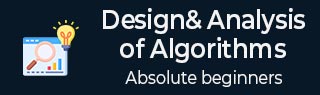
- 算法设计与分析
- 家
- 算法基础知识
- DAA - 简介
- DAA - 算法分析
- DAA-分析方法
- 渐近符号和先验分析
- 时间复杂度
- 马斯特定理
- DAA - 空间复杂性
- 分而治之
- DAA-分而治之
- DAA - 最大最小问题
- DAA-归并排序
- DAA-二分查找
- 施特拉森矩阵乘法
- 唐叶算法
- 河内塔
- 贪心算法
- DAA-贪婪法
- 旅行商问题
- Prim 的最小生成树
- 克鲁斯卡尔的最小生成树
- Dijkstra 的最短路径算法
- 地图着色算法
- DAA-分数背包
- DAA - 带截止日期的作业排序
- DAA - 最佳合并模式
- 动态规划
- DAA-动态规划
- 矩阵链乘法
- 弗洛伊德·沃歇尔算法
- DAA - 0-1 背包
- 最长公共子序列
- 旅行商问题| 动态规划
- 随机算法
- 随机算法
- 随机快速排序
- 卡格的最低削减
- 费舍尔-耶茨洗牌
- 近似算法
- 近似算法
- 顶点覆盖问题
- 设置封面问题
- 旅行推销员近似算法
- 排序技巧
- DAA-快速排序
- DAA-冒泡排序
- DAA——插入排序
- DAA-选择排序
- DAA——希尔排序
- DAA-堆排序
- DAA——桶排序
- DAA——计数排序
- DAA - 基数排序
- 搜索技巧
- 搜索技术介绍
- DAA - 线性搜索
- DAA-二分查找
- DAA - 插值搜索
- DAA - 跳转搜索
- DAA - 指数搜索
- DAA - 斐波那契搜索
- DAA - 子列表搜索
- DAA-哈希表
- 图论
- DAA-最短路径
- DAA - 多级图
- 最优成本二叉搜索树
- 堆算法
- DAA-二叉堆
- DAA-插入法
- DAA-Heapify 方法
- DAA-提取方法
- 复杂性理论
- 确定性计算与非确定性计算
- DAA-最大派系
- DAA - 顶点覆盖
- DAA - P 级和 NP 级
- DAA-库克定理
- NP 硬课程和 NP 完全课程
- DAA - 爬山算法
- DAA 有用资源
- DAA - 快速指南
- DAA - 有用的资源
- DAA - 讨论
设计与分析插入法
要在堆中插入元素,新元素最初会作为数组的最后一个元素附加到堆的末尾。
插入该元素后,可能会违反堆属性,因此通过将添加的元素与其父元素进行比较并将添加的元素向上移动一个级别,与父元素交换位置来修复堆属性。这个过程称为向上渗透。
重复比较,直到父元素大于或等于渗透元素。
Algorithm: Max-Heap-Insert (numbers[], key) heapsize = heapsize + 1 numbers[heapsize] = -∞ i = heapsize numbers[i] = key while i > 1 and numbers[Parent(numbers[], i)] < numbers[i] exchange(numbers[i], numbers[Parent(numbers[], i)]) i = Parent (numbers[], i)
分析
最初,一个元素被添加到数组的末尾。如果它违反了堆属性,则该元素将与其父元素交换。树的高度是log n。需要执行的最大log n次操作。
因此,该函数的复杂度为O(log n)。
例子
让我们考虑一个最大堆,如下所示,其中需要添加新元素 5。

最初,55 将添加到该数组的末尾。

插入后,它违反了堆属性。因此,该元素需要与其父元素交换。交换后,堆如下所示。

同样,该元素违反了堆的属性。因此,它与其父级交换。

现在,我们必须停下来。
例子
#include <stdio.h> void swap(int* a, int* b) { int temp = *a; *a = *b; *b = temp; } int parent(int i) { if (i == 0) return -1; else return (i - 1) / 2; } void maxHeapInsert(int arr[], int* heapSize, int key) { (*heapSize)++; int i = *heapSize; arr[i] = key; while (i > 1 && arr[parent(i)] < arr[i]) { swap(&arr[i], &arr[parent(i)]); i = parent(i); } } int main() { int arr[100] = { 50, 30, 40, 20, 15, 10 }; // Initial Max-Heap int heapSize = 5; // Current heap size // New element to be inserted int newElement = 5; // Insert the new element into the Max-Heap maxHeapInsert(arr, &heapSize, newElement); // Print the updated Max-Heap printf("Updated Max-Heap: "); for (int i = 0; i <= heapSize; i++) printf("%d ", arr[i]); printf("\n"); return 0; }
输出
Updated Max-Heap: 50 30 40 20 15 10 5
#include <iostream> #include <vector> using namespace std; void swap(int& a, int& b) { int temp = a; a = b; b = temp; } int parent(int i) { if (i == 0) return -1; else return (i - 1) / 2; } void maxHeapInsert(vector<int>& arr, int& heapSize, int key) { heapSize++; int i = heapSize; // Resize the vector to accommodate the new element arr.push_back(0); arr[i] = key; while (i > 1 && arr[parent(i)] < arr[i]) { swap(arr[i], arr[parent(i)]); i = parent(i); } } int main() { vector<int> arr = { 50, 30, 40, 20, 15, 10 }; // Initial Max-Heap int heapSize = 5; // Current heap size // New element to be inserted int newElement = 5; // Insert the new element into the Max-Heap maxHeapInsert(arr, heapSize, newElement); // Print the updated Max-Heap cout << "Updated Max-Heap: "; for (int i = 0; i <= heapSize; i++) cout << arr[i] << " "; cout << endl; return 0; }
输出
Updated Max-Heap: 50 30 40 20 15 10 5
import java.util.Arrays; public class MaxHeap { public static void swap(int arr[], int i, int j) { int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } public static int parent(int i) { if (i == 0) return -1; else return (i - 1) / 2; } public static void maxHeapInsert(int arr[], int heapSize, int key) { heapSize++; int i = heapSize - 1; // Adjust the index for array insertion arr[i] = key; while (i > 0 && arr[parent(i)] < arr[i]) { swap(arr, i, parent(i)); i = parent(i); } } public static void main(String args[]) { int arr[] = { 50, 30, 40, 20, 15, 10 }; // Initial Max-Heap int heapSize = 5; // Current heap size // New element to be inserted int newElement = 5; // Insert the new element into the Max-Heap maxHeapInsert(arr, heapSize, newElement); // Print the updated Max-Heap System.out.print("Updated Max-Heap: "); for (int i = 0; i <= heapSize; i++) System.out.print(arr[i] + " "); System.out.println(); } }
输出
Updated Max-Heap: 50 30 40 20 15 5
def swap(arr, i, j): arr[i], arr[j] = arr[j], arr[i] def parent(i): if i == 0: return -1 else: return (i - 1) // 2 def max_heap_insert(arr, heap_size, key): heap_size += 1 i = heap_size arr.append(key) while i > 0 and arr[parent(i)] < arr[i]: swap(arr, i, parent(i)) i = parent(i) if __name__ == "__main__": arr = [50, 30, 40, 20, 15, 10] # Initial Max-Heap heap_size = 5 # Current heap size # New element to be inserted new_element = 5 # Insert the new element into the Max-Heap max_heap_insert(arr, heap_size, new_element) # Print the updated Max-Heap print("Updated Max-Heap:", arr)
输出
Updated Max-Heap: [50, 30, 40, 20, 15, 10, 5]