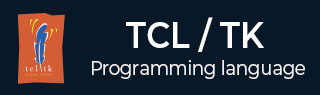
- Tcl教程
- Tcl - 首页
- Tcl - 概述
- Tcl - 环境设置
- Tcl - 特殊变量
- Tcl - 基本语法
- Tcl - 命令
- Tcl - 数据类型
- Tcl - 变量
- Tcl - 运营商
- Tcl - 决策
- Tcl - 循环
- Tcl - 数组
- Tcl - 字符串
- Tcl - 列表
- Tcl - 字典
- Tcl - 程序
- Tcl - 套餐
- Tcl - 命名空间
- Tcl - 文件 I/O
- Tcl - 错误处理
- Tcl - 内置函数
- Tcl - 正则表达式
- TK 教程
- Tk - 概述
- Tk-环境
- Tk - 特殊变量
- Tk - 小部件概述
- Tk - 基本小部件
- Tk - 布局小部件
- Tk - 选择小部件
- Tk - 画布小部件
- Tk - 大型小部件
- Tk-字体
- Tk - 图像
- Tk - 活动
- Tk-Windows 管理器
- Tk - 几何管理器
- Tcl/Tk 有用资源
- Tcl/Tk - 快速指南
- Tcl/Tk - 有用的资源
- Tcl/Tk - 讨论
Tcl - If else 语句
if语句后面可以跟一个可选的else语句,该语句在布尔表达式为 false 时执行。
句法
Tcl 语言中“ if...else ”语句的语法为 -
if {boolean_expression} { # statement(s) will execute if the boolean expression is true } else { # statement(s) will execute if the boolean expression is false }
如果布尔表达式的计算结果为true,则将执行if 代码块,否则将执行else 代码块。
Tcl语言内部使用expr命令,因此我们不需要显式地使用expr语句。
流程图

例子
#!/usr/bin/tclsh set a 100 #check the boolean condition if {$a < 20 } { #if condition is true then print the following puts "a is less than 20" } else { #if condition is false then print the following puts "a is not less than 20" } puts "value of a is : $a"
当上面的代码被编译并执行时,它会产生以下结果 -
a is not less than 20; value of a is : 100
if...else if...else 语句
“ if ”语句后面可以跟一个可选的else if...else语句,这对于使用单个 if...else if 语句测试各种条件非常有用。
当使用 if 、 else if 、 else 语句时,有几点需要记住 -
' if ' 可以有零个或一个else,并且它必须位于任何else if 之后。
一个“ if ”可以有零到多个else if,并且它们必须位于else 之前。
一旦“ else if ”成功,则不会测试其余的else if或else 。
句法
Tcl 语言中“ if...else if...else ”语句的语法为 -
if {boolean_expression 1} { # Executes when the boolean expression 1 is true } elseif {boolean_expression 2} { # Executes when the boolean expression 2 is true } elseif {boolean_expression 3} { # Executes when the boolean expression 3 is true } else { # executes when the none of the above condition is true }
例子
#!/usr/bin/tclsh set a 100 #check the boolean condition if { $a == 10 } { # if condition is true then print the following puts "Value of a is 10" } elseif { $a == 20 } { # if else if condition is true puts "Value of a is 20" } elseif { $a == 30 } { # if else if condition is true puts "Value of a is 30" } else { # if none of the conditions is true puts "None of the values is matching" } puts "Exact value of a is: $a"
当上面的代码被编译并执行时,它会产生以下结果 -
None of the values is matching Exact value of a is: 100
tcl_decisions.htm