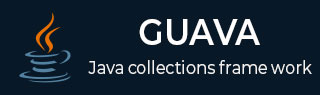
- Guava Tutorial
- Guava - Home
- Guava - Overview
- Guava - Environment Setup
- Guava - Optional Class
- Guava - Preconditions Class
- Guava - Ordering Class
- Guava - Objects Class
- Guava - Range Class
- Guava - Throwables Class
- Guava - Collections Utilities
- Guava - Caching Utilities
- Guava - String Utilities
- Guava - Primitive Utilities
- Guava - Math Utilities
- Guava Useful Resources
- Guava - Quick Guide
- Guava - Useful Resources
- Guava - Discussion
番石榴 - 可选课程
Optional 是一个不可变对象,用于包含非空对象。可选对象用于表示缺少值的 null。此类具有各种实用方法,可帮助代码将值处理为可用或不可用,而不是检查空值。
类别声明
以下是com.google.common.base.Optional<T>类的声明-
@GwtCompatible(serializable = true) public abstract class Optional<T> extends Object implements Serializable
类方法
先生编号 | 方法及说明 |
---|---|
1 | 静态 <T> 可选 <T> 缺席() 返回一个不包含引用的可选实例。 |
2 | 抽象 Set<T> asSet() 返回一个不可变的单例 Set,其唯一元素是包含的实例(如果存在);否则是一个空的不可变 Set。 |
3 | 抽象布尔等于(对象对象) 如果 object 是可选实例,并且所包含的引用彼此相等或两者都不存在,则返回 true。 |
4 | static <T> 可选 <T> fromNullable(T nullableReference) 如果 nullableReference 不为 null,则返回包含该引用的可选实例;否则返回absent()。 |
5 | 抽象 T get() 返回包含的实例,该实例必须存在。 |
6 | 抽象 int hashCode() 返回此实例的哈希码。 |
7 | 抽象布尔值 isPresent() 如果此持有者包含一个(非空)实例,则返回 true。 |
8 | static <T> 可选<T> of(T 引用) 返回包含给定非空引用的可选实例。 |
9 | 抽象可选<T>或(可选<?扩展T>第二个选择) 如果存在值,则返回此可选;否则第二个选择。 |
10 | 抽象 T 或(供应商 <? 扩展 T> 供应商) 如果存在则返回包含的实例;否则供应商.get()。 |
11 | 抽象 T 或(T 默认值) 如果存在则返回包含的实例;否则为默认值。 |
12 | 抽象 T 或 Null() 如果存在则返回包含的实例;否则为 null。 |
13 | static <T> Iterable<T>presentInstances(Iterable<?extendsOptional<?extendsT>>可选) 按顺序从提供的选项中返回每个存在实例的值,跳过出现的absent()。 |
14 | 抽象字符串 toString() 返回此实例的字符串表示形式。 |
15 | 抽象 <V> 可选 <V> 转换(函数 <? super T,V> 函数) 如果实例存在,则使用给定的 Function 对其进行转换;否则,返回absent()。 |
继承的方法
该类继承了以下类的方法 -
- java.lang.Object
可选类示例
使用您选择的任何编辑器(例如C:/> Guava)创建以下 java 程序。
GuavaTester.java
import com.google.common.base.Optional; public class GuavaTester { public static void main(String args[]) { GuavaTester guavaTester = new GuavaTester(); Integer value1 = null; Integer value2 = new Integer(10); //Optional.fromNullable - allows passed parameter to be null. Optional<Integer> a = Optional.fromNullable(value1); //Optional.of - throws NullPointerException if passed parameter is null Optional<Integer> b = Optional.of(value2); System.out.println(guavaTester.sum(a,b)); } public Integer sum(Optional<Integer> a, Optional<Integer> b) { //Optional.isPresent - checks the value is present or not System.out.println("First parameter is present: " + a.isPresent()); System.out.println("Second parameter is present: " + b.isPresent()); //Optional.or - returns the value if present otherwise returns //the default value passed. Integer value1 = a.or(new Integer(0)); //Optional.get - gets the value, value should be present Integer value2 = b.get(); return value1 + value2; } }
验证结果
使用javac编译器编译该类,如下所示 -
C:\Guava>javac GuavaTester.java
现在运行 GuavaTester 查看结果。
C:\Guava>java GuavaTester
查看结果。
First parameter is present: false Second parameter is present: true 10