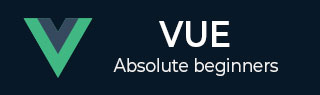
- VueJS Tutorial
- VueJS - Home
- VueJS - Overview
- VueJS - Environment Setup
- VueJS - Introduction
- VueJS - Instances
- VueJS - Template
- VueJS - Components
- VueJS - Computed Properties
- VueJS - Watch Property
- VueJS - Binding
- VueJS - Events
- VueJS - Rendering
- VueJS - Transition & Animation
- VueJS - Directives
- VueJS - Routing
- VueJS - Mixins
- VueJS - Render Function
- VueJS - Reactive Interface
- VueJS - Examples
- VueJS Useful Resources
- VueJS - Quick Guide
- VueJS - Useful Resources
- VueJS - Discussion
VueJS - 渲染
在本章中,我们将学习条件渲染和列表渲染。在条件渲染中,我们将讨论如何使用 if、if-else、if-else-if、show 等。在列表渲染中,我们将讨论如何使用 for 循环。
条件渲染
让我们首先通过一个示例来解释条件渲染的详细信息。使用条件渲染,我们希望仅在满足条件时输出,并且借助 if、if-else、if-else-if、show 等进行条件检查。
v-如果
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"> <button v-on:click = "showdata" v-bind:style = "styleobj">Click Me</button> <span style = "font-size:25px;"><b>{{show}}</b></span> <h1 v-if = "show">This is h1 tag</h1> <h2>This is h2 tag</h2> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { show: true, styleobj: { backgroundColor: '#2196F3!important', cursor: 'pointer', padding: '8px 16px', verticalAlign: 'middle', } }, methods : { showdata : function() { this.show = !this.show; } }, }); </script> </body> </html>
输出

在上面的示例中,我们创建了一个按钮和两个带有消息的 h1 标签。
声明一个名为 show 的变量并将其初始化为值 true。它显示在按钮附近。单击按钮时,我们将调用showdata方法,该方法会切换变量 show 的值。这意味着单击按钮时,变量 show 的值将从 true 更改为 false,然后从 false 更改为 true。
我们已将 if 分配给 h1 标记,如以下代码片段所示。
<button v-on:click = "showdata" v-bind:style = "styleobj">Click Me</button> <h1 v-if = "show">This is h1 tag</h1>
现在它要做的是检查变量 show 的值,如果为 true,则将显示 h1 标签。点击按钮并在浏览器中查看,由于show变量的值变为false,h1标签不会在浏览器中显示。仅当 show 变量为 true 时才显示。
以下是浏览器中的显示。

如果我们在浏览器中检查,这就是 show 为 false 时我们得到的结果。

当变量 show 设置为 false 时,h1 标签将从 DOM 中删除。

这就是当变量为 true 时我们看到的结果。当变量 show 设置为 true 时,h1 标签将添加回 DOM。
v-其他
在下面的示例中,我们将 v-else 添加到第二个 h1 标记。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"> <button v-on:click = "showdata" v-bind:style = "styleobj">Click Me</button> <span style = "font-size:25px;"><b>{{show}}</b></span> <h1 v-if = "show">This is h1 tag</h1> <h2 v-else>This is h2 tag</h2> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { show: true, styleobj: { backgroundColor: '#2196F3!important', cursor: 'pointer', padding: '8px 16px', verticalAlign: 'middle', } }, methods : { showdata : function() { this.show = !this.show; } }, }); </script> </body> </html>
v-else 使用以下代码片段添加。
<h1 v-if = "show">This is h1 tag</h1> <h2 v-else>This is h2 tag</h2>
现在,如果 show 为 true,将显示“这是 h1 标签” ,如果为 false ,将显示“这是 h2 标签” 。这就是我们将在浏览器中得到的内容。

上面的显示是show变量为true时的显示。由于我们添加了 v-else,因此第二个语句不存在。现在,当我们单击按钮时,显示变量将变为 false,并且将显示第二条语句,如下面的屏幕截图所示。

视频秀
v-show 的Behave与 v-if 相同。它还根据分配给它的条件显示和隐藏元素。v-if 和 v-show 之间的区别在于,如果条件为 false,v-if 会从 DOM 中删除 HTML 元素,如果条件为 true,则将其添加回来。而 v-show 则隐藏该元素,如果条件为 false,则使用 display:none。如果条件为真,它会返回元素。因此,该元素始终存在于 dom 中。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"> <button v-on:click = "showdata" v-bind:style = "styleobj">Click Me</button> <span style = "font-size:25px;"><b>{{show}}</b></span> <h1 v-if = "show">This is h1 tag</h1> <h2 v-else>This is h2 tag</h2> <div v-show = "show"> <b>V-Show:</b> <img src = "images/img.jpg" width = "100" height = "100" /> </div> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { show: true, styleobj: { backgroundColor: '#2196F3!important', cursor: 'pointer', padding: '8px 16px', verticalAlign: 'middle', } }, methods : { showdata : function() { this.show = !this.show; } }, }); </script> </body> </html>
使用以下代码片段将 v-show 分配给 HTML 元素。
<div v-show = "show"><b>V-Show:</b><img src = "images/img.jpg" width = "100" height = "100" /></div>
我们使用了相同的变量 show ,并根据它的真/假,图像显示在浏览器中。

现在,由于变量 show 为 true,图像如上面的屏幕截图所示。让我们单击按钮并查看显示。

变量 show 为 false,因此图像被隐藏。如果我们检查并看到该元素,则 div 和图像仍然是 DOM 的一部分,其样式属性为 display: none,如上面的屏幕截图所示。
列表渲染
v-for
现在让我们讨论使用 v-for 指令的列表渲染。
例子
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"> <input type = "text" v-on:keyup.enter = "showinputvalue" v-bind:style = "styleobj" placeholder = "Enter Fruits Names"/> <h1 v-if = "items.length>0">Display Fruits Name</h1> <ul> <li v-for = "a in items">{{a}}</li> </ul> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { items:[], styleobj: { width: "30%", padding: "12px 20px", margin: "8px 0", boxSizing: "border-box" } }, methods : { showinputvalue : function(event) { this.items.push(event.target.value); } }, }); </script> </body> </html>
名为 items 的变量被声明为数组。在方法中,有一个名为showinputvalue的方法,该方法被分配给采用水果名称的输入框。在该方法中,使用以下代码将文本框中输入的水果添加到数组中。
showinputvalue : function(event) { this.items.push(event.target.value); }
我们使用 v-for 来显示输入的水果,如下面的代码所示。V-for 有助于迭代数组中存在的值。
<ul> <li v-for = "a in items">{{a}}</li> </ul>
要使用 for 循环迭代数组,我们必须使用 v-for = ”a in items”,其中 a 保存数组中的值,并将显示直到所有项目完成。
输出
以下是浏览器中的输出。

检查项目时,这就是浏览器中显示的内容。在 DOM 中,我们没有看到任何针对 li 元素的 v-for 指令。它显示没有任何 VueJS 指令的 DOM。

如果我们希望显示数组的索引,可以使用以下代码来完成。
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"> <input type = "text" v-on:keyup.enter = "showinputvalue" v-bind:style = "styleobj" placeholder = "Enter Fruits Names"/> <h1 v-if = "items.length>0">Display Fruits Name</h1> <ul> <li v-for = "(a, index) in items">{{index}}--{{a}}</li> </ul> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { items:[], styleobj: { width: "30%", padding: "12px 20px", margin: "8px 0", boxSizing: "border-box" } }, methods : { showinputvalue : function(event) { this.items.push(event.target.value); } }, }); </script> </body> </html>
为了获取索引,我们在括号中添加了一个变量,如下面的代码所示。
<li v-for = "(a, index) in items">{{index}}--{{a}}</li>
在(a,index)中,a是值,index是键。浏览器显示现在将如以下屏幕截图所示。因此,借助索引,可以显示任何特定值。
