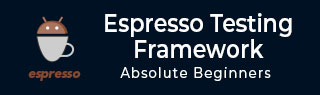
- Espresso 测试框架教程
- Espresso测试 - 主页
- 介绍
- 设置说明
- 在 Android Studio 中运行测试
- JUnit 概述
- 建筑学
- 查看匹配器
- 自定义视图匹配器
- 查看断言
- 查看操作
- 测试AdapterView
- 测试网页视图
- 测试异步操作
- 测试意图
- 测试多个应用程序的 UI
- 测试记录仪
- 测试用户界面性能
- 测试可访问性
- Espresso测试资源
- Espresso测试 - 快速指南
- Espresso 测试 - 有用资源
- Espresso测试 - 讨论
Espresso 测试框架 - WebView
WebView是android提供的一种特殊视图,用于在应用程序内显示网页。WebView不提供像 chrome 和 firefox 这样成熟的浏览器应用程序的所有功能。但是,它提供了对要显示的内容的完全控制,并公开了要在网页内调用的所有 Android 功能。它支持WebView并提供一个特殊的环境,可以使用 HTML 技术和本机功能(如相机和拨号联系人)轻松设计 UI。此功能集使WebView能够提供一种称为混合应用程序的新型应用程序,其中 UI 用 HTML 完成,业务逻辑用JavaScript或通过外部 API 端点完成。
通常,测试WebView是一项挑战,因为它的用户界面元素使用 HTML 技术,而不是本机用户界面/视图。Espresso 在这一领域表现出色,为 Web 匹配器和 Web 断言提供了一组新的设置,有意与本机视图匹配器和视图断言类似。同时,它还包含基于 Web 技术的测试环境,提供了一种均衡的方法。
Espresso Web 基于WebDriver Atom 框架构建,用于查找和操作 Web 元素。Atom与视图操作类似。Atom 将完成网页内的所有交互。WebDriver公开了一组预定义的方法,例如findElement()、getElement()来查找 Web 元素并返回相应的Atomics(以在网页中执行操作)。
标准的 Web 测试语句类似于以下代码,
onWebView() .withElement(Atom) .perform(Atom) .check(WebAssertion)
这里,
onWebView() - 与 onView() 类似,它公开了一组 API 来测试 WebView。
withElement() - 使用 Atom 在网页内定位 Web 元素的几种方法之一,并返回 WebInteration 对象,该对象类似于 ViewInteraction。
Perform() - 使用 Atom 在网页内执行操作并返回 WebInteraction。
check() - 这使用 WebAssertion 进行必要的断言。
示例 Web 测试代码如下:
onWebView() .withElement(findElement(Locator.ID, "apple")) .check(webMatches(getText(), containsString("Apple")))
这里,
findElement()定位一个元素并返回一个 Atom
webMatches与 matches 方法类似
编写示例应用程序
让我们编写一个基于WebView的简单应用程序,并使用onWebView()方法编写一个测试用例。按照以下步骤编写示例应用程序 -
启动 Android 工作室。
如前所述创建新项目并将其命名为MyWebViewApp。
使用Refactor → Migrate to AndroidX选项菜单将应用程序迁移到 AndroidX 框架。
在AndroidManifest.xml文件中添加以下配置选项以授予访问 Internet 的权限。
<uses-permission android:name = "android.permission.INTERNET" />
Espresso web 作为单独的插件提供。因此,在 app/build.gradle 中添加依赖项并同步它。
dependencies { androidTestImplementation 'androidx.test:rules:1.1.1' androidTestImplementation 'androidx.test.espresso:espresso-web:3.1.1' }
删除主 Activity 中的默认设计并添加 WebView。Activity_main.xml的内容如下,
<?xml version = "1.0" encoding = "utf-8"?> <RelativeLayout xmlns:android = "http://schemas.android.com/apk/res/android" xmlns:app = "http://schemas.android.com/apk/res-auto" xmlns:tools = "http://schemas.android.com/tools" android:layout_width = "match_parent" android:layout_height = "match_parent" tools:context = ".MainActivity"> <WebView android:id = "@+id/web_view_test" android:layout_width = "fill_parent" android:layout_height = "fill_parent" /> </RelativeLayout>
创建一个新类ExtendedWebViewClient扩展WebViewClient并重写shouldOverrideUrlLoading方法以在同一WebView中加载链接操作;否则,它将在应用程序外部打开一个新的浏览器窗口。将其放在MainActivity.java中。
private class ExtendedWebViewClient extends WebViewClient { @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { view.loadUrl(url); return true; } }
现在,在MainActivity的 onCreate 方法中添加以下代码。代码的目的是找到WebView,正确配置它,然后最终加载目标 url。
// Find web view WebView webView = (WebView) findViewById(R.id.web_view_test); // set web view client webView.setWebViewClient(new ExtendedWebViewClient()); // Clear cache webView.clearCache(true); // load Url webView.loadUrl("http://<your domain or IP>/index.html");
这里,
index.html的内容如下 -
<html> <head> <title>Android Web View Sample</title> </head> <body> <h1>Fruits</h1> <ol> <li><a href = "apple.html" id = "apple">Apple</a></li> <li><a href = "banana.html" id = "banana">Banana</a></li> </ol> </body> </html>
index.html中引用的apple.html文件的内容如下 -
<html> <head> <title>Android Web View Sample</title> </head> <body> <h1>Apple</h1> </body> </html>
banana.html中引用的banana.html文件内容如下,
<html> <head> <title>Android Web View Sample</title> </head> <body> <h1>Banana</h1> </body> </html>
将index.html、apple.html 和banana.html 放置在Web 服务器中
将 loadUrl 方法中的 url 替换为您配置的 url。
现在,运行应用程序并手动检查一切是否正常。下面是WebView 示例应用程序的屏幕截图-

现在,打开ExampleInstrumentedTest.java文件并添加以下规则 -
@Rule public ActivityTestRule<MainActivity> mActivityRule = new ActivityTestRule<MainActivity>(MainActivity.class, false, true) { @Override protected void afterActivityLaunched() { onWebView(withId(R.id.web_view_test)).forceJavascriptEnabled(); } };
在这里,我们找到了WebView并启用了WebView的 JavaScript ,因为 espresso Web 测试框架专门通过 JavaScript 引擎来识别和操作 Web 元素。
现在,添加测试用例来测试我们的WebView及其Behave。
@Test public void webViewTest(){ onWebView() .withElement(findElement(Locator.ID, "apple")) .check(webMatches(getText(), containsString("Apple"))) .perform(webClick()) .withElement(findElement(Locator.TAG_NAME, "h1")) .check(webMatches(getText(), containsString("Apple"))); }
在这里,测试按以下顺序完成,
找到链接,苹果通过findElement()方法和Locator.ID枚举使用其 id 属性。
使用webMatches()方法检查链接的文本
对链接执行单击操作。它会打开apple.html页面。
再次使用 findElement() 方法和Locator.TAG_NAME枚举找到 h1 元素。
最后再次使用webMatches()方法检查h1标签的文本。
最后,使用 android studio 上下文菜单运行测试用例。