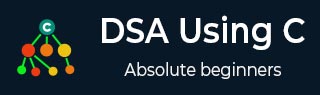
- DSA 使用 C 教程
- 使用 C 的 DSA - 主页
- 使用 C 语言的 DSA - 概述
- 使用 C 语言的 DSA - 环境
- 使用 C 算法的 DSA
- 使用 C 的 DSA - 概念
- 使用 C 数组的 DSA
- 使用 C 链表的 DSA
- 使用 C 的 DSA - 双向链表
- 使用 C 的 DSA - 循环链表
- 使用 C 的 DSA - 堆栈内存溢出
- 使用 C 的 DSA - 解析表达式
- 使用 C 队列的 DSA
- 使用 C 的 DSA - 优先级队列
- 使用 C 树的 DSA
- 使用 C 哈希表的 DSA
- 使用 C 堆的 DSA
- 使用 C - Graph 的 DSA
- 使用 C 搜索技术的 DSA
- 使用 C 排序技术的 DSA
- 使用 C 的 DSA - 递归
- 使用 C 语言的 DSA 有用资源
- 使用 C 的 DSA - 快速指南
- 使用 C 的 DSA - 有用资源
- 使用 C 的 DSA - 讨论
使用 C 数组的 DSA
概述
数组是一个可以容纳固定数量的项目的容器,并且这些项目应该具有相同的类型。大多数数据结构都使用数组来实现其算法。以下是理解数组概念的重要术语。
元素- 存储在数组中的每个项目称为元素。
索引- 数组中元素的每个位置都有一个数字索引,用于标识该元素。
数组表示

根据上图所示,以下是需要考虑的要点。
索引从0开始。
数组长度为8,这意味着它可以存储8个元素。
每个元素都可以通过其索引来访问。例如,我们可以将索引 6 处的元素获取为 9。
基本操作
以下是数组支持的基本操作。
插入- 在给定索引处添加一个元素。
删除- 删除给定索引处的元素。
搜索- 使用给定索引或按值搜索元素。
更新- 更新给定索引处的元素。
在 C 中,当使用大小初始化数组时,它会按以下顺序为其元素分配默认值。
先生编号 | 数据类型 | 默认值 |
---|---|---|
1 | 布尔值 | 错误的 |
2 | 字符 | 0 |
3 | 整数 | 0 |
4 | 漂浮 | 0.0 |
5 | 双倍的 | 0.0f |
6 | 空白 | |
7 | wchar_t | 0 |
例子
#include <stdio.h> #include <string.h> static void display(int intArray[], int length){ int i=0; printf("Array : ["); for(i = 0; i < length; i++) { /* display value of element at index i. */ printf(" %d ", intArray[i]); } printf(" ]\n "); } int main() { int i = 0; /* Declare an array */ int intArray[8]; // initialize elements of array n to 0 for ( i = 0; i < 8; i++ ) { intArray[ i ] = 0; // set elements to default value of 0; } printf("Array with default data."); /* Display elements of an array.*/ display(intArray,8); /* Operation : Insertion Add elements in the array */ for(i = 0; i < 8; i++) { /* place value of i at index i. */ printf("Adding %d at index %d\n",i,i); intArray[i] = i; } printf("\n"); printf("Array after adding data. "); display(intArray,8); /* Operation : Insertion Element at any location can be updated directly */ int index = 5; intArray[index] = 10; printf("Array after updating element at index %d.\n",index); display(intArray,8); /* Operation : Search using index Search an element using index.*/ printf("Data at index %d:%d\n" ,index,intArray[index]); /* Operation : Search using value Search an element using value.*/ int value = 4; for(i = 0; i < 8; i++) { if(intArray[i] == value ){ printf("value %d Found at index %d \n", intArray[i],i); break; } } return 0; }
输出
如果我们编译并运行上面的程序,那么它将产生以下输出 -
Array with default data.Array : [ 0 0 0 0 0 0 0 0 ] Adding 0 at index 0 Adding 1 at index 1 Adding 2 at index 2 Adding 3 at index 3 Adding 4 at index 4 Adding 5 at index 5 Adding 6 at index 6 Adding 7 at index 7 Array after adding data. Array : [ 0 1 2 3 4 5 6 7 ] Array after updating element at index 5. Array : [ 0 1 2 3 4 10 6 7 ] Data at index 5: 10 4 Found at index 4