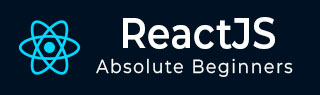
- ReactJS 教程
- ReactJS - 主页
- ReactJS - 简介
- ReactJS - 安装
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS-JSX
- ReactJS - 组件
- ReactJS - 样式
- ReactJS - 属性(props)
- ReactJS - 事件管理
- ReactJS - 状态管理
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 路由
- ReactJS-Redux
- ReactJS - 动画
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- ReactJS 有用的资源
- ReactJS - 快速指南
- ReactJS - 有用的资源
- ReactJS - 讨论
ReactJS - 测试
测试是确保任何应用程序中创建的功能按照业务逻辑和编码规范运行的过程之一。React 建议使用React 测试库来测试 React 组件,并使用 jest测试运行器来运行测试。React -testing-library允许单独检查组件。
可以使用以下命令将其安装在应用程序中 -
npm install --save @testing-library/react @testing-library/jest-dom
创建反应应用程序
创建 React 应用程序默认配置React 测试库和jest测试运行器。因此,测试使用Create React App创建的 React 应用程序只需一条命令。
cd /go/to/react/application npm test
npm test命令与npm build命令类似。当开发人员更改代码时,两者都会重新编译。在命令提示符中执行该命令后,它会发出以下问题。
No tests found related to files changed since last commit. Press `a` to run all tests, or run Jest with `--watchAll`. Watch Usage › Press a to run all tests. › Press f to run only failed tests. › Press q to quit watch mode. › Press p to filter by a filename regex pattern. › Press t to filter by a test name regex pattern. › Press Enter to trigger a test run.
按 a 将尝试运行所有测试脚本,最后总结结果如下所示 -
Test Suites: 1 passed, 1 total Tests: 1 passed, 1 total Snapshots: 0 total Time: 4.312 s, estimated 12 s Ran all test suites. Watch Usage: Press w to show more.
在自定义应用程序中进行测试
让我们使用Rollup 捆绑器编写一个自定义 React 应用程序,并使用本章中的React 测试库和jest测试运行器对其进行测试。
首先,按照创建React 应用程序章节中的说明,使用Rollup捆绑器创建一个新的React 应用程序, react-test-app。
接下来,安装测试库。
cd /go/to/react-test-app npm install --save @testing-library/react @testing-library/jest-dom
接下来,在您喜欢的编辑器中打开应用程序。
接下来,在src/components文件夹下创建一个文件HelloWorld.test.js来为HelloWorld组件编写测试并开始编辑。
接下来,导入react库。
import React from 'react';
接下来,导入测试库。
import { render, screen } from '@testing-library/react'; import '@testing-library/jest-dom';
接下来,导入我们的HelloWorld组件。
import HelloWorld from './HelloWorld';
接下来,编写一个测试来检查文档中是否存在Hello World 文本。
test('test scenario 1', () => { render(<HelloWorld />); const element = screen.getByText(/Hello World/i); expect(element).toBeInTheDocument(); });
测试代码的完整源代码如下:
import React from 'react'; import { render, screen } from '@testing-library/react'; import '@testing-library/jest-dom'; import HelloWorld from './HelloWorld'; test('test scenario 1', () => { render(<HelloWorld />); const element = screen.getByText(/Hello World/i); expect(element).toBeInTheDocument(); });
接下来,安装 jest 测试运行程序(如果系统中尚未安装)。
npm install jest -g
接下来,在应用程序的根文件夹中运行 jest 命令。
jest
接下来,在应用程序的根文件夹中运行 jest 命令。
PASS src/components/HelloWorld.test.js √ test scenario 1 (29 ms) Test Suites: 1 passed, 1 total Tests: 1 passed, 1 total Snapshots: 0 total Time: 5.148 s Ran all test suites.