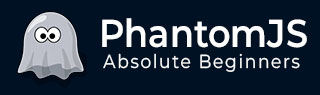
- 文件系统模块
- PhantomJS - 属性
- PhantomJS - 方法
- 系统模块
- PhantomJS - 属性
- 网络服务器模块
- PhantomJS - 属性
- PhantomJS - 方法
- 各种各样的
- 命令行界面
- PhantomJS - 屏幕截图
- PhantomJS - 页面自动化
- PhantomJS - 网络监控
- PhantomJS - 测试
- PhantomJS-REPL
- PhantomJS - 示例
- PhantomJS 有用资源
- PhantomJS - 快速指南
- PhantomJS - 有用的资源
- PhantomJS - 讨论
PhantomJS - 方法
PhantomJS 是一个无需浏览器即可帮助执行 JavaScript 的平台。为此,使用以下方法,有助于添加 Cookie、删除、清除、退出脚本、注入 JS 等。
我们将在本章中详细讨论这些 PhantomJS 方法及其语法。网页模块中也有类似的方法addcookie、injectjs ,这些方法将在后续章节中讨论。
PhantomJS 公开了以下方法,可以帮助我们在没有浏览器的情况下执行 JavaScript -
- 添加Cookie
- 清除Cookie
- 删除Cookie
- 出口
- 注入JS
现在让我们通过示例详细了解这些方法。
添加Cookie
addcookie方法用于添加cookie并存储在数据中。它与浏览器存储它的方式类似。它需要一个参数,该参数是一个具有 cookie 所有属性的对象,其语法如下所示 -
句法
其语法如下 -
phantom.addCookie ({ "name" : "cookie_name", "value" : "cookie_value", "domain" : "localhost" });
名称、值、域是要添加到 addcookie 函数中的强制属性。如果 cookie 对象中缺少任何此属性,则此方法将失败。
name - 指定 cookie 的名称。
value - 指定要使用的 cookie 的值。
域- cookie 将应用到的域。
例子
这是addcookie方法的示例。
var page = require('webpage').create(),url = 'http://localhost/tasks/a.html'; page.open(url, function(status) { if (status === 'success') { phantom.addCookie({ //add name cookie1 with value = 1 name: 'cookie1', value: '1', domain: 'localhost' }); phantom.addCookie({ // add cookie2 with value 2 name: 'cookie2', value: '2', domain: 'localhost' }); phantom.addCookie({ // add cookie3 with value 3 name: 'cookie3', value: '3', domain: 'localhost' }); console.log('Added 3 cookies'); console.log('Total cookies :'+phantom.cookies.length); // will output the total cookies added to the url. } else { console.error('Cannot open file'); phantom.exit(1); } });
例子
a.html
<html> <head> <title>Welcome to phantomjs test page</title> </head> <body> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> </body> </html>
上述程序生成以下输出。
Added 3 cookies Total cookies :3
代码注释是不言自明的。
清除Cookies
此方法允许删除所有 cookie。
句法
其语法如下 -
phantom.clearCookies();
此概念的工作原理类似于通过在浏览器菜单中进行选择来删除浏览器 cookie。
例子
这是clearCookies方法的示例。
var page = require('webpage').create(),url = 'http://localhost/tasks/a.html'; page.open(url, function(status) { if (status === 'success') { phantom.addCookie({ //add name cookie1 with value = 1 name: 'cookie1', value: '1', domain: 'localhost' }); phantom.addCookie({ // add cookie2 with value 2 name: 'cookie2', value: '2', domain: 'localhost' }); phantom.addCookie({ // add cookie3 with value 3 name: 'cookie3', value: '3', domain: 'localhost' }); console.log('Added 3 cookies'); console.log('Total cookies :'+phantom.cookies.length); phantom.clearCookies(); console.log( 'After clearcookies method total cookies :' +phantom.cookies.length); phantom.exit(); } else { console.error('Cannot open file'); phantom.exit(1); } });
a.html
<html> <head> <title>Welcome to phantomjs test page</title> </head> <body> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> <h1>This is a test page</h1> </body> </html>
上述程序生成以下输出。
Added 3 cookies Total cookies :3 After clearcookies method total cookies :0
删除Cookie
删除CookieJar中“name”属性与 cookieName 匹配的所有 cookie 。如果删除成功,则返回true ;否则为假。
句法
其语法如下 -
phantom.deleteCookie(cookiename);
让我们通过一个例子来了解addcookie、clearcookies和deletecookie 。
例子
这是一个演示 deleteCookie 方法使用的示例 -
文件:cookie.js
var page = require('webpage').create(),url = 'http://localhost/tasks/a.html'; page.open(url, function(status) { if (status === 'success') { phantom.addCookie({ //add name cookie1 with value = 1 name: 'cookie1', value: '1', domain: 'localhost' }); phantom.addCookie({ // add cookie2 with value 2 name: 'cookie2', value: '2', domain: 'localhost' }); phantom.addCookie({ // add cookie3 with value 3 name: 'cookie3', value: '3', domain: 'localhost' }); console.log('Added 3 cookies'); console.log('Total cookies :'+phantom.cookies.length); //will output the total cookies added to the url. console.log("Deleting cookie2"); phantom.deleteCookie('cookie2'); console.log('Total cookies :'+phantom.cookies.length); phantom.clearCookies(); console.log( 'After clearcookies method total cookies :' +phantom.cookies.length); phantom.exit(); } else { console.error('Cannot open file'); phantom.exit(1); } });
上述程序生成以下输出。
phantomjs cookie.js Added 3 cookies Total cookies :3 Deleting cookie2 Total cookies :2 After clearcookies method total cookies :0
出口
phantom.exit 方法将退出它已启动的脚本。它退出程序并带有提到的返回值。如果没有传递任何值,它会给出'0' 。
句法
其语法如下 -
phantom.exit(value);
如果您不添加phantom.exit,则命令行假定执行仍在进行并且不会完成。
例子
让我们看一个例子来了解exit方法的使用。
console.log('Welcome to phantomJs'); // outputs Welcome to phantomJS var a = 1; if (a === 1) { console.log('Exit 1'); //outputs Exit 1 phantom.exit(); // Code exits. } else { console.log('Exit 2'); phantom.exit(1); }
上述程序生成以下输出。
phantomjs exit.js
Welcome to phantomJs Exit 1
phantom.exit 之后的任何代码都不会被执行,因为 phantom.exit 是结束脚本的方法。
注入
InjectJs用于在phantom中添加addtionaljs文件。如果在当前目录 librarypath中找不到该文件,则将使用 phantom 属性 (phantom.libraryPath) 作为跟踪路径的附加位置。如果文件添加成功则返回true ,否则返回false,如果无法找到文件则失败。
句法
其语法如下 -
phantom.injectJs(filename);
例子
让我们看下面的例子来了解injectJs的使用。
文件名:inject.js
console.log(“Added file”);
文件名:addfile.js
var addfile = injectJs(inject.js); console.log(addfile); phantom.exit();
输出
命令- C:\phantomjs\bin>phantomjs addfile.js
Added file // coming from inject.js true
在上面的示例中,addfile.js使用injectJs调用文件inject.js。当您执行 addfile.js 时,inject.js 中存在的 console.log 将显示在输出中。由于文件 insert.js 已成功添加,因此 addfile 变量也显示为 true。