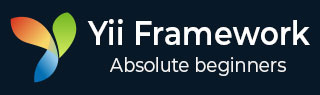
- Yii 教程
- Yii - 主页
- Yii - 概述
- Yii - 安装
- Yii - 创建页面
- Yii - 应用程序结构
- Yii - 入口脚本
- Yii - 控制器
- Yii - 使用控制器
- Yii - 使用动作
- Yii - 模型
- Yii - 小部件
- Yii - 模块
- Yii - 视图
- Yii - 布局
- Yii - 资产
- Yii - 资产转换
- Yii - 扩展
- Yii - 创建扩展
- Yii - HTTP 请求
- Yii - 响应
- Yii - URL 格式
- Yii - URL 路由
- Yii - URL 规则
- Yii - HTML 表单
- Yii - 验证
- Yii - 临时验证
- Yii - AJAX 验证
- Yii - 会话
- Yii - 使用闪存数据
- Yii - cookie
- Yii - 使用 Cookie
- Yii - 文件上传
- Yii - 格式化
- Yii - 分页
- Yii - 排序
- Yii - 属性
- Yii - 数据提供者
- Yii - 数据小部件
- Yii - 列表视图小部件
- Yii - GridView 小部件
- Yii - 活动
- Yii - 创建事件
- Yii - Behave
- Yii - 创建Behave
- Yii - 配置
- Yii - 依赖注入
- Yii - 数据库访问
- Yii - 数据访问对象
- Yii - 查询生成器
- Yii - 活动记录
- Yii - 数据库迁移
- Yii - 主题化
- Yii - RESTful API
- Yii - RESTful API 的实际应用
- Yii - 字段
- Yii - 测试
- Yii - 缓存
- Yii - 片段缓存
- Yii - 别名
- Yii - 日志记录
- Yii - 错误处理
- Yii - 身份验证
- Yii - 授权
- Yii - 本地化
- Yii-Gii
- Gii – 创建模型
- Gii – 生成控制器
- Gii – 生成模块
- Yii 有用的资源
- Yii - 快速指南
- Yii - 有用的资源
- Yii - 讨论
Yii - 字段
通过重写fields() 和 extraFields()方法,您可以定义可以将哪些数据放入响应中。这两种方法之间的区别在于,前者定义了默认字段集,这些字段应包含在响应中,而后者定义了其他字段,如果最终用户通过扩展查询参数请求这些字段,则这些字段可能会包含在响应中。
步骤 1 - 以这种方式修改MyUser模型。
<?php namespace app\models; use app\components\UppercaseBehavior; use Yii; /** * This is the model class for table "user". *@property integer $id * @property string $name * @property string $email */ class MyUser extends \yii\db\ActiveRecord { public function fields() { return [ 'id', 'name', //PHP callback 'datetime' => function($model) { return date("d:m:Y H:i:s"); } ]; } /** * @inheritdoc */ public static function tableName() { return 'user'; } /** * @inheritdoc */ public function rules() { return [ [['name', 'email'], 'string', 'max' => 255] ]; } /** * @inheritdoc */ public function attributeLabels() { return [ 'id' => 'ID', 'name' => 'Name', 'email' => 'Email', ]; } } ?>
除了默认字段: id 和 name 之外,我们还添加了一个自定义字段 - datetime。
步骤 2 - 在 Postman 中,运行 URL http://localhost:8080/users。

步骤 3 - 现在,以这种方式修改MyUser模型。
<?php namespace app\models; use app\components\UppercaseBehavior; use Yii; /** * This is the model class for table "user". * * @property integer $id * @property string $name * @property string $email */ class MyUser extends \yii\db\ActiveRecord { public function fields() { return [ 'id', 'name', ]; } public function extraFields() { return ['email']; } /** * @inheritdoc */ public static function tableName() { return 'user'; } /** * @inheritdoc */ public function rules() { return [ [['name', 'email'], 'string', 'max' => 255] ]; } /** * @inheritdoc */ public function attributeLabels() { return [ 'id' => 'ID', 'name' => 'Name', 'email' => 'Email', ]; } } ?>
请注意,电子邮件字段由extraFields()方法返回。
步骤 4 - 要获取此字段的数据,请运行http://localhost:8080/users?expand=email。

自定义操作
yii \rest\ActiveController类提供以下操作 -
索引- 逐页列出资源
查看- 返回指定资源的详细信息
创建- 创建新资源
更新- 更新现有资源
删除- 删除指定资源
选项- 返回支持的 HTTP 方法
所有上述动作均在 actions method() 中声明。
要禁用“删除”和“创建”操作,请以这种方式修改UserController -
<?php namespace app\controllers; use yii\rest\ActiveController; class UserController extends ActiveController { public $modelClass = 'app\models\MyUser'; public function actions() { $actions = parent::actions(); // disable the "delete" and "create" actions unset($actions['delete'], $actions['create']); return $actions; } } ?>
处理错误
当获取 RESTful API 请求时,如果请求出现错误或者服务器发生意外情况,您可以简单地抛出异常。如果您可以确定错误的原因,则应该抛出异常以及正确的 HTTP 状态代码。Yii REST 使用以下状态 -
200 - 好的。
201 - 已成功创建资源以响应 POST 请求。Location 标头包含指向新创建的资源的 URL。
204 - 请求已成功处理,响应不包含任何内容。
304 - 资源未修改。
400 - 错误的请求。
401 - 身份验证失败。
403 - 不允许经过身份验证的用户访问指定的 API 端点。
404 - 资源不存在。
405 - 方法不允许。
415 - 不支持的媒体类型。
422 - 数据验证失败。
429 - 请求太多。
500 - 内部服务器错误。