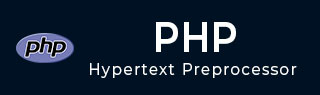
- PHP教程
- PHP-主页
- PHP - 简介
- PHP-环境设置
- PHP - 语法概述
- PHP - 变量类型
- PHP - 常量
- PHP - 运算符类型
- PHP - 决策
- PHP - 循环类型
- PHP-数组
- PHP-字符串
- PHP - 网络概念
- PHP - 获取和发布
- PHP - 文件包含
- PHP - 文件和 I/O
- PHP - 函数
- PHP-Cookie
- PHP-会话
- PHP - 发送电子邮件
- PHP-文件上传
- PHP - 编码标准
- 高级PHP
- PHP - 预定义变量
- PHP-正则表达式
- PHP - 错误处理
- PHP - 错误调试
- PHP - 日期和时间
- PHP 和 MySQL
- PHP 和 AJAX
- PHP 和 XML
- PHP——面向对象
- PHP - 面向 C 开发人员
- PHP - 适合 PERL 开发人员
- PHP 表单示例
- PHP-表单介绍
- PHP - 验证示例
- PHP - 完整表格
- PHP框架作品
- PHP-框架工程
- PHP - 核心 PHP 与 Frame Works
- PHP 设计模式
- PHP - 设计模式
- PHP 函数参考
- PHP - 内置函数
- PHP 有用资源
- PHP - 问题与解答
- PHP - 有用的资源
- PHP - 讨论
PHP - SAX 解析器示例
SAX 解析器用于解析 xml 文件,并且比示例 xml 解析器和 DOM 更适合内存管理。它不会在内存中保存任何数据,因此可用于非常大的文件。以下示例将展示如何使用 SAX API 从 xml 获取数据。
SAX.xml
XML 应如下所示 -
<?xml version = "1.0" encoding = "utf-8"?> <tutors> <course> <name>Android</name> <country>India</country> <email>contact@tutorialspoint.com</email> <phone>123456789</phone> </course> <course> <name>Java</name> <country>India</country> <email>contact@tutorialspoint.com</email> <phone>123456789</phone> </course> <course> <name>HTML</name> <country>India</country> <email>contact@tutorialspoint.com</email> <phone>123456789</phone> </course> </tutors>
SAX.php
PHP 文件应如下 -
<?php //Reading XML using the SAX(Simple API for XML) parser $tutors = array(); $elements = null; // Called to this function when tags are opened function startElements($parser, $name, $attrs) { global $tutors, $elements; if(!empty($name)) { if ($name == 'COURSE') { // creating an array to store information $tutors []= array(); } $elements = $name; } } // Called to this function when tags are closed function endElements($parser, $name) { global $elements; if(!empty($name)) { $elements = null; } } // Called on the text between the start and end of the tags function characterData($parser, $data) { global $tutors, $elements; if(!empty($data)) { if ($elements == 'NAME' || $elements == 'COUNTRY' || $elements == 'EMAIL' || $elements == 'PHONE') { $tutors[count($tutors)-1][$elements] = trim($data); } } } // Creates a new XML parser and returns a resource handle referencing it to be used by the other XML functions. $parser = xml_parser_create(); xml_set_element_handler($parser, "startElements", "endElements"); xml_set_character_data_handler($parser, "characterData"); // open xml file if (!($handle = fopen('sax.xml', "r"))) { die("could not open XML input"); } while($data = fread($handle, 4096)) // read xml file { xml_parse($parser, $data); // start parsing an xml document } xml_parser_free($parser); // deletes the parser $i = 1; foreach($tutors as $course) { echo "course No - ".$i.'<br/>'; echo "course Name - ".$course['NAME'].'<br/>'; echo "Country - ".$course['COUNTRY'].'<br/>'; echo "Email - ".$course['EMAIL'].'<br/>'; echo "Phone - ".$course['PHONE'].'<hr/>'; $i++; } ?>
它将产生以下结果 -
