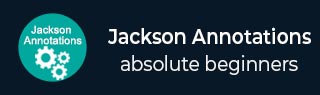
- Jackson 注释教程
- Jackson - 主页
- 序列化注解
- Jackson - @JsonAnyGetter
- Jackson - @JsonGetter
- @JsonPropertyOrder
- Jackson - @JsonRawValue
- Jackson-@JsonValue
- Jackson - @JsonRootName
- Jackson - @JsonSerialize
- 反序列化注解
- Jackson - @JsonCreator
- Jackson - @JacksonInject
- Jackson - @JsonAnySetter
- Jackson-@JsonSetter
- Jackson - @JsonDeserialize
- @JsonEnumDefaultValue
- 属性包含注释
- @JsonIgnoreProperties
- Jackson - @JsonIgnore
- Jackson - @JsonIgnoreType
- Jackson-@JsonIninclude
- Jackson - @JsonAutoDetect
- 类型处理注解
- Jackson - @JsonTypeInfo
- Jackson - @JsonSubTypes
- Jackson - @JsonTypeName
- 一般注释
- Jackson - @JsonProperty
- Jackson-@JsonFormat
- Jackson - @JsonUnwrapped
- Jackson-@JsonView
- @JsonManagedReference
- @JsonBackReference
- Jackson - @JsonIdentityInfo
- Jackson-@JsonFilter
- 各种各样的
- 自定义注释
- 混合注释
- 禁用注释
- Jackson 注释资源
- Jackson - 快速指南
- Jackson - 有用的资源
- Jackson - 讨论
Jackson 注释 - 快速指南
Jackson注释 - @JsonAnyGetter
@JsonAnyGetter允许 getter 方法返回 Map,然后使用该 Map 以与其他属性类似的方式序列化 JSON 的附加属性。
没有@JsonAnyGetter的示例
import java.io.IOException; import java.util.HashMap; import java.util.Map; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try{ Student student = new Student(); student.add("Name", "Mark"); student.add("RollNo", "1"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { private Map<String, String> properties; public Student(){ properties = new HashMap<>(); } public Map<String, String> getProperties(){ return properties; } public void add(String property, String value){ properties.put(property, value); } }
输出
{ "properties" : { "RollNo" : "1", "Name" : "Mark" } }
@JsonAnyGetter 的示例
import java.io.IOException; import java.util.HashMap; import java.util.Map; import com.fasterxml.jackson.annotation.JsonAnyGetter; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try{ Student student = new Student(); student.add("Name", "Mark"); student.add("RollNo", "1"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { private Map<String, String> properties; public Student(){ properties = new HashMap<>(); } @JsonAnyGetter public Map<String, String> getProperties(){ return properties; } public void add(String property, String value){ properties.put(property, value); } }
输出
{ "RollNo" : "1", "Name" : "Mark" }
Jackson注释 - @JsonGetter
@JsonGetter允许将特定方法标记为 getter 方法。
没有@JsonGetter的示例
import java.io.IOException; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student("Mark", 1); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { private String name; private int rollNo; public Student(String name, int rollNo){ this.name = name; this.rollNo = rollNo; } public String getStudentName(){ return name; } public int getRollNo(){ return rollNo; } }
输出
{ "studentName" : "Mark", "rollNo" : 1 }
@JsonGetter 的示例
import java.io.IOException; import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.annotation.JsonGetter; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student("Mark", 1); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { private String name; private int rollNo; public Student(String name, int rollNo){ this.name = name; this.rollNo = rollNo; } @JsonGetter public String getStudentName(){ return name; } public int getRollNo(){ return rollNo; } }
输出
{ "name" : "Mark", "rollNo" : 1 }
Jackson注释 - @JsonPropertyOrder
@JsonPropertyOrder允许在序列化 JSON 对象时保留特定的顺序。
没有@JsonPropertyOrder的示例
import java.io.IOException; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student("Mark", 1); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { private String name; private int rollNo; public Student(String name, int rollNo) { this.name = name; this.rollNo = rollNo; } public String getName(){ return name; } public int getRollNo(){ return rollNo; } }
输出
{ "name" : "Mark", "rollNo" : 1 }
示例@JsonPropertyOrder
import java.io.IOException; import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.annotation.JsonPropertyOrder; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student("Mark", 1); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } @JsonPropertyOrder({ "rollNo", "name" }) class Student { private String name; private int rollNo; public Student(String name, int rollNo){ this.name = name; this.rollNo = rollNo; } public String getName(){ return name; } public int getRollNo(){ return rollNo; } }
输出
{ "name" : "Mark", "rollNo" : 1 }
Jackson注释 - @JsonRawValue
@JsonRawValue允许序列化文本而无需转义或没有任何修饰。
没有@JsonRawValue的示例
import java.io.IOException; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student("Mark", 1, "{\"attr\":false}"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { private String name; private int rollNo; private String json; public Student(String name, int rollNo, String json){ this.name = name; this.rollNo = rollNo; this.json = json; } public String getName(){ return name; } public int getRollNo(){ return rollNo; } public String getJson(){ return json; } }
输出
{ "name" : "Mark", "rollNo" : 1, "json" : {\"attr\":false} }
@JsonRawValue 的示例
import java.io.IOException; import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.annotation.JsonRawValue; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student("Mark", 1, "{\"attr\":false}"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { private String name; private int rollNo; @JsonRawValue private String json; public Student(String name, int rollNo, String json) { this.name = name; this.rollNo = rollNo; this.json = json; } public String getName(){ return name; } public int getRollNo(){ return rollNo; } public String getJson(){ return json; } }
输出
{ "name" : "Mark", "rollNo" : 1, "json" : {"attr":false} }
Jackson注释 - @JsonValue
@JsonValue允许使用其单一方法序列化整个对象。
示例@JsonValue
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonValue; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student("Mark", 1); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { private String name; private int rollNo; public Student(String name, int rollNo){ this.name = name; this.rollNo = rollNo; } public String getName(){ return name; } public int getRollNo(){ return rollNo; } @JsonValue public String toString(){ return "{ name : " + name + " }"; } }
输出
"{ name : Mark }"
Jackson注释 - @JsonRootName
@JsonRootName允许通过 JSON 指定根节点。我们还需要启用环绕根值。
示例@JsonRootName
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonRootName; import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.databind.SerializationFeature; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student("Mark", 1); mapper.enable(SerializationFeature.WRAP_ROOT_VALUE); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } @JsonRootName(value = "student") class Student { private String name; private int rollNo; public Student(String name, int rollNo){ this.name = name; this.rollNo = rollNo; } public String getName(){ return name; } public int getRollNo(){ return rollNo; } }
输出
{ "student" : { "name" : "Mark", "rollNo" : 1 } }
Jackson注释 - @JsonSerialize
@JsonSerialize用于指定自定义序列化器来编组 json 对象。
@JsonSerialize 的示例
import java.io.IOException; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; import com.fasterxml.jackson.core.JsonGenerator; import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.databind.SerializerProvider; import com.fasterxml.jackson.databind.annotation.JsonSerialize; import com.fasterxml.jackson.databind.ser.std.StdSerializer; public class JacksonTester { public static void main(String args[]) throws ParseException { ObjectMapper mapper = new ObjectMapper(); SimpleDateFormat dateFormat = new SimpleDateFormat("dd-MM-yyyy"); try { Student student = new Student("Mark", 1, dateFormat.parse("20-11-1984")); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { private String name; private int rollNo; @JsonSerialize(using = CustomDateSerializer.class) private Date dateOfBirth; public Student(String name, int rollNo, Date dob){ this.name = name; this.rollNo = rollNo; this.dateOfBirth = dob; } public String getName(){ return name; } public int getRollNo(){ return rollNo; } public Date getDateOfBirth(){ return dateOfBirth; } } class CustomDateSerializer extends StdSerializer<Date> { private static final long serialVersionUID = 1L; private static SimpleDateFormat formatter = new SimpleDateFormat("dd-MM-yyyy"); public CustomDateSerializer() { this(null); } public CustomDateSerializer(Class<Date> t) { super(t); } @Override public void serialize(Date value, JsonGenerator generator, SerializerProvider arg2) throws IOException { generator.writeString(formatter.format(value)); } }
输出
{ "name" : "Mark", "rollNo" : 1, "dateOfBirth" : "20-11-1984" }
Jackson注释 - @JsonCreator
@JsonCreator用于微调反序列化中使用的构造函数或工厂方法。我们也将使用 @JsonProperty 来实现相同的目的。在下面的示例中,我们通过定义所需的属性名称将不同格式的 json 与我们的类匹配。
示例@JsonCreator
import java.io.IOException; import java.text.ParseException; import com.fasterxml.jackson.annotation.JsonCreator; import com.fasterxml.jackson.annotation.JsonProperty; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws ParseException{ String json = "{\"id\":1,\"theName\":\"Mark\"}"; ObjectMapper mapper = new ObjectMapper(); try { Student student = mapper .readerFor(Student.class) .readValue(json); System.out.println(student.rollNo +", " + student.name); } catch (IOException e) { e.printStackTrace(); } } } class Student { public String name; public int rollNo; @JsonCreator public Student(@JsonProperty("theName") String name, @JsonProperty("id") int rollNo){ this.name = name; this.rollNo = rollNo; } }
输出
1, Mark
Jackson注释 - @JacksonInject
当要注入属性值而不是从 Json 输入解析属性值时,使用@JacksonInject 。在下面的示例中,我们将一个值插入到对象中,而不是从 Json 中解析。
示例@JacksonInject
import java.io.IOException; import java.text.ParseException; import com.fasterxml.jackson.annotation.JacksonInject; import com.fasterxml.jackson.databind.InjectableValues; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws ParseException{ String json = "{\"name\":\"Mark\"}"; InjectableValues injectableValues = new InjectableValues.Std() .addValue(int.class, 1); ObjectMapper mapper = new ObjectMapper(); try { Student student = mapper .reader(injectableValues) .forType(Student.class) .readValue(json); System.out.println(student.rollNo +", " + student.name); } catch (IOException e) { e.printStackTrace(); } } } class Student { public String name; @JacksonInject public int rollNo; }
输出
1, Mark
Jackson注释 - @JsonAnySetter
@JsonAnySetter允许 setter 方法使用 Map,然后使用 Map 以与其他属性类似的方式反序列化 JSON 的附加属性。
示例@JsonAnySetter
import java.io.IOException; import java.util.HashMap; import java.util.Map; import com.fasterxml.jackson.annotation.JsonAnySetter; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); String jsonString = "{\"RollNo\" : \"1\",\"Name\" : \"Mark\"}"; try { Student student = mapper.readerFor(Student.class).readValue(jsonString); System.out.println(student.getProperties().get("Name")); System.out.println(student.getProperties().get("RollNo")); } catch (IOException e) { e.printStackTrace(); } } } class Student { private Map<String, String> properties; public Student(){ properties = new HashMap<>(); } public Map<String, String> getProperties(){ return properties; } @JsonAnySetter public void add(String property, String value){ properties.put(property, value); } }
输出
Mark 1
Jackson注释 - @JsonSetter
@JsonSetter允许将特定方法标记为 setter 方法。
示例@JsonSetter
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonSetter; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); String jsonString = "{\"rollNo\":1,\"name\":\"Marks\"}"; try { Student student = mapper.readerFor(Student.class).readValue(jsonString); System.out.println(student.name); } catch (IOException e) { e.printStackTrace(); } } } class Student { public int rollNo; public String name; @JsonSetter("name") public void setTheName(String name) { this.name = name; } }
输出
Marks
Jackson注释 - @JsonDeserialize
@JsonDeserialize用于指定自定义反序列化器来解组 json 对象。
示例@JsonDeserialize
import java.io.IOException; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; import com.fasterxml.jackson.core.JsonParser; import com.fasterxml.jackson.core.JsonProcessingException; import com.fasterxml.jackson.databind.DeserializationContext; import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.databind.annotation.JsonDeserialize; import com.fasterxml.jackson.databind.deser.std.StdDeserializer; public class JacksonTester { public static void main(String args[]) throws ParseException{ ObjectMapper mapper = new ObjectMapper(); String jsonString = "{\"name\":\"Mark\",\"dateOfBirth\":\"20-12-1984\"}"; try { Student student = mapper .readerFor(Student.class) .readValue(jsonString); System.out.println(student.dateOfBirth); } catch (IOException e) { e.printStackTrace(); } } } class Student { public String name; @JsonDeserialize(using = CustomDateDeserializer.class) public Date dateOfBirth; } class CustomDateDeserializer extends StdDeserializer<Date> { private static final long serialVersionUID = 1L; private static SimpleDateFormat formatter = new SimpleDateFormat("dd-MM-yyyy"); public CustomDateDeserializer() { this(null); } public CustomDateDeserializer(Class<Date> t) { super(t); } @Override public Date deserialize(JsonParser parser, DeserializationContext context) throws IOException, JsonProcessingException { String date = parser.getText(); try { return formatter.parse(date); } catch (ParseException e) { e.printStackTrace(); } return null; } }
输出
Thu Dec 20 00:00:00 IST 1984
Jackson注释 - @JsonEnumDefaultValue
@JsonEnumDefaultValue用于使用默认值反序列化未知的枚举值。
示例@JsonEnumDefaultValue
import java.io.IOException; import java.text.ParseException; import com.fasterxml.jackson.annotation.JsonEnumDefaultValue; import com.fasterxml.jackson.databind.DeserializationFeature; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws ParseException{ ObjectMapper mapper = new ObjectMapper(); mapper.enable(DeserializationFeature.READ_UNKNOWN_ENUM_VALUES_USING_DEFAULT_VALUE); String jsonString = "\"abc\""; try { LETTERS value = mapper.readValue(jsonString, LETTERS.class); System.out.println(value); } catch (IOException e) { e.printStackTrace(); } } } enum LETTERS { A, B, @JsonEnumDefaultValue UNKNOWN }
输出
UNKNOWN
Jackson注释 - @JsonIgnoreProperties
@JsonIgnoreProperties在类级别使用来标记要忽略的属性或属性列表。
示例 - @JsonIgnoreProperties
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonIgnoreProperties; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) { ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student(1,11,"1ab","Mark"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } @JsonIgnoreProperties({ "id", "systemId" }) class Student { public int id; public String systemId; public int rollNo; public String name; Student(int id, int rollNo, String systemId, String name){ this.id = id; this.systemId = systemId; this.rollNo = rollNo; this.name = name; } }
输出
{ "rollNo" : 11, "name" : "Mark" }
Jackson注释 - @JsonIgnore
@JsonIgnore在字段级别使用来标记要忽略的属性或属性列表。
示例 - @JsonIgnore
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonIgnore; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try{ Student student = new Student(1,11,"1ab","Mark"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { public int id; @JsonIgnore public String systemId; public int rollNo; public String name; Student(int id, int rollNo, String systemId, String name){ this.id = id; this.systemId = systemId; this.rollNo = rollNo; this.name = name; } }
输出
{ "id" : 1, "rollNo" : 11, "name" : "Mark" }
Jackson注释 - @JsonIgnoreType
@JsonIgnoreType用于标记要忽略的特殊类型的属性。
示例 - @JsonIgnoreType
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonIgnoreType; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student(1,11,"1ab","Mark"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { public int id; @JsonIgnore public String systemId; public int rollNo; public Name nameObj; Student(int id, int rollNo, String systemId, String name){ this.id = id; this.systemId = systemId; this.rollNo = rollNo; nameObj = new Name(name); } @JsonIgnoreType class Name { public String name; Name(String name){ this.name = name; } } }
输出
{ "id" : 1, "systemId" : "1ab", "rollNo" : 11 }
Jackson注释 - @JsonIninclude
@JsonInclude用于排除具有 null/空或默认值的属性。
示例 - @JsonIninclude
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonInclude; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student(1,null); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } @JsonInclude(JsonInclude.Include.NON_NULL) class Student { public int id; public String name; Student(int id,String name){ this.id = id; this.name = name; } }
输出
{ "id" : 1 }
Jackson注释 - @JsonAutoDetect
@JsonAutoDetect可用于包含否则无法访问的属性。
示例 - @JsonAutoDetect
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonAutoDetect; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try{ Student student = new Student(1,"Mark"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } @JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.ANY) class Student { private int id; private String name; Student(int id,String name) { this.id = id; this.name = name; } }
输出
{ "id" : 1, "name" : "Mark" }
Jackson注释 - @JsonTypeInfo
@JsonTypeInfo用于指示序列化和反序列化中要包含的类型信息的详细信息。
示例 - @JsonTypeInfo
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonSubTypes; import com.fasterxml.jackson.annotation.JsonTypeInfo; import com.fasterxml.jackson.annotation.JsonTypeInfo.As; import com.fasterxml.jackson.annotation.JsonTypeName; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException { Shape shape = new JacksonTester.Circle("CustomCircle", 1); String result = new ObjectMapper() .writerWithDefaultPrettyPrinter() .writeValueAsString(shape); System.out.println(result); String json = "{\"name\":\"CustomCircle\",\"radius\":1.0, \"type\":\"circle\"}"; Circle circle = new ObjectMapper().readerFor(Shape.class).readValue(json); System.out.println(circle.name); } @JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = As.PROPERTY, property = "type") @JsonSubTypes({ @JsonSubTypes.Type(value = Square.class, name = "square"), @JsonSubTypes.Type(value = Circle.class, name = "circle") }) static class Shape { public String name; Shape(String name){ this.name = name; } } @JsonTypeName("square") static class Square extends Shape { public double length; Square(){ this(null,0.0); } Square(String name, double length){ super(name); this.length = length; } } @JsonTypeName("circle") static class Circle extends Shape { public double radius; Circle(){ this(null,0.0); } Circle(String name, double radius) { super(name); this.radius = radius; } } }
输出
{ "type" : "circle", "name" : "CustomCircle", "radius" : 1.0 } CustomCircle
Jackson注释 - @JsonSubTypes
@JsonSubTypes用于指示注释类型的子类型。
示例 - @JsonSubTypes
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonSubTypes; import com.fasterxml.jackson.annotation.JsonTypeInfo; import com.fasterxml.jackson.annotation.JsonTypeInfo.As; import com.fasterxml.jackson.annotation.JsonTypeName; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException{ Shape shape = new JacksonTester.Circle("CustomCircle", 1); String result = new ObjectMapper() .writerWithDefaultPrettyPrinter() .writeValueAsString(shape); System.out.println(result); String json = "{\"name\":\"CustomCircle\",\"radius\":1.0, \"type\":\"circle\"}"; Circle circle = new ObjectMapper().readerFor(Shape.class).readValue(json); System.out.println(circle.name); } @JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = As.PROPERTY, property = "type") @JsonSubTypes({ @JsonSubTypes.Type(value = Square.class, name = "square"), @JsonSubTypes.Type(value = Circle.class, name = "circle") }) static class Shape { public String name; Shape(String name) { this.name = name; } } @JsonTypeName("square") static class Square extends Shape { public double length; Square(){ this(null,0.0); } Square(String name, double length){ super(name); this.length = length; } } @JsonTypeName("circle") static class Circle extends Shape { public double radius; Circle(){ this(null,0.0); } Circle(String name, double radius){ super(name); this.radius = radius; } } }
输出
{ "type" : "circle", "name" : "CustomCircle", "radius" : 1.0 } CustomCircle
Jackson注释 - @JsonTypeName
@JsonTypeName用于设置用于带注释的类的类型名称。
示例 - @JsonTypeName
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonSubTypes; import com.fasterxml.jackson.annotation.JsonTypeInfo; import com.fasterxml.jackson.annotation.JsonTypeInfo.As; import com.fasterxml.jackson.annotation.JsonTypeName; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException { Shape shape = new JacksonTester.Circle("CustomCircle", 1); String result = new ObjectMapper() .writerWithDefaultPrettyPrinter() .writeValueAsString(shape); System.out.println(result); String json = "{\"name\":\"CustomCircle\",\"radius\":1.0, \"type\":\"circle\"}"; Circle circle = new ObjectMapper().readerFor(Shape.class).readValue(json); System.out.println(circle.name); } @JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = As.PROPERTY, property = "type") @JsonSubTypes({ @JsonSubTypes.Type(value = Square.class, name = "square"), @JsonSubTypes.Type(value = Circle.class, name = "circle") }) static class Shape { public String name; Shape(String name){ this.name = name; } } @JsonTypeName("square") static class Square extends Shape { public double length; Square(){ this(null,0.0); } Square(String name, double length){ super(name); this.length = length; } } @JsonTypeName("circle") static class Circle extends Shape { public double radius; Circle(){ this(null,0.0); } Circle(String name, double radius){ super(name); this.radius = radius; } } }
输出
{ "type" : "circle", "name" : "CustomCircle", "radius" : 1.0 } CustomCircle
Jackson注释 - @JsonProperty
@JsonProperty用于标记针对 json 属性使用的非标准 getter/setter 方法。
示例 - @JsonProperty
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonProperty; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException { ObjectMapper mapper = new ObjectMapper(); String json = "{\"id\" : 1}"; Student student = mapper.readerFor(Student.class).readValue(json); System.out.println(student.getTheId()); } } class Student { private int id; Student(){} Student(int id){ this.id = id; } @JsonProperty("id") public int getTheId() { return id; } @JsonProperty("id") public void setTheId(int id) { this.id = id; } }
输出
1
Jackson注释 - @JsonFormat
@JsonFormat用于在序列化或反序列化时指定格式。它主要与日期字段一起使用。
示例 - @JsonFormat
import java.io.IOException; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; import com.fasterxml.jackson.annotation.JsonFormat; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException, ParseException { ObjectMapper mapper = new ObjectMapper(); SimpleDateFormat simpleDateFormat = new SimpleDateFormat("dd-MM-yyyy"); Date date = simpleDateFormat.parse("20-12-1984"); Student student = new Student(1, date); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } } class Student { public int id; @JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "dd-MM-yyyy") public Date birthDate; Student(int id, Date birthDate){ this.id = id; this.birthDate = birthDate; } }
输出
{ "id" : 1, "birthDate" : "19-12-1984" }
Jackson注释 - @JsonUnwrapped
@JsonUnwrapped用于在序列化或反序列化期间解开对象的值。
示例 - @JsonUnwrapped
import java.io.IOException; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; import com.fasterxml.jackson.annotation.JsonUnwrapped; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException, ParseException{ ObjectMapper mapper = new ObjectMapper(); SimpleDateFormat simpleDateFormat = new SimpleDateFormat("dd-MM-yyyy"); Date date = simpleDateFormat.parse("20-12-1984"); Student.Name name = new Student.Name(); name.first = "Jane"; name.last = "Doe"; Student student = new Student(1, name); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } } class Student { public int id; @JsonUnwrapped public Name name; Student(int id, Name name){ this.id = id; this.name = name; } static class Name { public String first; public String last; } }
输出
{ "id" : 1, "first" : "Jane", "last" : "Doe" }
Jackson注释 - @JsonView
@JsonView用于控制值是否被序列化。
示例 - @JsonView
import java.io.IOException; import java.text.ParseException; import com.fasterxml.jackson.annotation.JsonView; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException, ParseException { ObjectMapper mapper = new ObjectMapper(); Student student = new Student(1, "Mark", 12); String jsonString = mapper .writerWithDefaultPrettyPrinter() .withView(Views.Public.class) .writeValueAsString(student); System.out.println(jsonString); } } class Student { @JsonView(Views.Public.class) public int id; @JsonView(Views.Public.class) public String name; @JsonView(Views.Internal.class) public int age; Student(int id, String name, int age) { this.id = id; this.name = name; this.age = age; } } class Views { static class Public {} static class Internal extends Public {} }
输出
{ "id" : 1, "name" : "Mark" }
Jackson注释 - @JsonManagedReference
@JsonManagedReferences和JsonBackReferences用于显示具有父子关系的对象。@JsonManagedReferences用于引用父对象,@JsonBackReferences用于标记子对象。
示例 - @JsonManagedReferences
import java.io.IOException; import java.text.ParseException; import java.util.ArrayList; import java.util.List; import com.fasterxml.jackson.annotation.JsonBackReference; import com.fasterxml.jackson.annotation.JsonManagedReference; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException, ParseException { ObjectMapper mapper = new ObjectMapper(); Student student = new Student(1, "Mark"); Book book1 = new Book(1,"Learn HTML", student); Book book2 = new Book(1,"Learn JAVA", student); student.addBook(book1); student.addBook(book2); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(book1); System.out.println(jsonString); } } class Student { public int rollNo; public String name; @JsonBackReference public List<Book> books; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; this.books = new ArrayList<Book>(); } public void addBook(Book book){ books.add(book); } } class Book { public int id; public String name; Book(int id, String name, Student owner){ this.id = id; this.name = name; this.owner = owner; } @JsonManagedReference public Student owner; }
输出
{ "id" : 1, "name" : "Learn HTML", "owner" : { "rollNo" : 1, "name" : "Mark" } }
Jackson注释 - @JsonBackReference
@JsonManagedReferences和JsonBackReferences用于显示具有父子关系的对象。@JsonManagedReferences用于引用父对象,@JsonBackReferences用于标记子对象。
示例 - @JsonBackReferences
import java.io.IOException; import java.text.ParseException; import java.util.ArrayList; import java.util.List; import com.fasterxml.jackson.annotation.JsonBackReference; import com.fasterxml.jackson.annotation.JsonManagedReference; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException, ParseException { ObjectMapper mapper = new ObjectMapper(); Student student = new Student(1, "Mark"); Book book1 = new Book(1,"Learn HTML", student); Book book2 = new Book(1,"Learn JAVA", student); student.addBook(book1); student.addBook(book2); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(book1); System.out.println(jsonString); } } class Student { public int rollNo; public String name; @JsonBackReference public List<Book> books; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; this.books = new ArrayList<Book>(); } public void addBook(Book book){ books.add(book); } } class Book { public int id; public String name; Book(int id, String name, Student owner) { this.id = id; this.name = name; this.owner = owner; } @JsonManagedReference public Student owner; }
输出
{ "id" : 1, "name" : "Learn HTML", "owner" : { "rollNo" : 1, "name" : "Mark" } }
Jackson注释 - @JsonIdentityInfo
当对象具有父子关系时使用@JsonIdentityInfo。@JsonIdentityInfo 用于指示序列化/反序列化期间将使用对象标识。
示例 - @JsonIdentityInfo
import java.io.IOException; import java.text.ParseException; import java.util.ArrayList; import java.util.List; import com.fasterxml.jackson.annotation.JsonIdentityInfo; import com.fasterxml.jackson.annotation.ObjectIdGenerators; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException, ParseException{ ObjectMapper mapper = new ObjectMapper(); Student student = new Student(1,13, "Mark"); Book book1 = new Book(1,"Learn HTML", student); Book book2 = new Book(2,"Learn JAVA", student); student.addBook(book1); student.addBook(book2); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(book1); System.out.println(jsonString); } } @JsonIdentityInfo( generator = ObjectIdGenerators.PropertyGenerator.class, property = "id") class Student { public int id; public int rollNo; public String name; public List<Book> books; Student(int id, int rollNo, String name){ this.id = id; this.rollNo = rollNo; this.name = name; this.books = new ArrayList<Book>(); } public void addBook(Book book){ books.add(book); } } @JsonIdentityInfo( generator = ObjectIdGenerators.PropertyGenerator.class, property = "id") class Book{ public int id; public String name; Book(int id, String name, Student owner){ this.id = id; this.name = name; this.owner = owner; } public Student owner; }
输出
{ "id" : 1, "name" : "Learn HTML", "owner" : { "id" : 1, "rollNo" : 13, "name" : "Mark", "books" : [ 1, { "id" : 2, "name" : "Learn JAVA", "owner" : 1 } ] } }
Jackson注释 - @JsonFilter
@JsonFilter 用于在序列化/反序列化期间应用过滤器,例如要使用或不使用哪些属性。
示例 - @JsonFilter
import java.io.IOException; import java.text.ParseException; import com.fasterxml.jackson.annotation.JsonFilter; import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.databind.ser.FilterProvider; import com.fasterxml.jackson.databind.ser.impl.SimpleBeanPropertyFilter; import com.fasterxml.jackson.databind.ser.impl.SimpleFilterProvider; public class JacksonTester { public static void main(String args[]) throws IOException, ParseException { ObjectMapper mapper = new ObjectMapper(); Student student = new Student(1,13, "Mark"); FilterProvider filters = new SimpleFilterProvider() .addFilter( "nameFilter", SimpleBeanPropertyFilter.filterOutAllExcept("name")); String jsonString = mapper.writer(filters) .withDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } } @JsonFilter("nameFilter") class Student { public int id; public int rollNo; public String name; Student(int id, int rollNo, String name) { this.id = id; this.rollNo = rollNo; this.name = name; } }
输出
{ "name" : "Mark" }
Jackson 注释 - 自定义注释
我们可以使用 @JacksonAnnotationsInside 注释轻松创建自定义注释。
示例 - 自定义注释
import java.io.IOException; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.text.ParseException; import com.fasterxml.jackson.annotation.JacksonAnnotationsInside; import com.fasterxml.jackson.annotation.JsonInclude; import com.fasterxml.jackson.annotation.JsonInclude.Include; import com.fasterxml.jackson.annotation.JsonPropertyOrder; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) throws IOException, ParseException { ObjectMapper mapper = new ObjectMapper(); Student student = new Student(1,13, "Mark"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } } @CustomAnnotation class Student { public int id; public int rollNo; public String name; public String otherDetails; Student(int id, int rollNo, String name){ this.id = id; this.rollNo = rollNo; this.name = name; } } @Retention(RetentionPolicy.RUNTIME) @JacksonAnnotationsInside @JsonInclude(value = Include.NON_NULL) @JsonPropertyOrder({ "rollNo", "id", "name" }) @interface CustomAnnotation {}
输出
{ "rollNo" : 13, "id" : 1, "name" : "Mark" }
Jackson 注释 - Mixin
Mixin注解是一种在不修改目标类的情况下关联注解的方式。请参阅下面的示例 -
示例 - Mixin 注解
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonIgnoreType; import com.fasterxml.jackson.databind.MapperFeature; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]) { ObjectMapper mapper = new ObjectMapper(); try { Student student = new Student(1,11,"1ab","Mark"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); ObjectMapper mapper1 = new ObjectMapper(); mapper1.addMixIn(Name.class, MixInForIgnoreType.class); jsonString = mapper1 .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { public int id; public String systemId; public int rollNo; public Name nameObj; Student(int id, int rollNo, String systemId, String name) { this.id = id; this.systemId = systemId; this.rollNo = rollNo; nameObj = new Name(name); } } class Name { public String name; Name(String name){ this.name = name; } } @JsonIgnoreType class MixInForIgnoreType {}
输出
{ "id" : 1, "systemId" : "1ab", "rollNo" : 11, "nameObj" : { "name" : "Mark" } } { "id" : 1, "systemId" : "1ab", "rollNo" : 11 }
Jackson 注释 - 禁用
我们可以使用ObjectMapper的disable()函数禁用jackson注释。
示例 - 禁用注释
import java.io.IOException; import com.fasterxml.jackson.annotation.JsonIgnoreType; import com.fasterxml.jackson.databind.MapperFeature; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); try{ Student student = new Student(1,11,"1ab","Mark"); String jsonString = mapper .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); ObjectMapper mapper1 = new ObjectMapper(); mapper1.disable(MapperFeature.USE_ANNOTATIONS); jsonString = mapper1 .writerWithDefaultPrettyPrinter() .writeValueAsString(student); System.out.println(jsonString); } catch (IOException e) { e.printStackTrace(); } } } class Student { public int id; public String systemId; public int rollNo; public Name nameObj; Student(int id, int rollNo, String systemId, String name){ this.id = id; this.systemId = systemId; this.rollNo = rollNo; nameObj = new Name(name); } } @JsonIgnoreType class Name { public String name; Name(String name){ this.name = name; } }
输出
{ "id" : 1, "systemId" : "1ab", "rollNo" : 11 } { "id" : 1, "systemId" : "1ab", "rollNo" : 11, "nameObj" : { "name" : "Mark" } }