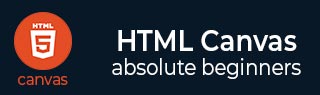
- HTML 画布教程
- HTML 画布 - 主页
- HTML 画布 - 简介
- 环境设置
- HTML Canvas - 第一个应用程序
- HTML Canvas - 绘制 2D 形状
- HTML Canvas - 路径元素
- 使用路径元素的 2D 形状
- HTML 画布 - 颜色
- HTML Canvas - 添加样式
- HTML Canvas - 添加文本
- HTML Canvas - 添加图像
- HTML Canvas - 画布时钟
- HTML Canvas - 转换
- 堆肥和修剪
- HTML Canvas - 基本动画
- 高级动画
- HTML Canvas 有用的资源
- HTML Canvas - 快速指南
- HTML Canvas - 有用的资源
- HTML Canvas - 讨论
HTML Canvas - 高级动画
在上一章中,基本动画帮助我们了解如何为 Canvas 元素设置动画。在这里,我们将了解动画的物理概念,例如速度、加速度等。
让我们研究一个简单的加速示例,其中我们使用一个小方块来展开和折叠。下面给出实现代码。
<!DOCTYPE html> <html lang="en"> <head> <title>Advanced Animations</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="animation();"> <canvas id="canvas" width="555" height="555" style="border: 1px solid black;"></canvas> <script> function animation() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); var reqframe; var square = { posx: 0, posy: 0, width: 50, height: 50, vx: 2, vy: 1, draw: function() { context.fillRect(this.posx, this.posy, this.width, this.height); context.fillStyle = 'red'; context.fill(); } }; function draw() { context.clearRect(0, 0, canvas.width, canvas.height); square.draw(); square.width += square.vx; square.height += square.vy; if (square.height + square.vy > canvas.height || square.height + square.vy < 0) { square.vy = -square.vy; } if (square.width + square.vx > canvas.width || square.width + square.vx < 0) { square.vx = -square.vx; } reqframe = window.requestAnimationFrame(draw); } canvas.addEventListener('mouseover', function(e) { reqframe = window.requestAnimationFrame(draw); }); canvas.addEventListener('mouseout', function(e) { window.cancelAnimationFrame(reqframe); }); } </script> </body> </html>
输出
代码返回的输出是

