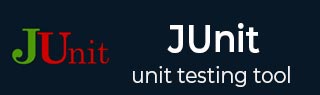
- JUnit 教程
- JUnit - 主页
- JUnit - 概述
- JUnit - 环境设置
- JUnit - 测试框架
- JUnit - 基本用法
- JUnit-API
- JUnit - 编写测试
- JUnit - 使用断言
- JUnit - 执行过程
- JUnit - 执行测试
- JUnit - 套件测试
- JUnit - 忽略测试
- JUnit - 时间测试
- JUnit - 异常测试
- JUnit - 参数化测试
- JUnit - 使用 Ant 插件
- JUnit - 插入 Eclipse
- JUnit - 扩展
- JUnit 有用资源
- JUnit - 问题与解答
- JUnit - 快速指南
- JUnit - 有用的资源
- JUnit - 讨论
JUnit - 编写测试
在这里,我们将看到一个使用 POJO 类、业务逻辑类和测试类进行 JUnit 测试的完整示例,该测试类将由测试运行器运行。
在C:\>JUNIT_WORKSPACE中创建EmployeeDetails.java,这是一个POJO类。
public class EmployeeDetails { private String name; private double monthlySalary; private int age; /** * @return the name */ public String getName() { return name; } /** * @param name the name to set */ public void setName(String name) { this.name = name; } /** * @return the monthlySalary */ public double getMonthlySalary() { return monthlySalary; } /** * @param monthlySalary the monthlySalary to set */ public void setMonthlySalary(double monthlySalary) { this.monthlySalary = monthlySalary; } /** * @return the age */ public int getAge() { return age; } /** * @param age the age to set */ public void setAge(int age) { this.age = age; } }
EmployeeDetails类用于 -
- 获取/设置员工姓名的值。
- 获取/设置员工的月薪值。
- 获取/设置员工年龄的值。
在 C:\>JUNIT_WORKSPACE 中创建一个名为EmpBusinessLogic.java的文件,其中包含业务逻辑。
public class EmpBusinessLogic { // Calculate the yearly salary of employee public double calculateYearlySalary(EmployeeDetails employeeDetails) { double yearlySalary = 0; yearlySalary = employeeDetails.getMonthlySalary() * 12; return yearlySalary; } // Calculate the appraisal amount of employee public double calculateAppraisal(EmployeeDetails employeeDetails) { double appraisal = 0; if(employeeDetails.getMonthlySalary() < 10000){ appraisal = 500; }else{ appraisal = 1000; } return appraisal; } }
EmpBusinessLogic类用于计算 -
- 雇员的年薪。
- 员工的评估金额。
在C:\>JUNIT_WORKSPACE中创建一个名为TestEmployeeDetails.java的文件,其中包含要测试的测试用例。
import org.junit.Test; import static org.junit.Assert.assertEquals; public class TestEmployeeDetails { EmpBusinessLogic empBusinessLogic = new EmpBusinessLogic(); EmployeeDetails employee = new EmployeeDetails(); //test to check appraisal @Test public void testCalculateAppriasal() { employee.setName("Rajeev"); employee.setAge(25); employee.setMonthlySalary(8000); double appraisal = empBusinessLogic.calculateAppraisal(employee); assertEquals(500, appraisal, 0.0); } // test to check yearly salary @Test public void testCalculateYearlySalary() { employee.setName("Rajeev"); employee.setAge(25); employee.setMonthlySalary(8000); double salary = empBusinessLogic.calculateYearlySalary(employee); assertEquals(96000, salary, 0.0); } }
TestEmployeeDetails类用于测试EmpBusinessLogic类的方法。它
- 测试员工的年薪。
- 测试员工的评价金额。
接下来,在 C:\>JUNIT_WORKSPACE 中创建一个名为TestRunner.java的 java 类来执行测试用例。
import org.junit.runner.JUnitCore; import org.junit.runner.Result; import org.junit.runner.notification.Failure; public class TestRunner { public static void main(String[] args) { Result result = JUnitCore.runClasses(TestEmployeeDetails.class); for (Failure failure : result.getFailures()) { System.out.println(failure.toString()); } System.out.println(result.wasSuccessful()); } }
使用 javac 编译测试用例和测试运行器类。
C:\JUNIT_WORKSPACE>javac EmployeeDetails.java EmpBusinessLogic.java TestEmployeeDetails.java TestRunner.java
现在运行测试运行程序,它将运行提供的测试用例类中定义的测试用例。
C:\JUNIT_WORKSPACE>java TestRunner
验证输出。
true