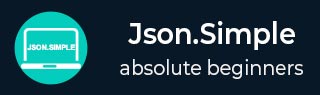
- JSON.simple 教程
- JSON.simple - 主页
- JSON.simple - 概述
- JSON.simple - 环境设置
- JSON.simple - JAVA 映射
- 解码示例
- 转义特殊字符
- JSON.simple - 使用 JSONValue
- JSON.simple - 异常处理
- JSON.simple - 容器工厂
- JSON.simple - 内容处理程序
- 编码示例
- JSON.simple - 编码 JSONObject
- JSON.simple - 编码 JSONArray
- 合并示例
- JSON.simple - 合并对象
- JSON.simple - 合并数组
- 组合示例
- JSON.simple - 原始、对象、数组
- JSON.simple - 原始、映射、列表
- 基元、对象、映射、列表
- 定制示例
- JSON.simple - 自定义输出
- 定制输出流
- JSON.simple 有用资源
- JSON.simple - 快速指南
- JSON.simple - 有用的资源
- JSON.simple - 讨论
JSON.simple - ContentHandler
ContentHandler 接口用于提供类似 SAX 的接口来流式传输大型 json。它还提供可停止的能力。下面的例子说明了这个概念。
例子
import java.io.IOException; import java.util.List; import java.util.Stack; import org.json.simple.JSONArray; import org.json.simple.JSONObject; import org.json.simple.parser.ContentHandler; import org.json.simple.parser.JSONParser; import org.json.simple.parser.ParseException; class JsonDemo { public static void main(String[] args) { JSONParser parser = new JSONParser(); String text = "{\"first\": 123, \"second\": [4, 5, 6], \"third\": 789}"; try { CustomContentHandler handler = new CustomContentHandler(); parser.parse(text, handler,true); } catch(ParseException pe) { } } } class CustomContentHandler implements ContentHandler { @Override public boolean endArray() throws ParseException, IOException { System.out.println("inside endArray"); return true; } @Override public void endJSON() throws ParseException, IOException { System.out.println("inside endJSON"); } @Override public boolean endObject() throws ParseException, IOException { System.out.println("inside endObject"); return true; } @Override public boolean endObjectEntry() throws ParseException, IOException { System.out.println("inside endObjectEntry"); return true; } public boolean primitive(Object value) throws ParseException, IOException { System.out.println("inside primitive: " + value); return true; } @Override public boolean startArray() throws ParseException, IOException { System.out.println("inside startArray"); return true; } @Override public void startJSON() throws ParseException, IOException { System.out.println("inside startJSON"); } @Override public boolean startObject() throws ParseException, IOException { System.out.println("inside startObject"); return true; } @Override public boolean startObjectEntry(String key) throws ParseException, IOException { System.out.println("inside startObjectEntry: " + key); return true; } }
输出
inside startJSON inside startObject inside startObjectEntry: first inside primitive: 123 inside endObjectEntry inside startObjectEntry: second inside startArray inside primitive: 4 inside primitive: 5 inside primitive: 6 inside endArray inside endObjectEntry inside startObjectEntry: third inside primitive: 789 inside endObjectEntry inside endObject inside endJSON