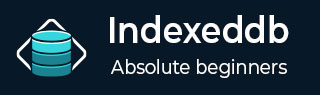
- IndexedDB Tutorial
- IndexedDB - Home
- IndexedDB - Introduction
- IndexedDB - Installation
- IndexedDB - Connection
- IndexedDB - Object Stores
- IndexedDB - Creating Data
- IndexedDB - Reading Data
- IndexedDB - Updating Data
- IndexedDB - Deleting Data
- Using getAll() Functions
- IndexedDB - Indexes
- IndexedDB - Ranges
- IndexedDB - Transactions
- IndexedDB - Error Handling
- IndexedDB - Searching
- IndexedDB - Cursors
- IndexedDB - Promise Wrapper
- IndexedDB - Ecmascript Binding
- IndexedDB Useful Resources
- IndexedDB - Quick Guide
- IndexedDB - Useful Resources
- IndexedDB - Discussion
IndexedDB - 游标
在检索数据时,当我们知道要检索哪个键时,我们使用get()函数,但如果我们想逐步遍历对象存储的所有值,我们可以使用游标。
首先我们使用打开游标函数,然后我们可以向其中添加我们的参数。我们可以在openCursor()函数中插入的参数是 -
- 使用键范围限制对象范围
- 我们想要迭代的方向
以下是游标的语法
句法
ObjectStore.openCursor(optionalKeyRange, optionalDirection);
对于对象存储,我们使用 openCursor ()
optionalKeyRange - 我们可以限制需要检索的对象数量的范围。
optionDirection - 我们可以指定我们想要迭代的方向。
实施例1
在此示例中,我们学习如何使用 JavaScript 打开光标函数 -
var objectStore = db.transaction("student").objectStore("student”); objectStore.openCursor().onsuccess = event => { var cursor = event.target.result; if (cursor) { document.write("Name" + cursor.key + cursor.value.name); cursor.continue(); } else { document.write("entries closed"); } };
实施例2
当我们想要从对象存储中检索所有对象并将它们放入数组中时。
var student = []; objectStore.openCursor().onsuccess = event => { var cursor = event.target.result; if (cursor) { student.push(cursor.value); cursor.continue(); } else { document.write(student); } };
实施例3
下面给出了在 JavaScript 中实现 openCursor() 函数的另一个示例 -
var singleKeyRange = IDBKeyRange.only("Jason"); var lowerBoundKeyRange = IDBKeyRange.lowerBound("Praneeth"); var lowerBoundOpenKeyRange = IDBKeyRange.lowerBound("jason", true); var upperBoundOpenKeyRange = IDBKeyRange.upperBound("praneeth", true); var boundKeyRange = IDBKeyRange.bound("jason", "praneeth", false, true); index.openCursor(boundKeyRange).onsuccess = event => { var cursor = event.target.result; if (cursor) { cursor.continue(); } };
或者如果我们想给出方向 -
objectStore.openCursor(boundKeyRange, "prev").onsuccess = event => { var cursor = event.target.result; if (cursor) { cursor.continue(); } };
HTML 示例
实现光标功能使用的 HTML 脚本如下:
<!DOCTYPE html> <html lang="en"> <head> <title>Document</title> </head> <body> <script> const request = indexedDB.open("botdatabase",1); request.onupgradeneeded = function(){ const db = request.result; const store = db.createObjectStore("bots",{ keyPath: "id"}); store.createIndex("branch_db",["branch"],{unique: false}); } request.onsuccess = function(){ document.write("database opened successfully"); const db = request.result; const transaction=db.transaction("bots","readwrite"); const store = transaction.objectStore("bots"); const branchIndex = store.index("branch_db"); store.add({id: 1, name: "jason",branch: "IT"}); store.add({id: 2, name: "praneeth",branch: "CSE"}); store.add({id: 3, name: "palli",branch: "EEE"}); store.add({id: 4, name: "abdul",branch: "IT"}); store.put({id: 4, name: "deevana",branch: "CSE"}); const req = store.openCursor(); req.onsuccess = function(){ const cursor = req.result; if(cursor){ const key = cursor.key; const value = cursor.value; document.write(key,value); cursor.continue(); } else { document.write("bots completed"); } } transaction.oncomplete = function(){ db.close; } } </script> </body> </html>
输出
database opened successfully 1 {id: 1, name: 'jason', branch: 'IT'} 2 {id: 2, name: 'praneeth', branch: 'CSE'} 3 {id: 3, name: 'palli', branch: 'EEE'} 4 {id: 4, name: 'deevana', branch: 'CSE'} bots completed