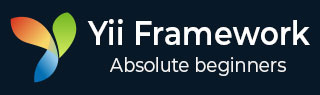
- Yii 教程
- Yii - 主页
- Yii - 概述
- Yii - 安装
- Yii - 创建页面
- Yii - 应用程序结构
- Yii - 入口脚本
- Yii - 控制器
- Yii - 使用控制器
- Yii - 使用动作
- Yii - 模型
- Yii - 小部件
- Yii - 模块
- Yii - 视图
- Yii - 布局
- Yii - 资产
- Yii - 资产转换
- Yii - 扩展
- Yii - 创建扩展
- Yii - HTTP 请求
- Yii - 响应
- Yii - URL 格式
- Yii - URL 路由
- Yii - URL 规则
- Yii - HTML 表单
- Yii - 验证
- Yii - 临时验证
- Yii - AJAX 验证
- Yii - 会话
- Yii - 使用闪存数据
- Yii - cookie
- Yii - 使用 Cookie
- Yii - 文件上传
- Yii - 格式化
- Yii - 分页
- Yii - 排序
- Yii - 属性
- Yii - 数据提供者
- Yii - 数据小部件
- Yii - 列表视图小部件
- Yii - GridView 小部件
- Yii - 活动
- Yii - 创建事件
- Yii - Behave
- Yii - 创建Behave
- Yii - 配置
- Yii - 依赖注入
- Yii - 数据库访问
- Yii - 数据访问对象
- Yii - 查询生成器
- Yii - 活动记录
- Yii - 数据库迁移
- Yii - 主题化
- Yii - RESTful API
- Yii - RESTful API 的实际应用
- Yii - 字段
- Yii - 测试
- Yii - 缓存
- Yii - 片段缓存
- Yii - 别名
- Yii - 日志记录
- Yii - 错误处理
- Yii - 身份验证
- Yii - 授权
- Yii - 本地化
- Yii-Gii
- Gii – 创建模型
- Gii – 生成控制器
- Gii – 生成模块
- Yii 有用的资源
- Yii - 快速指南
- Yii - 有用的资源
- Yii - 讨论
Yii - 验证
您永远不应该相信从用户那里收到的数据。要使用用户输入验证模型,您应该调用yii\base\Model::validate()方法。如果验证成功,它会返回一个布尔值。如果有错误,您可以从yii\base\Model::$errors属性中获取它们。
使用规则
为了使validate()函数正常工作,您应该重写yii\base\Model::rules()方法。
步骤 1 - Rules()方法返回以下格式的数组。
[ // required, specifies which attributes should be validated ['attr1', 'attr2', ...], // required, specifies the type a rule. 'type_of_rule', // optional, defines in which scenario(s) this rule should be applied 'on' => ['scenario1', 'scenario2', ...], // optional, defines additional configurations 'property' => 'value', ... ]
对于每个规则,您至少应该定义该规则应用到哪些属性以及应用的规则类型。
核心验证规则是 -布尔、验证码、比较、日期、默认、双精度、每个、电子邮件、存在、文件、过滤器、图像、ip、in、整数、匹配、数字、必需、安全、字符串、修剪、唯一、网址。
步骤 2 - 在模型文件夹中创建一个新模型。
<?php namespace app\models; use Yii; use yii\base\Model; class RegistrationForm extends Model { public $username; public $password; public $email; public $country; public $city; public $phone; public function rules() { return [ // the username, password, email, country, city, and phone attributes are //required [['username' ,'password', 'email', 'country', 'city', 'phone'], 'required'], // the email attribute should be a valid email address ['email', 'email'], ]; } } ?>
我们已经声明了注册表的模型。该模型有五个属性:用户名、密码、电子邮件、国家/地区、城市和电话。它们都是必需的,并且 email 属性必须是有效的电子邮件地址。
步骤 3 - 将actionRegistration方法添加到 SiteController ,我们在其中创建一个新的RegistrationForm模型并将其传递给视图。
public function actionRegistration() { $model = new RegistrationForm(); return $this->render('registration', ['model' => $model]); }
步骤 4 - 添加注册表单视图。在views/site 文件夹中,使用以下代码创建一个名为registration.php 的文件。
<?php use yii\bootstrap\ActiveForm; use yii\bootstrap\Html; ?> <div class = "row"> <div class = "col-lg-5"> <?php $form = ActiveForm::begin(['id' => 'registration-form']); ?> <?= $form->field($model, 'username') ?> <?= $form->field($model, 'password')->passwordInput() ?> <?= $form->field($model, 'email')->input('email') ?> <?= $form->field($model, 'country') ?> <?= $form->field($model, 'city') ?> <?= $form->field($model, 'phone') ?> <div class = "form-group"> <?= Html::submitButton('Submit', ['class' => 'btn btn-primary', 'name' => 'registration-button']) ?> </div> <?php ActiveForm::end(); ?> </div> </div>
我们使用ActiveForm小部件来显示我们的注册表单。
步骤 5 - 如果您转到本地主机http://localhost:8080/index.php?r=site/registration并单击提交按钮,您将看到正在运行的验证规则。

步骤6 - 要自定义用户名属性的错误消息,请按以下方式修改RegistrationForm的rules()方法。
public function rules() { return [ // the username, password, email, country, city, and phone attributes are required [['password', 'email', 'country', 'city', 'phone'], 'required'], ['username', 'required', 'message' => 'Username is required'], // the email attribute should be a valid email address ['email', 'email'], ]; }
步骤 7 - 转到本地主机http://localhost:8080/index.php?r=site/registration并单击提交按钮。您会注意到用户名属性的错误消息已更改。

步骤 8 - 要自定义验证过程,您可以覆盖这些方法。
yii\base\Model::beforeValidate(): 触发
yii\base\Model::EVENT_BEFORE_VALIDATE 事件。
yii\base\Model::afterValidate(): 触发
yii\base\Model::EVENT_AFTER_VALIDATE 事件。
步骤 9 - 要修剪国家属性周围的空间并将城市属性的空输入变为空,您可以修剪和默认验证器。
public function rules() { return [ // the username, password, email, country, city, and phone attributes are required [['password', 'email', 'country', 'city', 'phone'], 'required'], ['username', 'required', 'message' => 'Username is required'], ['country', 'trim'], ['city', 'default'], // the email attribute should be a valid email address ['email', 'email'], ]; }
步骤 10 - 如果输入为空,您可以为其设置默认值。
public function rules() { return [ ['city', 'default', 'value' => 'Paris'], ]; }
如果城市属性为空,则将使用默认的“巴黎”值。