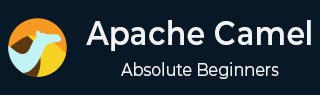
- Apache Camel教程
- Apache Camel - 主页
- Apache Camel - 简介
- Apache Camel - 概述
- Apache Camel - 功能
- Apache Camel - 架构
- Apache Camel - CamelContext
- Apache Camel - 端点
- Apache Camel - 组件
- Apache Camel - 消息队列
- Apache Camel - 项目
- 使用 Camel 和 Spring
- Apache Camel 有用资源
- Apache Camel - 快速指南
- Apache Camel - 有用的资源
- Apache Camel - 讨论
Apache Camel - 与 Spring 一起使用
现在,我们将使用 Spring 重新创建上一章中的应用程序。这将使我们了解如何在 XML 而不是 DSL 中创建 Camel 路由。
创建新项目
创建一个新的Maven项目并指定以下内容 -
GroupId: BasketWithSpring ArtifactId: BasketWithSpring
选择项目的默认位置,或者如果您愿意指定您选择的目录。
添加依赖项
除了在早期应用程序中使用的核心依赖项之外,您还需要添加更多依赖项才能使用 Spring。依赖项添加到 pom.xml 中。现在,打开 pom.xml 并添加以下依赖项 -
<dependencies> ... <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.1.3.RELEASE</version> </dependency> <dependency> <groupId>org.apache.activemq</groupId> <artifactId>activemq-pool</artifactId> <version>5.15.2</version> </dependency> <dependency> <groupId>org.apache.activemq</groupId> <artifactId>activemq-pool</artifactId> <version>5.15.1</version> </dependency> <dependency> <groupId>org.apache.camel</groupId> <artifactId>camel-spring</artifactId> <version>2.15.1</version> </dependency> </dependencies>
为 Spring 创建 Java DSL
现在让我们创建一个名为DistributeOrderXML的新 Java 类。添加以下代码 -
public class DistributeOrderXML { public static void main(String[] args) throws Exception { ApplicationContext appContext = new ClassPathXmlApplicationContext( "SpringRouteContext.xml"); CamelContext camelContext = SpringCamelContext.springCamelContext(appContext, false); try { camelContext.start(); ProducerTemplate orderProducerTemplate = camelContext.createProducerTemplate(); InputStream orderInputStream = new FileInputStream(ClassLoader.getSystemClassLoader() .getResource("order.xml").getFile()); orderProducerTemplate.sendBody("direct:DistributeOrderXML", orderInputStream); } finally { camelContext.stop(); } } }
在main方法中,首先我们创建一个ApplicationContext实例,它是 Spring 应用程序中的中心接口。在其构造函数中,我们指定包含路由和过滤信息的 XML 文件的名称。
ApplicationContext appContext = new ClassPathXmlApplicationContext( "SpringRouteContext.xml");
接下来,我们创建CamelContext,并在其参数中指定上面创建的ApplicationContext。
CamelContext camelContext = SpringCamelContext.springCamelContext(appContext, false);
至此,我们的路由和过滤就设置好了。因此,我们使用CamelContext的start方法来启动它。与前面的情况一样,我们定义用于加载 order.xml 文件的端点并开始处理。现在,让我们了解如何在 XML 中定义路由。
创建应用程序上下文
将新的 XML 文件添加到项目中并将其命名为SpringRouteContext.xml。将以下内容剪切并粘贴到此文件中。
<?xml version = "1.0" encoding = "UTF-8"?> <beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = " http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://camel.apache.org/schema/spring http://camel.apache.org/schema/spring/camel-spring.xsd "> <camelContext xmlns = "http://camel.apache.org/schema/spring"> <route> <from uri = "direct:DistributeOrderXML"/> <log message = "Split by Distribute Order"/> <split> <xpath>//order[@product = 'Oil']/items</xpath> <to uri = "file:src/main/resources/order/"/> <to uri = "stream:out"/> </split> </route> </camelContext> </beans>
在这里,我们定义 xpath 查询如下,请注意,我们现在选择“oil”的所有订单。
<xpath>//order[@product = 'Oil']/items</xpath>
输出端点有多个。第一个端点指定订单文件夹,第二个端点指定控制台。
<to uri = "file:src/main/resources/order/"/> <to uri = "stream:out"/>
运行应用程序。
检测结果
运行该应用程序时,您将在屏幕上看到以下输出。
<items> <item> <Brand>Cinthol</Brand> <Type>Original</Type> <Quantity>4</Quantity> <Price>25</Price> </item> <item> <Brand>Cinthol</Brand> <Type>Lime</Type> <Quantity>6</Quantity> <Price>30</Price> </item> </items>
查看您指定路径下的订单文件夹。您将发现一个新创建的文件,其中包含上述 XML 代码。
结论
Camel 提供了一个可实现 EIP 的即用型框架,以简化您的集成项目。它支持使用特定于领域的语言进行编码以及使用 XML。