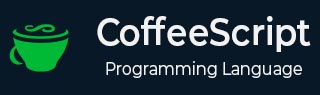
- CoffeeScript 教程
- CoffeeScript - 主页
- CoffeeScript - 概述
- CoffeeScript - 环境
- CoffeeScript - 命令行实用程序
- CoffeeScript - 语法
- CoffeeScript - 数据类型
- CoffeeScript - 变量
- CoffeeScript - 运算符和别名
- CoffeeScript - 条件
- CoffeeScript - 循环
- CoffeeScript - 理解
- CoffeeScript - 函数
- CoffeeScript 面向对象
- CoffeeScript - 字符串
- CoffeeScript - 数组
- CoffeeScript - 对象
- CoffeeScript - 范围
- CoffeeScript - Splat
- CoffeeScript - 日期
- CoffeeScript - 数学
- CoffeeScript - 异常处理
- CoffeeScript - 正则表达式
- CoffeeScript - 类和继承
- CoffeeScript 高级版
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript-MongoDB
- CoffeeScript-SQLite
- CoffeeScript 有用资源
- CoffeeScript - 快速指南
- CoffeeScript - 有用的资源
- CoffeeScript - 讨论
CoffeeScript - 循环
在编码时,您可能会遇到需要一遍又一遍地执行一段代码的情况。在这种情况下,您可以使用循环语句。
一般来说,语句是按顺序执行的:首先执行函数中的第一个语句,然后执行第二个语句,依此类推。
循环语句允许我们多次执行一条语句或一组语句。下面给出的是大多数编程语言中循环语句的一般形式

JavaScript 提供while、for和for..in循环。CoffeeScript 中的循环与 JavaScript 中的循环类似。
while循环及其变体是 CoffeeScript 中唯一的循环结构。CoffeeScript 为您提供了推导式,而不是常用的for循环,这些推导式将在后面的章节中详细讨论。
CoffeeScript 中的 while 循环
while循环是 CoffeeScript 提供的唯一低级循环。它包含一个布尔表达式和一个语句块。只要给定的布尔表达式为 true,while 循环就会重复执行指定的语句块。一旦表达式变为假,循环就会终止。
句法
以下是 CoffeeScript 中while循环的语法。在这里,不需要括号来指定布尔表达式,我们必须使用(一致数量的)空格来缩进循环体,而不是用大括号括起来。
while expression statements to be executed
例子
以下示例演示了CoffeeScript 中while循环的用法。将此代码保存在名为while_loop_example.coffee的文件中
console.log "Starting Loop " count = 0 while count < 10 console.log "Current Count : " + count count++; console.log "Set the variable to different value and then try"
打开命令提示符并编译 .coffee 文件,如下所示。
c:\> coffee -c while_loop_example.coffee
编译时,它会给出以下 JavaScript。
// Generated by CoffeeScript 1.10.0 (function() { var count; console.log("Starting Loop "); count = 0; while (count < 10) { console.log("Current Count : " + count); count++; } console.log("Set the variable to different value and then try"); }).call(this);
现在,再次打开命令提示符并运行 CoffeeScript 文件,如下所示。
c:\> coffee while_loop_example.coffee
执行时,CoffeeScript 文件会产生以下输出。
Starting Loop Current Count : 0 Current Count : 1 Current Count : 2 Current Count : 3 Current Count : 4 Current Count : 5 Current Count : 6 Current Count : 7 Current Count : 8 Current Count : 9 Set the variable to different value and then try
while 的变体
CoffeeScript中的While循环有两种变体,即until变体和loop变体。
编号 | 循环类型和描述 |
---|---|
1 | 直到 while 的变体
while 循环的until变体包含一个布尔表达式和一个代码块。只要给定的布尔表达式为假,就会执行该循环的代码块。 |
2 | while 的循环变体
循环变体相当于具有真值(while true)的 while循环。该循环中的语句将重复执行,直到我们使用Break语句退出循环。 |