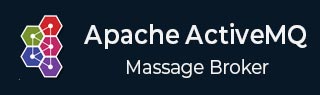
- Apache ActiveMQ 教程
- Apache ActiveMQ - 主页
- Apache ActiveMQ - 概述
- Apache ActiveMQ - 环境设置
- Apache ActiveMQ - 功能
- Apache ActiveMQ - 运行代理服务器
- Apache ActiveMQ - 管理控制台
- 基于 Apache ActiveMQ 队列的示例
- Apache ActiveMQ - 生产者应用程序
- Apache ActiveMQ - 消费者应用程序
- Apache ActiveMQ - 测试应用程序
- Apache ActiveMQ 基于主题的示例
- Apache ActiveMQ - 发布者应用程序
- Apache ActiveMQ - 订阅者应用程序
- Apache ActiveMQ - 测试应用程序
- Apache ActiveMQ 有用资源
- Apache ActiveMQ - 快速指南
- Apache ActiveMQ - 有用的资源
- Apache ActiveMQ - 讨论
Apache ActiveMQ - 发布者应用程序
现在让我们创建一个发布者应用程序,它将向 ActiveMQ 队列发送消息。
创建项目
使用 eclipse,选择File → New → Maven Project。勾选“创建一个简单项目(跳过原型选择)”,然后单击“下一步”。
输入详细信息,如下所示 -
groupId - com.tutorialspoint
artifactId - 发布者
版本- 0.0.1-SNAPSHOT
名称- ActiveMQ 发布者
单击完成按钮,将创建一个新项目。
pom.xml
现在更新 pom.xml 的内容以包含 ActiveMQ 的依赖项。
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint.activemq</groupId> <artifactId>publisher</artifactId> <version>0.0.1-SNAPSHOT</version> <name>ActiveMQ Publisher</name> <dependencies> <dependency> <groupId>org.apache.geronimo.specs</groupId> <artifactId>geronimo-jms_1.1_spec</artifactId> <version>1.1</version> </dependency> <dependency> <groupId>org.apache.qpid</groupId> <artifactId>qpid-jms-client</artifactId> <version>0.40.0</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>2.1</version> <configuration> <source>1.6</source> <target>1.6</target> </configuration> </plugin> <plugin> <groupId>org.fusesource.mvnplugins</groupId> <artifactId>maven-uberize-plugin</artifactId> <version>1.14</version> <executions> <execution> <phase>package</phase> <goals><goal>uberize</goal></goals> </execution> </executions> </plugin> </plugins> </build> </project>
现在创建一个 Publisher 类,它将向 ActiveMQ 主题发送消息,以将其广播给所有订阅者。
package com.tutorialspoint.activemq; import java.io.Console; import java.util.Scanner; import javax.jms.Connection; import javax.jms.Destination; import javax.jms.MessageProducer; import javax.jms.Session; import javax.jms.TextMessage; import org.apache.qpid.jms.JmsConnectionFactory; public class Publisher { public static void main(String[] args) throws Exception { // Create a connection to ActiveMQ JMS broker using AMQP protocol JmsConnectionFactory factory = new JmsConnectionFactory("amqp://localhost:5672"); Connection connection = factory.createConnection("admin", "password"); connection.start(); // Create a session Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE); // Create a topic Destination destination = session.createTopic("MyFirstTopic"); // Create a publisher specific to topic MessageProducer publisher = session.createProducer(destination); Scanner input = new Scanner(System.in); String response; do { System.out.println("Enter message: "); response = input.nextLine(); // Create a message object TextMessage msg = session.createTextMessage(response); // Send the message to the topic publisher.send(msg); } while (!response.equalsIgnoreCase("Quit")); input.close(); // Close the connection connection.close(); } }
生产者类创建一个连接,启动会话,创建一个生产者,然后要求用户输入消息。如果用户输入 quit,则应用程序终止,否则它将向主题发送消息。
我们将在ActiveMQ - 测试应用程序一章中运行此应用程序。