---|---|
1 |
资源应用程序 此属性保存对使用中间件的 Express 应用程序实例的引用。 |
2 |
res.headers已发送 布尔属性,指示应用程序是否发送响应的 HTTP 标头。 |
3 |
当地人资源 包含作用域为请求的响应局部变量的对象 |
响应对象方法
res.append(字段[,值])
res.append(field [, value])
此方法将指定的值附加到 HTTP 响应标头字段。以下是一些例子 -
res.append('Link', ['<http://localhost/>', '<http://localhost:3000/>']); res.append('Set-Cookie', 'foo=bar; Path=/; HttpOnly'); res.append('Warning', '199 Miscellaneous warning');
res.attachment([文件名])
res.attachment([filename])
此方法用于将文件作为 HTTP 响应中的附件发送。以下是一些例子 -
res.attachment('path/to/logo.png');
res.cookie(名称, 值 [, 选项])
res.cookie(name, value [, options])
该方法用于将cookie名称设置为值。value 参数可以是转换为 JSON 的字符串或对象。以下是一些例子 -
res.cookie('name', 'tobi', { domain: '.example.com', path: '/admin', secure: true }); res.cookie('cart', { items: [1,2,3] }); res.cookie('cart', { items: [1,2,3] }, { maxAge: 900000 });
res.clearCookie(名称[,选项])
res.clearCookie(name [, options])
该方法用于清除指定名称的cookie。以下是一些例子 -
res.cookie('name', 'tobi', { path: '/admin' }); res.clearCookie('name', { path: '/admin' });
res.download(路径[,文件名][,fn])
res.download(path [, filename] [, fn])
此方法用于将路径中的文件作为“附件”传输。通常,浏览器会提示用户下载。以下是一些例子 -
res.download('/report-12345.pdf'); res.download('/report-12345.pdf', 'report.pdf'); res.download('/report-12345.pdf', 'report.pdf', function(err){ });
res.end([数据] [, 编码])
res.end([data] [, encoding])
该方法用于结束响应过程。以下是一些例子 -
res.end(); res.status(404).end();
res.format(对象)
res.format(object)
此方法用于对请求对象上的 Accept HTTP 标头(如果存在)执行内容协商。以下是一些例子 -
res.format ({ 'text/plain': function() { res.send('hey'); }, 'text/html': function() { res.send('hey'); }, 'application/json': function() { res.send({ message: 'hey' }); }, 'default': function() { // log the request and respond with 406 res.status(406).send('Not Acceptable'); } });
res.get(字段)
res.get(field)
该方法用于返回field指定的HTTP响应头。这是一个例子 -
res.get('Content-Type');
res.json([正文])
res.json([body])
该方法用于发送 JSON 响应。以下是一些例子 -
res.json(null) res.json({ user: 'tobi' }) res.status(500).json({ error: 'message' })
res.jsonp([正文])
res.jsonp([body])
此方法用于发送支持 JSONP 的 JSON 响应。以下是一些例子 -
res.jsonp(null) res.jsonp({ user: 'tobi' }) res.status(500).jsonp({ error: 'message' })
res.links(链接)
res.links(links)
此方法用于连接作为参数属性提供的链接,以填充响应的 Link HTTP 标头字段。以下是一些例子 -
res.links ({ next: 'http://api.example.com/users?page=2', last: 'http://api.example.com/users?page=5' });
res.位置(路径)
res.location(path)
该方法用于根据指定的路径参数设置响应的Location HTTP头字段。以下是一些例子 -
res.location('/foo/bar'); res.location('foo/bar'); res.location('http://example.com');
res.redirect([状态,]路径)
res.redirect([status,] path)
该方法用于重定向到从指定路径派生的 URL,并具有指定的 HTTP 状态代码状态。以下是一些例子 -
res.redirect('/foo/bar'); res.redirect('http://example.com'); res.redirect(301, 'http://example.com');
res.render(视图[,局部变量][,回调])
res.render(view [, locals] [, callback])
该方法用于渲染视图并将渲染的 HTML 字符串发送到客户端。以下是一些例子 -
// send the rendered view to the client res.render('index'); // pass a local variable to the view res.render('user', { name: 'Tobi' }, function(err, html) { // ... });
res.send([正文])
res.send([body])
该方法用于发送 HTTP 响应。以下是一些例子 -
res.send(new Buffer('whoop')); res.send({ some: 'json' }); res.send('<p>some html</p>');
res.sendFile(路径[,选项][,fn])
res.sendFile(path [, options] [, fn])
该方法用于传输给定路径下的文件。根据文件名的扩展名设置 Content-Type 响应 HTTP 标头字段。这是一个例子 -
res.sendFile(fileName, options, function (err) { // ... });
res.sendStatus(状态代码)
res.sendStatus(statusCode)
此方法用于将响应 HTTP 状态代码设置为 statusCode 并将其字符串表示形式作为响应正文发送。以下是一些例子 -
res.sendStatus(200); // equivalent to res.status(200).send('OK') res.sendStatus(403); // equivalent to res.status(403).send('Forbidden') res.sendStatus(404); // equivalent to res.status(404).send('Not Found') res.sendStatus(500); // equivalent to res.status(500).send('Internal Server Error')
res.set(字段[,值])
res.set(field [, value])
此方法用于将响应的 HTTP 标头字段设置为值。以下是一些例子 -
res.set('Content-Type', 'text/plain'); res.set ({ 'Content-Type': 'text/plain', 'Content-Length': '123', 'ETag': '12345' })
res.status(代码)
res.status(code)
该方法用于设置响应的 HTTP 状态。以下是一些例子 -
res.status(403).end(); res.status(400).send('Bad Request'); res.status(404).sendFile('/absolute/path/to/404.png');
res.type(类型)
res.type(type)
此方法用于将 Content-Type HTTP 标头设置为 MIME 类型。以下是一些例子 -
res.type('.html'); // => 'text/html' res.type('html'); // => 'text/html' res.type('json'); // => 'application/json' res.type('application/json'); // => 'application/json' res.type('png'); // => image/png: