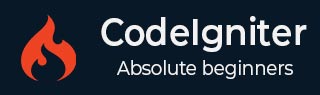
- CodeIgniter 教程
- CodeIgniter - 主页
- CodeIgniter - 概述
- CodeIgniter - 安装 CodeIgniter
- CodeIgniter - 应用程序架构
- CodeIgniter - MVC 框架
- CodeIgniter - 基本概念
- CodeIgniter - 配置
- CodeIgniter - 使用数据库
- CodeIgniter - 库
- CodeIgniter - 错误处理
- CodeIgniter - 文件上传
- CodeIgniter - 发送电子邮件
- CodeIgniter - 表单验证
- CodeIgniter - 会话管理
- CodeIgniter - Flashdata
- CodeIgniter - 临时数据
- CodeIgniter - Cookie 管理
- CodeIgniter - 常用函数
- CodeIgniter - 页面缓存
- CodeIgniter - 页面重定向
- CodeIgniter - 应用程序分析
- CodeIgniter - 基准测试
- CodeIgniter - 添加 JS 和 CSS
- CodeIgniter - 国际化
- CodeIgniter - 安全
- CodeIgniter 有用资源
- CodeIgniter - 快速指南
- CodeIgniter - 有用的资源
- CodeIgniter - 讨论
CodeIgniter - 发送电子邮件
在 CodeIgniter 中发送电子邮件要容易得多。您还可以在 CodeIgniter 中配置有关电子邮件的首选项。CodeIgniter 提供以下用于发送电子邮件的功能 -
- 多种协议 - Mail、Sendmail 和 SMTP
- SMTP 的 TLS 和 SSL 加密
- 多个收件人
- 抄送和密件抄送
- HTML 或纯文本电子邮件
- 附件
- 自动换行
- 优先事项
- BCC 批处理模式,使大型电子邮件列表能够分成小批 BCC。
- 电子邮件调试工具
Email 类具有以下函数来简化发送电子邮件的工作。
序列号 | 句法 | 参数 | 返回 | 返回类型 |
---|---|---|---|---|
1 | from( $from [, $name = '' [, $return_path = NULL ]]) |
$from ( string ) − “发件人”电子邮件地址 $name ( string ) − “发件人”显示名称 $return_path ( string ) - 可选电子邮件地址,用于将未送达的电子邮件重定向到 |
CI_Email 实例(方法链接) | CI_电子邮件 |
2 | 回复($回复[,$名称='' ]) |
$replyto ( string ) - 用于回复的电子邮件地址 $name ( string ) - 回复电子邮件地址的显示名称 |
CI_Email 实例(方法链接) | CI_电子邮件 |
2 | 至($ 至) |
$to ( mixed ) - 逗号分隔的字符串或电子邮件地址数组 |
CI_Email 实例(方法链接) | CI_电子邮件 |
3 | 抄送($抄送) |
$cc ( mixed ) - 逗号分隔的字符串或电子邮件地址数组 |
CI_Email 实例(方法链接) | CI_电子邮件 |
4 | 密件抄送( $密件抄送[, $limit = '' ]) |
$bcc ( mixed ) - 逗号分隔的字符串或电子邮件地址数组 $limit ( int ) - 每批发送的最大电子邮件数 |
CI_Email 实例(方法链接) | CI_电子邮件 |
5 | 主题($主题) |
$subject ( string ) - 电子邮件主题行 |
CI_Email 实例(方法链接) | CI_电子邮件 |
6 | 消息($body) |
$body ( string ) - 电子邮件正文 |
CI_Email 实例(方法链接) | CI_电子邮件 |
7 | set_alt_message( $str ) |
$str ( string ) - 替代电子邮件正文 |
CI_Email 实例(方法链接) | CI_电子邮件 |
8 | set_header( $header, $value ) |
$header ( string ) - 标头名称 $value ( string ) - 标头值 |
CI_Email 实例(方法链接) | CI_电子邮件 |
9 | 清除([ $clear_attachments = FALSE ]) |
$clear_attachments ( bool ) – 是否清除附件 |
CI_Email 实例(方法链接) | CI_电子邮件 |
10 | 发送([ $auto_clear = TRUE ]) |
$auto_clear ( bool ) - 是否自动清除消息数据 |
CI_Email 实例(方法链接) | CI_电子邮件 |
11 | 附加($filename[, $disposition = ''[, $newname = NULL[, $mime = '']]]) |
$filename ( string ) - 文件名 $disposition ( string ) - 附件的“处置”。无论此处使用的 MIME 规范如何,大多数电子邮件客户端都会做出自己的决定。伊安娜 $newname ( string ) - 电子邮件中使用的自定义文件名 $mime ( string ) - 要使用的 MIME 类型(对于缓冲数据有用) |
CI_Email 实例(方法链接) | CI_电子邮件 |
12 | Attachment_cid( $文件名) |
$filename ( string ) - 现有附件文件名 |
附件 Content-ID 或 FALSE(如果未找到) | 细绳 |
发送电子邮件
要使用 CodeIgniter 发送电子邮件,首先您必须使用以下命令加载电子邮件库 -
$this->load->library('email');
加载库后,只需执行以下函数即可设置发送电子邮件所需的元素。from ()函数用于设置 - 电子邮件从何处发送,to()函数用于设置 - 电子邮件发送给谁。subject ()和message()函数用于设置电子邮件的主题和消息。
$this->email->from('your@example.com', 'Your Name'); $this->email->to('someone@example.com'); $this->email->subject('Email Test'); $this->email->message('Testing the email class.');
之后,执行如下所示的send()函数来发送电子邮件。
$this->email->send();
例子
创建一个控制器文件Email_controller.php并将其保存在application/controller/Email_controller.php中。
<?php class Email_controller extends CI_Controller { function __construct() { parent::__construct(); $this->load->library('session'); $this->load->helper('form'); } public function index() { $this->load->helper('form'); $this->load->view('email_form'); } public function send_mail() { $from_email = "your@example.com"; $to_email = $this->input->post('email'); //Load email library $this->load->library('email'); $this->email->from($from_email, 'Your Name'); $this->email->to($to_email); $this->email->subject('Email Test'); $this->email->message('Testing the email class.'); //Send mail if($this->email->send()) $this->session->set_flashdata("email_sent","Email sent successfully."); else $this->session->set_flashdata("email_sent","Error in sending Email."); $this->load->view('email_form'); } } ?>
创建一个名为email_form.php的视图文件并将其保存在application/views/email_form.php
<!DOCTYPE html> <html lang = "en"> <head> <meta charset = "utf-8"> <title>CodeIgniter Email Example</title> </head> <body> <?php echo $this->session->flashdata('email_sent'); echo form_open('/Email_controller/send_mail'); ?> <input type = "email" name = "email" required /> <input type = "submit" value = "SEND MAIL"> <?php echo form_close(); ?> </body> </html>
在application/config/routes.php中的paths.php文件中进行更改,并在文件末尾添加以下行。
$route['email'] = 'Email_Controller';
通过访问以下链接来执行上述示例。将 yoursite.com 替换为您网站的 URL。
http://yoursite.com/index.php/email