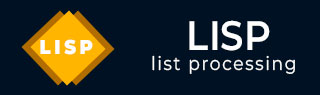
- LISP 教程
- LISP - 主页
- LISP - 概述
- LISP - 环境
- LISP - 程序结构
- LISP - 基本语法
- LISP - 数据类型
- LISP - 宏
- LISP - 变量
- LISP - 常量
- LISP - 运算符
- LISP - 决策
- LISP - 循环
- LISP - 函数
- LISP - 谓词
- LISP - 数字
- LISP - 字符
- LISP - 数组
- LISP - 字符串
- LISP - 序列
- LISP - 列表
- LISP - 符号
- LISP - 向量
- LISP - 设置
- LISP - 树
- LISP - 哈希表
- LISP - 输入和输出
- LISP - 文件 I/O
- LISP - 结构
- LISP - 包
- LISP - 错误处理
- LISP-CLOS
- LISP 有用资源
- Lisp - 快速指南
- Lisp - 有用的资源
- Lisp - 讨论
LISP - 字符串
Common Lisp 中的字符串是向量,即一维字符数组。
字符串文字用双引号括起来。字符集支持的任何字符都可以用双引号括起来以形成字符串,但双引号字符 (") 和转义字符 (\) 除外。但是,您可以通过使用反斜杠 (\) 转义它们来包含这些字符。
例子
创建一个名为 main.lisp 的新源代码文件,并在其中键入以下代码。
(write-line "Hello World") (write-line "Welcome to Tutorials Point") ;escaping the double quote character (write-line "Welcome to \"Tutorials Point\"")
当您执行代码时,它会返回以下结果 -
Hello World Welcome to Tutorials Point Welcome to "Tutorials Point"
字符串比较函数
数字比较函数和运算符(例如 < 和 >)不适用于字符串。Common LISP 提供了另外两组函数来比较代码中的字符串。一组区分大小写,另一组不区分大小写。
下表提供了功能 -
区分大小写的函数 | 不区分大小写的函数 | 描述 |
---|---|---|
字符串= | 字符串等于 | 检查操作数的值是否全部相等,如果相等则条件成立。 |
字符串/= | 字符串不等于 | 检查操作数的值是否全部不同,如果值不相等则条件成立。 |
字符串< | 无字符串 | 检查操作数的值是否单调递减。 |
字符串> | 字符串-greaterp | 检查操作数的值是否单调递增。 |
字符串<= | 字符串非greaterp | 检查任何左操作数的值是否大于或等于下一个右操作数的值,如果是,则条件为真。 |
字符串>= | 字符串非lessp | 检查任何左操作数的值是否小于或等于其右操作数的值,如果是,则条件为真。 |
例子
创建一个名为 main.lisp 的新源代码文件,并在其中键入以下代码。
; case-sensitive comparison (write (string= "this is test" "This is test")) (terpri) (write (string> "this is test" "This is test")) (terpri) (write (string< "this is test" "This is test")) (terpri) ;case-insensitive comparision (write (string-equal "this is test" "This is test")) (terpri) (write (string-greaterp "this is test" "This is test")) (terpri) (write (string-lessp "this is test" "This is test")) (terpri) ;checking non-equal (write (string/= "this is test" "this is Test")) (terpri) (write (string-not-equal "this is test" "This is test")) (terpri) (write (string/= "lisp" "lisping")) (terpri) (write (string/= "decent" "decency"))
当您执行代码时,它会返回以下结果 -
NIL 0 NIL T NIL NIL 8 NIL 4 5
案例控制函数
下表描述了案例控制功能 -
先生。 | 功能说明 |
---|---|
1 | 字符串大写 将字符串转换为大写 |
2 | 字符串小写 将字符串转换为小写 |
3 | 字符串大写 将字符串中的每个单词大写 |
例子
创建一个名为 main.lisp 的新源代码文件,并在其中键入以下代码。
(write-line (string-upcase "a big hello from tutorials point")) (write-line (string-capitalize "a big hello from tutorials point"))
当您执行代码时,它会返回以下结果 -
A BIG HELLO FROM TUTORIALS POINT A Big Hello From Tutorials Point
修剪琴弦
下表描述了字符串修剪功能 -
先生。 | 功能说明 |
---|---|
1 | 弦修剪 它采用一个字符串作为第一个参数,一个字符串作为第二个参数,并返回一个子字符串,其中第一个参数中的所有字符都从参数字符串中删除。 |
2 | 弦左修剪 它采用一个字符串作为第一个参数,一个字符串作为第二个参数,并返回一个子字符串,其中第一个参数中的所有字符都从参数字符串的开头删除。 |
3 | 弦右修剪 它接受一个字符串字符作为第一个参数,一个字符串作为第二个参数,并返回一个子字符串,其中第一个参数中的所有字符都从参数字符串的末尾删除。 |
例子
创建一个名为 main.lisp 的新源代码文件,并在其中键入以下代码。
(write-line (string-trim " " " a big hello from tutorials point ")) (write-line (string-left-trim " " " a big hello from tutorials point ")) (write-line (string-right-trim " " " a big hello from tutorials point ")) (write-line (string-trim " a" " a big hello from tutorials point "))
当您执行代码时,它会返回以下结果 -
a big hello from tutorials point a big hello from tutorials point a big hello from tutorials point big hello from tutorials point
其他字符串函数
LISP 中的字符串是数组,因此也是序列。我们将在接下来的教程中介绍这些数据类型。所有适用于数组和序列的函数也适用于字符串。但是,我们将使用各种示例来演示一些常用的功能。
计算长度
length函数计算字符串的长度。
提取子串
subseq函数返回从特定索引开始并继续到特定结束索引或字符串末尾的子字符串(因为字符串也是序列)。
访问字符串中的字符
char函数允许访问字符串的各个字符。
例子
创建一个名为 main.lisp 的新源代码文件,并在其中键入以下代码。
(write (length "Hello World")) (terpri) (write-line (subseq "Hello World" 6)) (write (char "Hello World" 6))
当您执行代码时,它会返回以下结果 -
11 World #\W
字符串的排序和合并
sort函数允许对字符串进行排序。它接受一个序列(向量或字符串)和一个双参数谓词,并返回序列的排序版本。
merge函数接受两个序列和一个谓词,并根据谓词返回通过合并两个序列而产生的序列。
例子
创建一个名为 main.lisp 的新源代码文件,并在其中键入以下代码。
;sorting the strings (write (sort (vector "Amal" "Akbar" "Anthony") #'string<)) (terpri) ;merging the strings (write (merge 'vector (vector "Rishi" "Zara" "Priyanka") (vector "Anju" "Anuj" "Avni") #'string<))
当您执行代码时,它会返回以下结果 -
#("Akbar" "Amal" "Anthony") #("Anju" "Anuj" "Avni" "Rishi" "Zara" "Priyanka")
反转字符串
反向函数反转字符串。
例如,创建一个名为 main.lisp 的新源代码文件,并在其中键入以下代码。
(write-line (reverse "Are we not drawn onward, we few, drawn onward to new era"))
当您执行代码时,它会返回以下结果 -
are wen ot drawno nward ,wef ew ,drawno nward ton ew erA
连接字符串
concatenate 函数连接两个字符串。这是通用序列函数,您必须提供结果类型作为第一个参数。
例如,创建一个名为 main.lisp 的新源代码文件,并在其中键入以下代码。
(write-line (concatenate 'string "Are we not drawn onward, " "we few, drawn onward to new era"))
当您执行代码时,它会返回以下结果 -
Are we not drawn onward, we few, drawn onward to new era