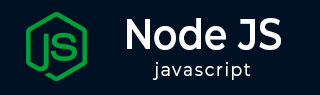
- Node.js 教程
- Node.js - 主页
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 第一个应用程序
- Node.js - REPL 终端
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 缓冲区
- Node.js - 流
- Node.js - 文件系统
- Node.js - 全局对象
- Node.js - 实用模块
- Node.js - Web 模块
- Node.js - Express 框架
- Node.js - RESTFul API
- Node.js - 扩展应用程序
- Node.js - 打包
- Node.js - 内置模块
- Node.js 有用资源
- Node.js - 快速指南
- Node.js - 有用的资源
- Node.js - 讨论
Node.js - Console
Node.js console is a global object and is used to print different levels of messages to stdout and stderr. There are built-in methods to be used for printing informational, warning, and error messages.
It is used in synchronous way when the destination is a file or a terminal and in asynchronous way when the destination is a pipe.
Console Methods
Following is a list of methods available with the console global object.
Sr.No. | Method & Description |
---|---|
1 |
console.log([data][, ...]) Prints to stdout with newline. This function can take multiple arguments in a printf()-like way. |
2 |
console.info([data][, ...]) Prints to stdout with newline. This function can take multiple arguments in a printf()-like way. |
3 |
console.error([data][, ...]) Prints to stderr with newline. This function can take multiple arguments in a printf()-like way. |
4 |
console.warn([data][, ...]) Prints to stderr with newline. This function can take multiple arguments in a printf()-like way |
5 |
console.dir(obj[, options]) Uses util.inspect on obj and prints resulting string to stdout. |
6 |
console.time(label) Mark a time. |
7 |
console.timeEnd(label) Finish timer, record output. |
8 |
console.trace(message[, ...]) Print to stderr 'Trace :', followed by the formatted message and stack trace to the current position. |
9 |
console.assert(value[, message][, ...]) Similar to assert.ok(), but the error message is formatted as util.format(message...). |
Example
Let us create a js file named main.js with the following code −
console.info("Program Started"); var counter = 10; console.log("Counter: %d", counter); console.time("Getting data"); // // Do some processing here... // console.timeEnd('Getting data'); console.info("Program Ended")
Now run the main.js to see the result −
node main.js
Verify the Output.
Program Started Counter: 10 Getting data: 0ms Program Ended