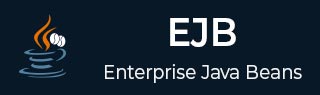
- EJB教程
- EJB - 主页
- EJB - 概述
- EJB - 环境设置
- EJB - 创建应用程序
- EJB-无状态Bean
- EJB - 有状态 Bean
- EJB-持久性
- EJB - 消息驱动 Bean
- EJB - 注释
- EJB-回调
- EJB-定时器服务
- EJB - 依赖注入
- EJB-拦截器
- EJB - 可嵌入对象
- EJB - Blob/Clob
- EJB - 事务
- EJB-安全性
- EJB - JNDI 绑定
- EJB - 实体关系
- EJB - 访问数据库
- EJB - 查询语言
- EJB - 异常处理
- EJB-Web 服务
- EJB - 打包应用程序
- EJB 有用资源
- EJB - 快速指南
- EJB - 有用的资源
- EJB - 讨论
EJB-定时器服务
定时器服务是一种可以构建计划应用程序的机制。例如,每月 1 日生成工资单。EJB 3.0 规范指定了@Timeout 注释,这有助于在无状态或消息驱动的bean 中对EJB 服务进行编程。EJB 容器调用该方法,该方法由@Timeout 注解。
EJB计时器服务是EJB容器提供的服务,它有助于创建计时器并在计时器到期时安排回调。
创建计时器的步骤
使用 @Resource 注释在 bean 中注入 SessionContext -
@Stateless public class TimerSessionBean { @Resource private SessionContext context; ... }
使用 SessionContext 对象获取 TimerService 并创建计时器。传递以毫秒为单位的时间和消息。
public void createTimer(long duration) { context.getTimerService().createTimer(duration, "Hello World!"); }
使用定时器的步骤
对方法使用 @Timeout 注释。返回类型应为 void 并传递 Timer 类型的参数。我们将在第一次执行后取消计时器,否则它将在固定间隔后继续运行。
@Timeout public void timeOutHandler(Timer timer) { System.out.println("timeoutHandler : " + timer.getInfo()); timer.cancel(); }
应用示例
让我们创建一个测试 EJB 应用程序来测试 EJB 中的计时器服务。
步 | 描述 |
---|---|
1 | 按照EJB - 创建应用程序一章中的说明,在com.tutorialspoint.timer包下创建一个名为EjbComponent的项目。 |
2 | 按照EJB - 创建应用程序一章中的说明创建TimerSessionBean.java和TimerSessionBeanRemote。保持其余文件不变。 |
3 | 清理并构建应用程序,以确保业务逻辑按照要求运行。 |
4 | 最后,将应用程序以jar文件的形式部署在JBoss应用服务器上。如果 JBoss 应用服务器尚未启动,它将自动启动。 |
5 | 现在创建 EJB 客户端,这是一个基于控制台的应用程序,其方式与EJB - 创建应用程序一章中创建客户端以访问 EJB主题下所述的方式相同。 |
EJB组件(EJB模块)
TimerSessionBean.java
package com.tutorialspoint.timer; import javax.annotation.Resource; import javax.ejb.SessionContext; import javax.ejb.Timer; import javax.ejb.Stateless; import javax.ejb.Timeout; @Stateless public class TimerSessionBean implements TimerSessionBeanRemote { @Resource private SessionContext context; public void createTimer(long duration) { context.getTimerService().createTimer(duration, "Hello World!"); } @Timeout public void timeOutHandler(Timer timer) { System.out.println("timeoutHandler : " + timer.getInfo()); timer.cancel(); } }
TimerSessionBeanRemote.java
package com.tutorialspoint.timer; import javax.ejb.Remote; @Remote public interface TimerSessionBeanRemote { public void createTimer(long milliseconds); }
在 JBOSS 上部署 EjbComponent 项目后,请注意 jboss 日志。
JBoss 已经自动为我们的会话 bean 创建了一个 JNDI 条目 - TimerSessionBean/remote。
我们将使用此查找字符串来获取类型为 - com.tutorialspoint.timer.TimerSessionBeanRemote 的远程业务对象
JBoss应用服务器日志输出
... 16:30:01,401 INFO [JndiSessionRegistrarBase] Binding the following Entries in Global JNDI: TimerSessionBean/remote - EJB3.x Default Remote Business Interface TimerSessionBean/remote-com.tutorialspoint.timer.TimerSessionBeanRemote - EJB3.x Remote Business Interface 16:30:02,723 INFO [SessionSpecContainer] Starting jboss.j2ee:jar=EjbComponent.jar,name=TimerSessionBean,service=EJB3 16:30:02,723 INFO [EJBContainer] STARTED EJB: com.tutorialspoint.timer.TimerSessionBeanRemote ejbName: TimerSessionBean ...
EJBTester(EJB 客户端)
jndi.属性
java.naming.factory.initial=org.jnp.interfaces.NamingContextFactory java.naming.factory.url.pkgs=org.jboss.naming:org.jnp.interfaces java.naming.provider.url=localhost
这些属性用于初始化java命名服务的InitialContext对象。
InitialContext 对象将用于查找无状态会话 bean。
EJBTester.java
package com.tutorialspoint.test; import com.tutorialspoint.stateful.TimerSessionBeanRemote; import java.io.BufferedReader; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStreamReader; import java.util.List; import java.util.Properties; import javax.naming.InitialContext; import javax.naming.NamingException; public class EJBTester { BufferedReader brConsoleReader = null; Properties props; InitialContext ctx; { props = new Properties(); try { props.load(new FileInputStream("jndi.properties")); } catch (IOException ex) { ex.printStackTrace(); } try { ctx = new InitialContext(props); } catch (NamingException ex) { ex.printStackTrace(); } brConsoleReader = new BufferedReader(new InputStreamReader(System.in)); } public static void main(String[] args) { EJBTester ejbTester = new EJBTester(); ejbTester.testTimerService(); } private void showGUI() { System.out.println("**********************"); System.out.println("Welcome to Book Store"); System.out.println("**********************"); System.out.print("Options \n1. Add Book\n2. Exit \nEnter Choice: "); } private void testTimerService() { try { TimerSessionBeanRemote timerServiceBean = (TimerSessionBeanRemote)ctx.lookup("TimerSessionBean/remote"); System.out.println("["+(new Date()).toString()+ "]" + "timer created."); timerServiceBean.createTimer(2000); } catch (NamingException ex) { ex.printStackTrace(); } } }
EJBTester 正在执行以下任务。
从 jndi.properties 加载属性并初始化 InitialContext 对象。
在 testTimerService() 方法中,使用名称“TimerSessionBean/remote”进行 jndi 查找,以获取远程业务对象(计时器无状态 EJB)。
然后调用 createTimer,并传递 2000 毫秒作为计划时间。
EJB 容器在 2 秒后调用 timeoutHandler 方法。
运行客户端访问EJB
在项目资源管理器中找到 EJBTester.java。右键单击 EJBTester 类并选择运行文件。
在 Netbeans 控制台中验证以下输出。
run: [Wed Jun 19 11:35:47 IST 2013]timer created. BUILD SUCCESSFUL (total time: 0 seconds)
JBoss应用服务器日志输出
您可以在JBoss日志中找到以下回调条目
... 11:35:49,555 INFO [STDOUT] timeoutHandler : Hello World! ...