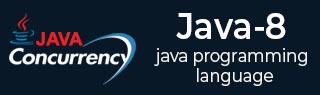
- Java并发教程
- 并发 - 主页
- 并发 - 概述
- 并发 - 环境设置
- 并发-主要操作
- 线程间通信
- 并发-同步
- 并发-死锁
- 实用程序类示例
- 并发-ThreadLocal
- 并发 - ThreadLocalRandom
- 锁示例
- 并发-锁
- 并发-ReadWriteLock
- 并发-条件
- 原子变量示例
- 并发-AtomicInteger
- 并发-AtomicLong
- 并发 - AtomicBoolean
- 并发 - AtomicReference
- 并发 - AtomicIntegerArray
- 并发-AtomicLongArray
- 并发 - AtomicReferenceArray
- 执行器示例
- 并发-执行器
- 并发-ExecutorService
- 预定执行服务
- 线程池示例
- 并发-newFixedThreadPool
- 并发-newCachedThreadPool
- 新的调度线程池
- 新的单线程执行器
- 并发-ThreadPoolExecutor
- 调度线程池执行器
- 高级示例
- 并发 - Futures 和 Callables
- 并发 - Fork-Join 框架
- 并发集合
- 并发-BlockingQueue
- 并发 - ConcurrentMap
- 并发导航地图
- 并发有用的资源
- 并发 - 快速指南
- 并发 - 有用的资源
- 并发 - 讨论
ThreadPoolExecutor 类
java.util.concurrent.ThreadPoolExecutor 是一个 ExecutorService,用于使用可能的多个池线程之一执行每个提交的任务,通常使用 Executors 工厂方法进行配置。它还提供了各种实用方法来检查当前线程统计信息并控制它们。
ThreadPoolExecutor 方法
先生。 | 方法及说明 |
---|---|
1 | protected void afterExecute(Runnable r, Throwable t) 给定 Runnable 执行完成时调用的方法。 |
2 | 无效allowCoreThreadTimeOut(布尔值) 设置策略,控制如果在保持活动时间内没有任务到达,核心线程是否可能超时并终止,并在新任务到达时根据需要进行替换。 |
3 | 布尔值允许CoreThreadTimeOut() 如果此池允许核心线程超时,则返回 true,如果在 keepAlive 时间内没有任务到达则终止,并在新任务到达时根据需要进行替换。 |
4 | boolean waitTermination(长超时, TimeUnit 单位) 阻塞,直到所有任务在关闭请求后完成执行,或者发生超时,或者当前线程被中断,以先发生者为准。 |
5 | protected void beforeExecute(线程 t, Runnable r) 在给定线程中执行给定 Runnable 之前调用的方法。 |
6 | 无效执行(可运行命令) 在将来的某个时间执行给定的任务。 |
7 | 受保护的无效终结() 当不再引用此执行器并且它没有线程时调用关闭。 |
8 | int getActiveCount() 返回正在主动执行任务的线程的大致数量。 |
9 | 长 getCompletedTaskCount() 返回已完成执行的任务的大致总数。 |
10 | int getCorePoolSize() 返回线程的核心数。 |
11 | long getKeepAliveTime(TimeUnit 单位) 返回线程保持活动时间,即超过核心池大小的线程在终止之前可以保持空闲的时间量。 |
12 | int getLargestPoolSize() 返回池中曾经同时存在的最大线程数。 |
13 | int getMaximumPoolSize() 返回允许的最大线程数。 |
14 | int getPoolSize() 返回池中当前的线程数。 |
15 | 阻塞队列 返回此执行器使用的任务队列。 |
15 | RejectedExecutionHandler getRejectedExecutionHandler() 返回不可执行任务的当前处理程序。 |
16 | 长 getTaskCount() 返回已计划执行的任务的大致总数。 |
17 号 | ThreadFactory getThreadFactory() 返回用于创建新线程的线程工厂。 |
18 | 布尔 isShutdown() 如果此执行器已关闭,则返回 true。 |
19 | 布尔值已终止() 如果关闭后所有任务均已完成,则返回 true。 |
20 | 布尔 isTerminate() 如果此执行器在 shutdown() 或 shutdownNow() 之后正在终止但尚未完全终止,则返回 true。 |
21 | int prestartAllCoreThreads() 启动所有核心线程,导致它们空闲等待工作。 |
22 | 布尔预启动CoreThread() 启动一个核心线程,使其闲置等待工作。 |
23 | 无效清除() 尝试从工作队列中删除所有已取消的 Future 任务。 |
24 | 布尔删除(可运行任务) 如果该任务存在,则从执行程序的内部队列中删除该任务,从而导致它在尚未启动时无法运行。 |
25 | 无效 setCorePoolSize(int corePoolSize) 设置核心线程数。 |
26 | void setKeepAliveTime(长时间, TimeUnit 单位) 设置线程在终止之前可以保持空闲的时间限制。 |
27 | void setMaximumPoolSize(int maxPoolSize) 设置允许的最大线程数。 |
28 | 无效setRejectedExecutionHandler(RejectedExecutionHandler处理程序) 为无法执行的任务设置新的处理程序。 |
29 | void setThreadFactory(ThreadFactory threadFactory) 设置用于创建新线程的线程工厂。 |
30 | 无效关闭() 启动有序关闭,其中执行先前提交的任务,但不会接受新任务。 |
31 | 列表<Runnable> shutdownNow() 尝试停止所有正在执行的任务,停止正在等待的任务的处理,并返回正在等待执行的任务的列表。 |
32 | 受保护无效终止() 当执行器终止时调用的方法。 |
33 | 字符串转字符串() 返回标识此池及其状态的字符串,包括运行状态的指示以及估计的工作人员和任务计数。 |
例子
以下 TestThread 程序显示了 ThreadPoolExecutor 接口在基于线程的环境中的用法。
import java.util.concurrent.Executors; import java.util.concurrent.ThreadPoolExecutor; import java.util.concurrent.TimeUnit; public class TestThread { public static void main(final String[] arguments) throws InterruptedException { ThreadPoolExecutor executor = (ThreadPoolExecutor)Executors.newCachedThreadPool(); //Stats before tasks execution System.out.println("Largest executions: " + executor.getLargestPoolSize()); System.out.println("Maximum allowed threads: " + executor.getMaximumPoolSize()); System.out.println("Current threads in pool: " + executor.getPoolSize()); System.out.println("Currently executing threads: " + executor.getActiveCount()); System.out.println("Total number of threads(ever scheduled): " + executor.getTaskCount()); executor.submit(new Task()); executor.submit(new Task()); //Stats after tasks execution System.out.println("Core threads: " + executor.getCorePoolSize()); System.out.println("Largest executions: " + executor.getLargestPoolSize()); System.out.println("Maximum allowed threads: " + executor.getMaximumPoolSize()); System.out.println("Current threads in pool: " + executor.getPoolSize()); System.out.println("Currently executing threads: " + executor.getActiveCount()); System.out.println("Total number of threads(ever scheduled): " + executor.getTaskCount()); executor.shutdown(); } static class Task implements Runnable { public void run() { try { Long duration = (long) (Math.random() * 5); System.out.println("Running Task! Thread Name: " + Thread.currentThread().getName()); TimeUnit.SECONDS.sleep(duration); System.out.println("Task Completed! Thread Name: " + Thread.currentThread().getName()); } catch (InterruptedException e) { e.printStackTrace(); } } } }
这将产生以下结果。
输出
Largest executions: 0 Maximum allowed threads: 2147483647 Current threads in pool: 0 Currently executing threads: 0 Total number of threads(ever scheduled): 0 Core threads: 0 Largest executions: 2 Maximum allowed threads: 2147483647 Current threads in pool: 2 Currently executing threads: 2 Total number of threads(ever scheduled): 2 Running Task! Thread Name: pool-1-thread-2 Running Task! Thread Name: pool-1-thread-1 Task Completed! Thread Name: pool-1-thread-1 Task Completed! Thread Name: pool-1-thread-2