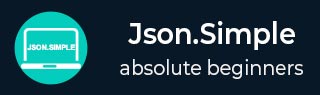
- JSON.simple 教程
- JSON.simple - 主页
- JSON.simple - 概述
- JSON.simple - 环境设置
- JSON.simple - JAVA 映射
- 解码示例
- 转义特殊字符
- JSON.simple - 使用 JSONValue
- JSON.simple - 异常处理
- JSON.simple - 容器工厂
- JSON.simple - 内容处理程序
- 编码示例
- JSON.simple - 编码 JSONObject
- JSON.simple - 编码 JSONArray
- 合并示例
- JSON.simple - 合并对象
- JSON.simple - 合并数组
- 组合示例
- JSON.simple - 原始、对象、数组
- JSON.simple - 原始、映射、列表
- 基元、对象、映射、列表
- 定制示例
- JSON.simple - 自定义输出
- 定制输出流
- JSON.simple 有用资源
- JSON.simple - 快速指南
- JSON.simple - 有用的资源
- JSON.simple - 讨论
JSON.simple - 快速指南
JSON.simple - 概述
JSON.simple是一个简单的基于 Java 的 JSON 工具包。您可以使用 JSON.simple 对 JSON 数据进行编码或解码。
特征
符合规范- JSON.simple 完全符合 JSON 规范 - RFC4627。
轻量级- 它有很少的类,并提供必要的功能,如编码/解码和转义 json。
重用集合- 大多数操作都是使用 Map/List 接口完成的,增加了可重用性。
支持流式传输- 支持 JSON 输出文本的流式传输。
SAX like Content Handler - 提供类似 SAX 的接口来传输大量 JSON 数据。
高性能- 使用基于堆的解析器并提供高性能。
无依赖性- 无外部库依赖性。可以独立包含。
JDK1.2 兼容- 源代码和二进制文件与 JDK1.2 兼容
JSON.simple - 环境设置
本地环境设置
JSON.simple 是一个 Java 库,因此第一个要求是在您的计算机上安装 JDK。
系统要求
JDK | 记忆 | 磁盘空间 | 操作系统 |
---|---|---|---|
1.5或以上。 | 没有最低要求。 | 没有最低要求。 | 没有最低要求。 |
第 1 步:验证计算机中的 Java 安装
首先,打开控制台并根据您正在使用的操作系统执行java命令。
操作系统 | 任务 | 命令 |
---|---|---|
Windows | 打开命令控制台 | c:\> java -版本 |
Linux | 打开命令终端 | $ java -版本 |
苹果 | 打开终端 | 机器:< joseph$ java -版本 |
让我们验证所有操作系统的输出 -
操作系统 | 输出 |
---|---|
Windows | java版本“1.8.0_101” Java(TM) SE 运行时环境(版本 1.8.0_101) |
Linux | java版本“1.8.0_101” Java(TM) SE 运行时环境(版本 1.8.0_101) |
苹果 | java版本“1.8.0_101” Java(TM) SE 运行时环境(版本 1.8.0_101) |
如果您的系统上没有安装 Java,请从以下链接www.oracle.com下载 Java 软件开发工具包 (SDK) 。我们假设 Java 1.8.0_101 作为本教程的安装版本。
第二步:设置JAVA环境
设置JAVA_HOME环境变量以指向计算机上安装 Java 的基本目录位置。例如。
操作系统 | 输出 |
---|---|
Windows | 设置环境变量JAVA_HOME为C:\Program Files\Java\jdk1.8.0_101 |
Linux | 导出 JAVA_HOME = /usr/local/java-current |
苹果 | 导出 JAVA_HOME = /Library/Java/Home |
将 Java 编译器位置附加到系统路径。
操作系统 | 输出 |
---|---|
Windows | 将字符串C:\Program Files\Java\jdk1.8.0_101\bin添加到系统变量Path的末尾。 |
Linux | 导出路径 = $PATH:$JAVA_HOME/bin/ |
苹果 | 不需要 |
如上所述,使用命令java -version验证 Java 安装。
第 3 步:下载 JSON.simple 存档
从json-simple @ MVNRepository下载最新版本的 JSON.simple jar 文件。在编写本教程时,我们已经下载了 json-simple-1.1.1.jar,并将其复制到 C:\>JSON 文件夹中。
操作系统 | 档案名称 |
---|---|
Windows | json-simple-1.1.1.jar |
Linux | json-simple-1.1.1.jar |
苹果 | json-simple-1.1.1.jar |
第四步:设置JSON_JAVA环境
将JSON_JAVA环境变量设置为指向计算机上存储 JSON.simple jar 的基本目录位置。假设我们已将 json-simple-1.1.1.jar 存储在 JSON 文件夹中。
先生编号 | 操作系统和描述 |
---|---|
1 | Windows 将环境变量 JSON_JAVA 设置为 C:\JSON |
2 | Linux 导出 JSON_JAVA = /usr/local/JSON |
3 | 苹果 导出 JSON_JAVA = /Library/JSON |
第 5 步:设置 CLASSPATH 变量
将CLASSPATH环境变量设置为指向 JSON.simple jar 位置。
先生编号 | 操作系统和描述 |
---|---|
1 | Windows 设置环境变量CLASSPATH为%CLASSPATH%;%JSON_JAVA%\json-simple-1.1.1.jar;.; |
2 | Linux 导出 CLASSPATH = $CLASSPATH:$JSON_JAVA/json-simple-1.1.1.jar:。 |
3 | 苹果 导出 CLASSPATH = $CLASSPATH:$JSON_JAVA/json-simple-1.1.1.jar:。 |
JSON.simple - JAVA 映射
JSON.simple 在解码或解析时将实体从左侧映射到右侧,在编码时将实体从右侧映射到左侧。
JSON | 爪哇 |
---|---|
细绳 | java.lang.String |
数字 | java.lang.Number |
真|假 | java.lang.布尔值 |
无效的 | 无效的 |
大批 | java.util.List |
目的 | java.util.Map |
解码时, java.util.List的默认具体类是org.json.simple.JSONArray , java.util.Map的默认具体类是org.json.simple.JSONObject。
JSON.simple - 转义特殊字符
以下字符是保留字符,不能在 JSON 中使用,必须正确转义才能在字符串中使用。
退格键替换为 \b
换页符替换为 \f
换行符替换为\n
回车符替换为\r
制表符替换为 \t
双引号替换为\"
反斜杠替换为 \\
JSONObject.escape()方法可用于转义 JSON 字符串中的此类保留关键字。以下是示例 -
例子
import org.json.simple.JSONObject; public class JsonDemo { public static void main(String[] args) { JSONObject jsonObject = new JSONObject(); String text = "Text with special character /\"\'\b\f\t\r\n."; System.out.println(text); System.out.println("After escaping."); text = jsonObject.escape(text); System.out.println(text); } }
输出
Text with special character /"' . After escaping. Text with special character \/\"'\b\f\t\r\n.
JSON.simple - 使用 JSONValue
JSONValue 提供静态方法 parse() 来解析给定的 json 字符串以返回 JSONObject,然后可以使用该 JSONObject 来获取解析的值。请参阅下面的示例。
例子
import org.json.simple.JSONArray; import org.json.simple.JSONObject; import org.json.simple.JSONValue; public class JsonDemo { public static void main(String[] args) { String s = "[0,{\"1\":{\"2\":{\"3\":{\"4\":[5,{\"6\":7}]}}}}]"; Object obj = JSONValue.parse(s); JSONArray array = (JSONArray)obj; System.out.println("The 2nd element of array"); System.out.println(array.get(1)); System.out.println(); JSONObject obj2 = (JSONObject)array.get(1); System.out.println("Field \"1\""); System.out.println(obj2.get("1")); s = "{}"; obj = JSONValue.parse(s); System.out.println(obj); s = "[5,]"; obj = JSONValue.parse(s); System.out.println(obj); s = "[5,,2]"; obj = JSONValue.parse(s); System.out.println(obj); } }
输出
The 2nd element of array {"1":{"2":{"3":{"4":[5,{"6":7}]}}}} Field "1" {"2":{"3":{"4":[5,{"6":7}]}}} {} [5] [5,2]
JSON.simple - 异常处理
如果 JSON 无效,JSONParser.parse() 会抛出 ParseException。以下示例展示了如何处理 ParseException。
例子
import org.json.simple.parser.JSONParser; import org.json.simple.parser.ParseException; class JsonDemo { public static void main(String[] args) { JSONParser parser = new JSONParser(); String text = "[[null, 123.45, \"a\tb c\"]}, true"; try{ Object obj = parser.parse(text); System.out.println(obj); }catch(ParseException pe) { System.out.println("position: " + pe.getPosition()); System.out.println(pe); } } }
输出
position: 24 Unexpected token RIGHT BRACE(}) at position 24.
JSON.simple - 容器工厂
ContainerFactory 可用于为解析的 JSON 对象/数组创建自定义容器。首先我们需要创建一个 ContainerFactory 对象,然后在 JSONParser 的 parse 方法中使用它来获取所需的对象。请参阅下面的示例 -
例子
import java.util.LinkedHashMap; import java.util.LinkedList; import java.util.List; import java.util.Map; import org.json.simple.parser.ContainerFactory; import org.json.simple.parser.JSONParser; import org.json.simple.parser.ParseException; class JsonDemo { public static void main(String[] args) { JSONParser parser = new JSONParser(); String text = "{\"first\": 123, \"second\": [4, 5, 6], \"third\": 789}"; ContainerFactory containerFactory = new ContainerFactory() { @Override public Map createObjectContainer() { return new LinkedHashMap<>(); } @Override public List creatArrayContainer() { return new LinkedList<>(); } }; try { Map map = (Map)parser.parse(text, containerFactory); map.forEach((k,v)->System.out.println("Key : " + k + " Value : " + v)); } catch(ParseException pe) { System.out.println("position: " + pe.getPosition()); System.out.println(pe); } } }
输出
Key : first Value : 123 Key : second Value : [4, 5, 6] Key : third Value : 789
JSON.simple - ContentHandler
ContentHandler 接口用于提供类似 SAX 的接口来流式传输大型 json。它还提供可停止的能力。下面的例子说明了这个概念。
例子
import java.io.IOException; import java.util.List; import java.util.Stack; import org.json.simple.JSONArray; import org.json.simple.JSONObject; import org.json.simple.parser.ContentHandler; import org.json.simple.parser.JSONParser; import org.json.simple.parser.ParseException; class JsonDemo { public static void main(String[] args) { JSONParser parser = new JSONParser(); String text = "{\"first\": 123, \"second\": [4, 5, 6], \"third\": 789}"; try { CustomContentHandler handler = new CustomContentHandler(); parser.parse(text, handler,true); } catch(ParseException pe) { } } } class CustomContentHandler implements ContentHandler { @Override public boolean endArray() throws ParseException, IOException { System.out.println("inside endArray"); return true; } @Override public void endJSON() throws ParseException, IOException { System.out.println("inside endJSON"); } @Override public boolean endObject() throws ParseException, IOException { System.out.println("inside endObject"); return true; } @Override public boolean endObjectEntry() throws ParseException, IOException { System.out.println("inside endObjectEntry"); return true; } public boolean primitive(Object value) throws ParseException, IOException { System.out.println("inside primitive: " + value); return true; } @Override public boolean startArray() throws ParseException, IOException { System.out.println("inside startArray"); return true; } @Override public void startJSON() throws ParseException, IOException { System.out.println("inside startJSON"); } @Override public boolean startObject() throws ParseException, IOException { System.out.println("inside startObject"); return true; } @Override public boolean startObjectEntry(String key) throws ParseException, IOException { System.out.println("inside startObjectEntry: " + key); return true; } }
输出
inside startJSON inside startObject inside startObjectEntry: first inside primitive: 123 inside endObjectEntry inside startObjectEntry: second inside startArray inside primitive: 4 inside primitive: 5 inside primitive: 6 inside endArray inside endObjectEntry inside startObjectEntry: third inside primitive: 789 inside endObjectEntry inside endObject inside endJSON
JSON.simple - 编码 JSONObject
使用 JSON.simple,我们可以使用以下方式对 JSON 对象进行编码 -
将 JSON 对象编码为字符串- 简单编码。
编码 JSON 对象 - 流式传输- 输出可用于流式传输。
对 JSON 对象进行编码 - 使用映射- 通过保留顺序进行编码。
对 JSON 对象进行编码 - 使用映射和流式传输- 通过保留顺序和流式传输进行编码。
以下示例说明了上述概念。
例子
import java.io.IOException; import java.io.StringWriter; import java.util.LinkedHashMap; import java.util.Map; import org.json.simple.JSONObject; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { JSONObject obj = new JSONObject(); String jsonText; obj.put("name", "foo"); obj.put("num", new Integer(100)); obj.put("balance", new Double(1000.21)); obj.put("is_vip", new Boolean(true)); jsonText = obj.toString(); System.out.println("Encode a JSON Object - to String"); System.out.print(jsonText); StringWriter out = new StringWriter(); obj.writeJSONString(out); jsonText = out.toString(); System.out.println("\nEncode a JSON Object - Streaming"); System.out.print(jsonText); Map obj1 = new LinkedHashMap(); obj1.put("name", "foo"); obj1.put("num", new Integer(100)); obj1.put("balance", new Double(1000.21)); obj1.put("is_vip", new Boolean(true)); jsonText = JSONValue.toJSONString(obj1); System.out.println("\nEncode a JSON Object - Preserving Order"); System.out.print(jsonText); out = new StringWriter(); JSONValue.writeJSONString(obj1, out); jsonText = out.toString(); System.out.println("\nEncode a JSON Object - Preserving Order and Stream"); System.out.print(jsonText); } }
输出
Encode a JSON Object - to String {"balance":1000.21,"is_vip":true,"num":100,"name":"foo"} Encode a JSON Object - Streaming {"balance":1000.21,"is_vip":true,"num":100,"name":"foo"} Encode a JSON Object - Preserving Order {"name":"foo","num":100,"balance":1000.21,"is_vip":true} Encode a JSON Object - Preserving Order and Stream {"name":"foo","num":100,"balance":1000.21,"is_vip":true}
JSON.simple - 编码 JSONArray
使用 JSON.simple,我们可以使用以下方式对 JSON 数组进行编码 -
将 JSON 数组编码为字符串- 简单编码。
编码 JSON 数组 - 流式传输- 输出可用于流式传输。
对 JSON 数组进行编码 - 使用列表- 使用列表进行编码。
对 JSON 数组进行编码 - 使用列表和流式传输- 使用列表和流式传输进行编码。
以下示例说明了上述概念。
例子
import java.io.IOException; import java.io.StringWriter; import java.util.LinkedList; import java.util.List; import org.json.simple.JSONArray; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray list = new JSONArray(); String jsonText; list.add("foo"); list.add(new Integer(100)); list.add(new Double(1000.21)); list.add(new Boolean(true)); list.add(null); jsonText = list.toString(); System.out.println("Encode a JSON Array - to String"); System.out.print(jsonText); StringWriter out = new StringWriter(); list.writeJSONString(out); jsonText = out.toString(); System.out.println("\nEncode a JSON Array - Streaming"); System.out.print(jsonText); List list1 = new LinkedList(); list1.add("foo"); list1.add(new Integer(100)); list1.add(new Double(1000.21)); list1.add(new Boolean(true)); list1.add(null); jsonText = JSONValue.toJSONString(list1); System.out.println("\nEncode a JSON Array - Using List"); System.out.print(jsonText); out = new StringWriter(); JSONValue.writeJSONString(list1, out); jsonText = out.toString(); System.out.println("\nEncode a JSON Array - Using List and Stream"); System.out.print(jsonText); } }
输出
Encode a JSON Array - to String ["foo",100,1000.21,true,null] Encode a JSON Array - Streaming ["foo",100,1000.21,true,null] Encode a JSON Array - Using List ["foo",100,1000.21,true,null] Encode a JSON Array - Using List and Stream ["foo",100,1000.21,true,null]
JSON.simple - 合并对象
在 JSON.simple 中,我们可以使用 JSONObject.putAll() 方法轻松合并两个 JSON 对象。
下面的例子说明了上述概念。
例子
import java.io.IOException; import org.json.simple.JSONObject; class JsonDemo { public static void main(String[] args) throws IOException { JSONObject obj1 = new JSONObject(); obj1.put("name", "foo"); obj1.put("num", new Integer(100)); JSONObject obj2 = new JSONObject(); obj2.put("balance", new Double(1000.21)); obj2.put("is_vip", new Boolean(true)); obj1.putAll(obj2); System.out.println(obj1); } }
输出
{"balance":1000.21,"is_vip":true,"num":100,"name":"foo"}
JSON.simple - 合并数组
在 JSON.simple 中,我们可以使用 JSONArray.addAll() 方法轻松合并两个 JSON 数组。
下面的例子说明了上述概念。
例子
import java.io.IOException; import org.json.simple.JSONArray; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray list1 = new JSONArray(); list1.add("foo"); list1.add(new Integer(100)); JSONArray list2 = new JSONArray(); list2.add(new Double(1000.21)); list2.add(new Boolean(true)); list2.add(null); list1.addAll(list2); System.out.println(list1); } }
输出
["foo",100,1000.21,true,null]
JSON.simple - 原始、对象、数组
使用 JSONArray 对象,我们可以创建一个由原语、对象和数组组成的 JSON。
下面的例子说明了上述概念。
例子
import java.io.IOException; import org.json.simple.JSONArray; import org.json.simple.JSONObject; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray list1 = new JSONArray(); list1.add("foo"); list1.add(new Integer(100)); JSONArray list2 = new JSONArray(); list2.add(new Double(1000.21)); list2.add(new Boolean(true)); list2.add(null); JSONObject obj = new JSONObject(); obj.put("name", "foo"); obj.put("num", new Integer(100)); obj.put("balance", new Double(1000.21)); obj.put("is_vip", new Boolean(true)); obj.put("list1", list1); obj.put("list2", list2); System.out.println(obj); } }
输出
{"list1":["foo",100],"balance":1000.21,"is_vip":true,"num":100,"list2":[1000.21,true,null],"name":"foo"}
JSON.simple - 原始、映射、列表组合
使用 JSONValue 对象,我们可以创建一个由原语、Map 和 List 组成的 JSON。
下面的例子说明了上述概念。
例子
import java.io.IOException; import java.util.LinkedHashMap; import java.util.LinkedList; import java.util.List; import java.util.Map; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { Map m1 = new LinkedHashMap(); m1.put("k11","v11"); m1.put("k12","v12"); m1.put("k13", "v13"); List l1 = new LinkedList(); l1.add(m1); l1.add(new Integer(100)); String jsonString = JSONValue.toJSONString(l1); System.out.println(jsonString); } }
输出
[{"k11":"v11","k12":"v12","k13":"v13"},100]
JSON.simple - 原始、对象、映射、列表
使用 JSONValue 对象,我们可以创建一个由原语、对象、映射和列表组成的 JSON。
下面的例子说明了上述概念。
例子
import java.io.IOException; import java.util.LinkedHashMap; import java.util.LinkedList; import java.util.List; import java.util.Map; import org.json.simple.JSONObject; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { JSONObject obj = new JSONObject(); Map m1 = new LinkedHashMap(); m1.put("k11","v11"); m1.put("k12","v12"); m1.put("k13", "v13"); List l1 = new LinkedList(); l1.add(new Integer(100)); obj.put("m1", m1); obj.put("l1", l1); String jsonString = JSONValue.toJSONString(obj); System.out.println(jsonString); } }
输出
{"m1":{"k11":"v11","k12":"v12","k13":"v13"},"l1":[100]}
JSON.simple - 自定义输出
我们可以根据自定义类自定义 JSON 输出。唯一的要求是实现 JSONAware 接口。
下面的例子说明了上述概念。
例子
import java.io.IOException; import org.json.simple.JSONArray; import org.json.simple.JSONAware; import org.json.simple.JSONObject; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray students = new JSONArray(); students.add(new Student(1,"Robert")); students.add(new Student(2,"Julia")); System.out.println(students); } } class Student implements JSONAware { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toJSONString() { StringBuilder sb = new StringBuilder(); sb.append("{"); sb.append("name"); sb.append(":"); sb.append("\"" + JSONObject.escape(name) + "\""); sb.append(","); sb.append("rollNo"); sb.append(":"); sb.append(rollNo); sb.append("}"); return sb.toString(); } }
输出
[{name:"Robert",rollNo:1},{name:"Julia",rollNo:2}]
JSON.simple - 定制输出流
我们可以根据自定义类自定义 JSON 流输出。唯一的要求是实现 JSONStreamAware 接口。
下面的例子说明了上述概念。
例子
import java.io.IOException; import java.io.StringWriter; import java.io.Writer; import java.util.LinkedHashMap; import java.util.Map; import org.json.simple.JSONArray; import org.json.simple.JSONStreamAware; import org.json.simple.JSONValue; class JsonDemo { public static void main(String[] args) throws IOException { JSONArray students = new JSONArray(); students.add(new Student(1,"Robert")); students.add(new Student(2,"Julia")); StringWriter out = new StringWriter(); students.writeJSONString(out); System.out.println(out.toString()); } } class Student implements JSONStreamAware { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public void writeJSONString(Writer out) throws IOException { Map obj = new LinkedHashMap(); obj.put("name", name); obj.put("rollNo", new Integer(rollNo)); JSONValue.writeJSONString(obj, out); } }
输出
[{name:"Robert",rollNo:1},{name:"Julia",rollNo:2}]