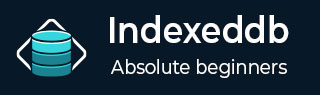
- IndexedDB Tutorial
- IndexedDB - Home
- IndexedDB - Introduction
- IndexedDB - Installation
- IndexedDB - Connection
- IndexedDB - Object Stores
- IndexedDB - Creating Data
- IndexedDB - Reading Data
- IndexedDB - Updating Data
- IndexedDB - Deleting Data
- Using getAll() Functions
- IndexedDB - Indexes
- IndexedDB - Ranges
- IndexedDB - Transactions
- IndexedDB - Error Handling
- IndexedDB - Searching
- IndexedDB - Cursors
- IndexedDB - Promise Wrapper
- IndexedDB - Ecmascript Binding
- IndexedDB Useful Resources
- IndexedDB - Quick Guide
- IndexedDB - Useful Resources
- IndexedDB - Discussion
IndexedDB - 搜索
我们遇到很多需要在对象存储中搜索值的情况。对象存储在内部排序。可以通过以下方式完成 -
- 按键值或键范围搜索。
- 基于另一个对象字段进行搜索。
按键搜索
我们可以通过使用具有可接受的键范围的 IDBKeyRange 对象来搜索精确的键值或一系列键值。IDBKeyRange 对象有以下调用 -
IDBKeyRange.lowerBound(lower, [open]) for >=lower
IDBKeyRange.upperBound(upper, [open]) for >=upper
IDBKeyRange.bound(lower, upper, [lowerOpen] , [upperOpen]) 介于上下之间
IDBKeyRange.only(key) 如果范围仅包含一个键。
为了执行实际的搜索,我们使用对象存储上的查询参数。执行这些操作的不同类型的方法是
store.get(query) - 通过键或范围搜索存储中的第一个值
store.getAll([query],[count]) - 搜索存储中的所有值,直到达到提到的计数限制。
store.getKey(query) - 搜索满足查询的第一个键。
store.getAllKeys([query],[count]) - 搜索满足查询的所有键,直到完成计数限制。
store.count([query]) - 获取满足查询的键的总数。
例子
在此示例中,我们使用 getAll() 方法检索所有对象并按对象的键搜索对象 -
class.get(‘student’) class.getAll(IDBKeyRange.bound(‘science’,’math’) class.getAll(IDBKeyRange.upperbound(‘science’,true) class.getAll() class.getAllKeys(IDBKeyRange.lowerbound(‘student’,true))
按字段或索引搜索
要基于其他对象字段进行搜索,我们需要使用索引。索引存储具有所需值的对象的键列表。索引也像对象存储一样在内部排序。
句法
objectStore.createIndex(name, keyPath, [options]);
名称- 索引名称
keyPath - 搜索将在对象字段的路径上完成
选项- 选项有两种类型
unique - 存储中具有唯一值的对象将出现在关键路径上,并且不能对其进行重复。
多条目- 如果键路径上的值是一个数组,那么默认情况下索引会将整个数组视为键,但如果我们使用多条目,则数组成员将成为索引键。
例子
如果我们想根据价格搜索手机,示例程序如下 -
openRequest.onupgradeneeded = function() { let books = db.createObjectStore('phone', {keyPath: 'id'}); let index = books.createIndex('pricephone', 'price'); };
要创建索引,我们需要使用所需的升级。
- 该指数将跟踪价格字段。
- 如果价格不唯一,我们就无法设置唯一选项。
- 如果价格不是数组,则 multiEntry 不适用。
例子
在下面的示例中,我们创建一个事务并使用 getAll() 函数检索所有对象。一旦检索到它们,我们就在该事务中搜索对象值。如果找到,则返回该对象;如果不是,则返回 false。
let transaction = db.transaction("phones"); let books = transaction.objectStore("phones"); let priceIndex = books.index("price_index"); let request = priceIndex.getAll(7); request.onsuccess = function() { if (request.result !== undefined) { document.write("Phones", request.result); } else { document.write("There are no such phones"); } };
HTML 示例
下面给出了在对象存储中搜索值的 HTML 脚本实现 -
<!DOCTYPE html> <html lang="en"> <head> <title>Document</title> </head> <body> <script> const request = indexedDB.open("botdatabase",1); request.onupgradeneeded = function(){ const db = request.result; const store = db.createObjectStore("bots",{ keyPath: "id"}); store.createIndex("branch_db",["branch"],{unique: false}); } request.onsuccess = function(){ document.write("database opened successfully"); const db = request.result; const transaction=db.transaction("bots","readwrite"); const store = transaction.objectStore("bots"); const branchIndex = store.index("branch_db"); store.add({id: 1, name: "jason",branch: "IT"}); store.add({id: 2, name: "praneeth",branch: "CSE"}); store.add({id: 3, name: "palli",branch: "EEE"}); store.add({id: 4, name: "abdul",branch: "IT"}); store.put({id: 4, name: "deevana",branch: "CSE"}); const req = branchIndex.getAll(["CSE"]); req.onsuccess = function(){ if(req.result!==undefined){ document.write("bots",req.result); } else{ document.write("There are no such bots"); } }; transaction.oncomplete = function(){ db.close; } } </script> </body> </html>
输出
database opened successfully bots (2) [{...}, {...}] arg1:(2) [{...}, {...}] 0:{id: 2, name: 'praneeth', branch: 'CSE'} 1:{id: 4, name: 'deevana', branch: 'CSE'} length:2 [[Prototype]]:Array(0) [[Prototype]]:Object