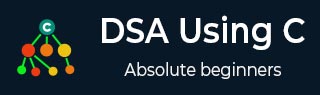
- DSA 使用 C 教程
- 使用 C 的 DSA - 主页
- 使用 C 语言的 DSA - 概述
- 使用 C 语言的 DSA - 环境
- 使用 C 算法的 DSA
- 使用 C 的 DSA - 概念
- 使用 C 数组的 DSA
- 使用 C 链表的 DSA
- 使用 C 的 DSA - 双向链表
- 使用 C 的 DSA - 循环链表
- 使用 C 的 DSA - 堆栈内存溢出
- 使用 C 的 DSA - 解析表达式
- 使用 C 队列的 DSA
- 使用 C 的 DSA - 优先级队列
- 使用 C 树的 DSA
- 使用 C 哈希表的 DSA
- 使用 C 堆的 DSA
- 使用 C - Graph 的 DSA
- 使用 C 搜索技术的 DSA
- 使用 C 排序技术的 DSA
- 使用 C 的 DSA - 递归
- 使用 C 语言的 DSA 有用资源
- 使用 C 的 DSA - 快速指南
- 使用 C 的 DSA - 有用资源
- 使用 C 的 DSA - 讨论
使用 C 的 DSA - 插值搜索
概述
插值搜索是二分搜索的改进变体。该搜索算法作用于所需值的探测位置。为了使该算法正常工作,数据收集应该采用排序的形式。
插值搜索通过计算探针位置来搜索特定项目。最初探针位置是集合中最中间项目的位置。如果发生匹配,则返回项目的索引。如果中间项大于项,则在中间项右侧的子数组中再次计算探测位置,否则在中间项左侧的子数组中搜索项。这个过程也在子数组上继续,直到子数组的大小减少到零。
插值搜索的示例是字典搜索,要搜索从 X 开始的单词,我们会在字典末尾附近进行搜索,从而通过插值探针位置等来进行搜索。
算法
Interpolation Search ( A: array of item, n: total no. of items ,x: item to be searched) Step 1: Set lowerBound = 0 Step 2: Set upperBound = n - 1 Step 3: if lowerBound = upperBound or A[lowerBound] = A[upperBound] go to step 12 Step 4: set midPoint = lowerBound + ((upperBound -lowerBound) / (A[upperBound] - A[lowerBound])) * (x - A[lowerBound]) Step 5: if A[midPoint] < x Step 6: set from = midPoint + 1 Step 7: if A[midPoint] > x Step 8: set to = midPoint - 1 Step 9: if A[midPoint] = x go to step 11 Step 10: Go to Step 3 Step 11: Print Element x Found at index midPoint and go to step 13 Step 12: Print element not found Step 13: Exit
例子
#include <stdio.h> #include <math.h> #define MAX 20 // array of items on which linear search will be conducted. int intArray[MAX] = {1,2,3,4,6,7,9,11,12,14,15,16,17,19,33,34,43,45,55,66}; void printline(int count){ int i; for(i=0;i <count-1;i++){ printf("="); } printf("=\n"); } int find(int data){ int lowerBound = 0; int upperBound = MAX -1; int midPoint = -1; int comparisons = 0; int index = -1; while(lowerBound <= upperBound){ printf("Comparison %d\n" , (comparisons +1) ) ; printf("lowerBound : %d, intArray[%d] = %d\n", lowerBound,lowerBound,intArray[lowerBound]); printf("upperBound : %d, intArray[%d] = %d\n", upperBound,upperBound,intArray[upperBound]); comparisons++; // probe the mid point midPoint = lowerBound + round((double)(upperBound - lowerBound) / (intArray[upperBound] - intArray[lowerBound]) * (data - intArray[lowerBound])); printf("midPoint = %d\n",midPoint); // data found if(intArray[midPoint] == data){ index = midPoint; break; } else { // if data is larger if(intArray[midPoint] < data){ // data is in upper half lowerBound = midPoint + 1; } // data is smaller else{ // data is in lower half upperBound = midPoint -1; } } } printf("Total comparisons made: %d" , comparisons); return index; } void display(){ int i; printf("["); // navigate through all items for(i=0;i<MAX;i++){ printf("%d ",intArray[i]); } printf("]\n"); } int main(){ printf("Input Array: "); display(); printline(50); //find location of 1 int location = find(55); // if element was found if(location != -1) printf("\nElement found at location: %d" ,(location+1)); else printf("Element not found."); }
输出
如果我们编译并运行上面的程序,那么它将产生以下输出 -
Input Array: [1 2 3 4 6 7 9 11 12 14 15 16 17 19 33 34 43 45 55 66 ] ================================================== Comparison 1 lowerBound : 0, intArray[0] = 1 upperBound : 19, intArray[19] = 66 midPoint = 16 Comparison 2 lowerBound : 17, intArray[17] = 45 upperBound : 19, intArray[19] = 66 midPoint = 18 Total comparisons made: 2 Element found at location: 19
dsa_using_c_search_techniques.htm