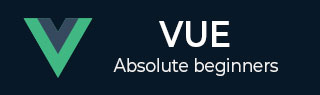
- VueJS Tutorial
- VueJS - Home
- VueJS - Overview
- VueJS - Environment Setup
- VueJS - Introduction
- VueJS - Instances
- VueJS - Template
- VueJS - Components
- VueJS - Computed Properties
- VueJS - Watch Property
- VueJS - Binding
- VueJS - Events
- VueJS - Rendering
- VueJS - Transition & Animation
- VueJS - Directives
- VueJS - Routing
- VueJS - Mixins
- VueJS - Render Function
- VueJS - Reactive Interface
- VueJS - Examples
- VueJS Useful Resources
- VueJS - Quick Guide
- VueJS - Useful Resources
- VueJS - Discussion
VueJS - 混合
Mixin 基本上是与组件一起使用的。它们在组件之间共享可重用的代码。当组件使用mixin时,mixin的所有选项都会成为组件选项的一部分。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"></div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { }, methods : { }, }); var myMixin = { created: function () { this.startmixin() }, methods: { startmixin: function () { alert("Welcome to mixin example"); } } }; var Component = Vue.extend({ mixins: [myMixin] }) var component = new Component(); </script> </body> </html>
输出

当 mixin 和组件包含重叠选项时,它们会合并,如下例所示。
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"></div> <script type = "text/javascript"> var mixin = { created: function () { console.log('mixin called') } } new Vue({ mixins: [mixin], created: function () { console.log('component called') } }); </script> </body> </html>
现在 mixin 和 vue 实例创建了相同的方法。这是我们在控制台中看到的输出。如图所示,vue 和 mixin 的选项将被合并。

如果方法中碰巧有相同的函数名,那么主 vue 实例将优先。
例子

<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"></div> <script type = "text/javascript"> var mixin = { methods: { hellworld: function () { console.log('In HelloWorld'); }, samemethod: function () { console.log('Mixin:Same Method'); } } }; var vm = new Vue({ mixins: [mixin], methods: { start: function () { console.log('start method'); }, samemethod: function () { console.log('Main: same method'); } } }); vm.hellworld(); vm.start(); vm.samemethod(); </script> </body> </html>
我们将看到 mixin 有一个 method 属性,其中定义了 helloworld 和 Samemethod 函数。类似地,vue 实例有一个methods 属性,其中又定义了两个方法start 和samemethod。
调用以下每个方法。
vm.hellworld(); // In HelloWorld vm.start(); // start method vm.samemethod(); // Main: same method
如上所示,我们调用了 helloworld、start 和 Samemethod 函数。mixin 中也存在 Samemethod,但是,优先级将给予主实例,如以下控制台所示。
