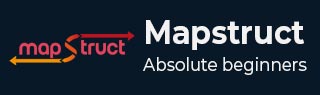
- 地图结构教程
- 地图结构 - 主页
- MapStruct - 概述
- MapStruct - 环境设置
- 测绘
- MapStruct - 基本映射
- MapStruct - 自定义映射
- MapStruct - 映射多个
- MapStruct - 映射嵌套 Bean
- MapStruct - 映射直接字段
- MapStruct - 生成器
- 数据类型转换
- MapStruct - 隐式类型转换
- MapStruct - 使用 numberFormat
- MapStruct - 使用日期格式
- MapStruct - 使用表达式
- MapStruct - 使用常量
- MapStruct - 使用defaultValue
- MapStruct - 使用defaultExpression
- 映射集合
- MapStruct - 映射列表
- MapStruct - 映射地图
- 各种各样的
- MapStruct - 映射流
- MapStruct - 映射枚举
- MapStruct - 抛出异常
- MapStruct - 自定义映射器
- MapStruct 有用资源
- MapStruct - 快速指南
- MapStruct - 有用的资源
- MapStruct - 讨论
MapStruct - 使用自定义映射器
Mapstruct 映射器允许创建自定义映射器方法来映射对象。对于mapper接口,我们可以添加一个默认方法。
句法
@Mapper(uses=DateMapper.class) public interface UtilityMapper { default Car getCar(CarEntity entity) { Car car = new Car(); car.setId(entity.getId()); car.setName(entity.getName()); return car; } }
以下示例演示了相同的内容。
例子
打开 Eclipse 中的映射枚举章节中更新的项目映射。
使用以下代码更新 UtilityMapper.java -
实用程序映射器.java
package com.tutorialspoint.mapper; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; import java.util.GregorianCalendar; import java.util.Map; import java.util.stream.Stream; import org.mapstruct.MapMapping; import org.mapstruct.Mapper; import org.mapstruct.ValueMapping; import com.tutorialspoint.entity.CarEntity; import com.tutorialspoint.enums.OrderType; import com.tutorialspoint.enums.PlacedOrderType; import com.tutorialspoint.model.Car; @Mapper(uses=DateMapper.class) public interface UtilityMapper { @MapMapping(valueDateFormat = "dd.MM.yyyy") Map<String, String> getMap(Map<Long, GregorianCalendar> source); Stream<String> getStream(Stream<Integer> source); @ValueMapping(source = "EXTRA", target = "SPECIAL") PlacedOrderType getEnum(OrderType order); CarEntity getCarEntity(Car car) throws ParseException; default Car getCar(CarEntity entity) { Car car = new Car(); car.setId(entity.getId()); car.setName(entity.getName()); return car; } } class DateMapper { public String asString(GregorianCalendar date) { return date != null ? new SimpleDateFormat( "yyyy-MM-dd" ) .format( date.getTime() ) : null; } public GregorianCalendar asDate(String date) throws ParseException { Date date1 = date != null ? new SimpleDateFormat( "yyyy-MM-dd" ) .parse( date ) : null; if(date1 != null) { return new GregorianCalendar(date1.getYear(), date1.getMonth(),date1.getDay()); } return null; } }
使用以下代码更新 UtilityMapperTest.java -
UtilityMapperTest.java
package com.tutorialspoint.mapping; import static org.junit.jupiter.api.Assertions.assertEquals; import static org.junit.jupiter.api.Assertions.assertTrue; import java.text.ParseException; import java.util.Arrays; import java.util.GregorianCalendar; import java.util.HashMap; import java.util.Map; import java.util.stream.Stream; import org.junit.jupiter.api.Test; import org.mapstruct.factory.Mappers; import com.tutorialspoint.entity.CarEntity; import com.tutorialspoint.enums.OrderType; import com.tutorialspoint.enums.PlacedOrderType; import com.tutorialspoint.mapper.UtilityMapper; import com.tutorialspoint.model.Car; public class UtilityMapperTest { private UtilityMapper utilityMapper = Mappers.getMapper(UtilityMapper.class); @Test public void testMapMapping() { Map<Long, GregorianCalendar> source = new HashMap<>(); source.put(1L, new GregorianCalendar(2015, 3, 5)); Map<String, String> target = utilityMapper.getMap(source); assertEquals("2015-04-05", target.get("1")); } @Test public void testGetStream() { Stream<Integer> numbers = Arrays.asList(1, 2, 3, 4).stream(); Stream<String> strings = utilityMapper.getStream(numbers); assertEquals(4, strings.count()); } @Test public void testGetEnum() { PlacedOrderType placedOrderType = utilityMapper.getEnum(OrderType.EXTRA); PlacedOrderType placedOrderType1 = utilityMapper.getEnum(OrderType.NORMAL); PlacedOrderType placedOrderType2 = utilityMapper.getEnum(OrderType.STANDARD); assertEquals(PlacedOrderType.SPECIAL.name(), placedOrderType.name()); assertEquals(PlacedOrderType.NORMAL.name(), placedOrderType1.name()); assertEquals(PlacedOrderType.STANDARD.name(), placedOrderType2.name()); } @Test public void testGetCarEntity() { Car car = new Car(); car.setId(1); car.setManufacturingDate("11/10/2020"); boolean exceptionOccured = false; try { CarEntity carEntity = utilityMapper.getCarEntity(car); } catch (ParseException e) { exceptionOccured = true; } assertTrue(exceptionOccured); } @Test public void testGetCar() { CarEntity entity = new CarEntity(); entity.setId(1); entity.setName("ZEN"); Car car = utilityMapper.getCar(entity); assertEquals(entity.getId(), car.getId()); assertEquals(entity.getName(), car.getName()); } }
运行以下命令来测试映射。
mvn clean test
输出
一旦命令成功。验证输出。
mvn clean test [INFO] Scanning for projects... ... [INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ mapping --- [INFO] Surefire report directory: \mvn\mapping\target\surefire-reports ------------------------------------------------------- T E S T S ------------------------------------------------------- Running com.tutorialspoint.mapping.CarMapperTest Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.256 sec Running com.tutorialspoint.mapping.DeliveryAddressMapperTest Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.001 sec Running com.tutorialspoint.mapping.StudentMapperTest Tests run: 2, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0 sec Running com.tutorialspoint.mapping.UtilityMapperTest Tests run: 5, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0 sec Results : Tests run: 9, Failures: 0, Errors: 0, Skipped: 0 ...