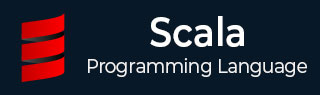
- Scala Tutorial
- Scala - Home
- Scala - Overview
- Scala - Environment Setup
- Scala - Basic Syntax
- Scala - Data Types
- Scala - Variables
- Scala - Classes & Objects
- Scala - Access Modifiers
- Scala - Operators
- Scala - IF ELSE
- Scala - Loop Statements
- Scala - Functions
- Scala - Closures
- Scala - Strings
- Scala - Arrays
- Scala - Collections
- Scala - Traits
- Scala - Pattern Matching
- Scala - Regular Expressions
- Scala - Exception Handling
- Scala - Extractors
- Scala - Files I/O
- Scala Useful Resources
- Scala - Quick Guide
- Scala - Useful Resources
- Scala - Discussion
Scala -break 语句
因此,Scala 中没有可用的内置 Break 语句,但如果您运行的是 Scala 2.8 版本,则可以使用Break语句。当循环内遇到break语句时,循环立即终止,程序控制从循环后面的下一条语句恢复。
流程图

句法
以下是break语句的语法。
// import following package import scala.util.control._ // create a Breaks object as follows val loop = new Breaks; // Keep the loop inside breakable as follows loop.breakable { // Loop will go here for(...){ .... // Break will go here loop.break; } }
尝试以下示例程序来理解break语句。
例子
import scala.util.control._ object Demo { def main(args: Array[String]) { var a = 0; val numList = List(1,2,3,4,5,6,7,8,9,10); val loop = new Breaks; loop.breakable { for( a <- numList){ println( "Value of a: " + a ); if( a == 4 ){ loop.break; } } } println( "After the loop" ); } }
将上述程序保存在Demo.scala中。以下命令用于编译和执行该程序。
命令
\>scalac Demo.scala \>scala Demo
输出
Value of a: 1 Value of a: 2 Value of a: 3 Value of a: 4 After the loop
打破嵌套循环
现有的break在使用嵌套循环时存在问题。为了防止嵌套循环使用break,请遵循此方法。这是一个用于打破嵌套循环的示例程序。
例子
import scala.util.control._ object Demo { def main(args: Array[String]) { var a = 0; var b = 0; val numList1 = List(1,2,3,4,5); val numList2 = List(11,12,13); val outer = new Breaks; val inner = new Breaks; outer.breakable { for( a <- numList1){ println( "Value of a: " + a ); inner.breakable { for( b <- numList2){ println( "Value of b: " + b ); if( b == 12 ){ inner.break; } } } // inner breakable } } // outer breakable. } }
将上述程序保存在Demo.scala中。以下命令用于编译和执行该程序。
命令
\>scalac Demo.scala \>scala Demo
输出
Value of a: 1 Value of b: 11 Value of b: 12 Value of a: 2 Value of b: 11 Value of b: 12 Value of a: 3 Value of b: 11 Value of b: 12 Value of a: 4 Value of b: 11 Value of b: 12 Value of a: 5 Value of b: 11 Value of b: 12
scala_loop_types.htm