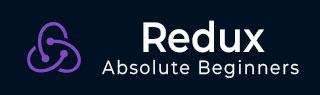
- Redux Tutorial
- Redux - Home
- Redux - Overview
- Redux - Installation
- Redux - Core Concepts
- Redux - Data Flow
- Redux - Store
- Redux - Actions
- Redux - Pure Functions
- Redux - Reducers
- Redux - Middleware
- Redux - Devtools
- Redux - Testing
- Redux - Integrate React
- Redux - React Example
- Redux Useful Resources
- Redux - Quick Guide
- Redux - Useful Resources
- Redux - Discussion
Redux - 测试
测试 Redux 代码很容易,因为我们主要编写函数,而且大多数都是纯函数。所以我们可以测试它,甚至不用嘲笑它们。在这里,我们使用 JEST 作为测试引擎。它工作在node环境下,不访问DOM。
我们可以使用下面给出的代码安装 JEST -
npm install --save-dev jest
使用 babel,您需要安装babel-jest,如下所示 -
npm install --save-dev babel-jest
并将其配置为使用 .babelrc 文件中的 babel-preset-env 功能,如下所示 -
{ "presets": ["@babel/preset-env"] } And add the following script in your package.json: { //Some other code "scripts": { //code "test": "jest", "test:watch": "npm test -- --watch" }, //code }
最后,运行 npm test 或 npm run test。让我们检查一下如何为操作创建者和缩减者编写测试用例。
动作创建者的测试用例
让我们假设您有动作创建者,如下所示 -
export function itemsRequestSuccess(bool) { return { type: ITEMS_REQUEST_SUCCESS, isLoading: bool, } }
这个动作创建者可以进行测试,如下所示 -
import * as action from '../actions/actions'; import * as types from '../../constants/ActionTypes'; describe('actions', () => { it('should create an action to check if item is loading', () => { const isLoading = true, const expectedAction = { type: types.ITEMS_REQUEST_SUCCESS, isLoading } expect(actions.itemsRequestSuccess(isLoading)).toEqual(expectedAction) }) })
减速器测试用例
我们已经了解到,当应用操作时,Reducer 应该返回一个新的状态。因此,reducer 对此Behave进行了测试。
考虑一个减速器,如下所示 -
const initialState = { isLoading: false }; const reducer = (state = initialState, action) => { switch (action.type) { case 'ITEMS_REQUEST': return Object.assign({}, state, { isLoading: action.payload.isLoading }) default: return state; } } export default reducer;
为了测试上述减速器,我们需要将状态和操作传递给减速器,并返回一个新状态,如下所示 -
import reducer from '../../reducer/reducer' import * as types from '../../constants/ActionTypes' describe('reducer initial state', () => { it('should return the initial state', () => { expect(reducer(undefined, {})).toEqual([ { isLoading: false, } ]) }) it('should handle ITEMS_REQUEST', () => { expect( reducer( { isLoading: false, }, { type: types.ITEMS_REQUEST, payload: { isLoading: true } } ) ).toEqual({ isLoading: true }) }) })
如果您不熟悉测试用例的编写,可以查看JEST的基础知识。