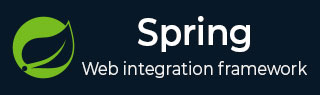
- Spring 核心基础知识
- 春天 - 主页
- 春天 - 概述
- 春天-建筑
- Spring - 环境设置
- Spring - Hello World 示例
- Spring - IoC 容器
- Spring - Bean 定义
- Spring - Bean 范围
- Spring - Bean 生命周期
- Spring - Bean 后处理器
- Spring-Bean定义继承
- Spring - 依赖注入
- Spring - 注入内部 Bean
- Spring - 注入集合
- Spring - Bean 自动装配
- 基于注释的配置
- Spring - 基于Java的配置
- Spring - Spring 中的事件处理
- Spring - Spring 中的自定义事件
- Spring - 使用 Spring 框架的 AOP
- Spring - JDBC 框架
- Spring-事务管理
- Spring - Web MVC 框架
- Spring - 使用 Log4J 进行日志记录
- 春季问答
- 春天 - 问题与解答
- 春季有用资源
- 春天 - 快速指南
- Spring - 有用的资源
- 春天 - 讨论
Spring - 基于Java的配置
到目前为止,您已经了解了如何使用 XML 配置文件配置 Spring bean。如果您熟悉 XML 配置,那么实际上不需要学习如何继续进行基于 Java 的配置,因为您将使用任何可用的配置来实现相同的结果。
基于 Java 的配置选项使您能够在不使用 XML 的情况下编写大部分 Spring 配置,但需要借助本章中介绍的一些基于 Java 的注释。
@Configuration & @Bean 注解
使用@Configuration注释一个类表明该类可以被Spring IoC容器用作bean定义的源。@Bean注释告诉Spring,用@Bean注释的方法将返回一个应该在Spring应用程序上下文中注册为bean的对象。最简单的 @Configuration 类如下 -
package com.tutorialspoint; import org.springframework.context.annotation.*; @Configuration public class HelloWorldConfig { @Bean public HelloWorld helloWorld(){ return new HelloWorld(); } }
上面的代码相当于下面的 XML 配置 -
<beans> <bean id = "helloWorld" class = "com.tutorialspoint.HelloWorld" /> </beans>
这里,方法名称用 @Bean 注释,作为 bean ID,它创建并返回实际的 bean。您的配置类可以有多个 @Bean 的声明。定义配置类后,您可以使用AnnotationConfigApplicationContext加载它们并将其提供给 Spring 容器,如下所示 -
public static void main(String[] args) { ApplicationContext ctx = new AnnotationConfigApplicationContext(HelloWorldConfig.class); HelloWorld helloWorld = ctx.getBean(HelloWorld.class); helloWorld.setMessage("Hello World!"); helloWorld.getMessage(); }
您可以加载各种配置类,如下所示 -
public static void main(String[] args) { AnnotationConfigApplicationContext ctx = new AnnotationConfigApplicationContext(); ctx.register(AppConfig.class, OtherConfig.class); ctx.register(AdditionalConfig.class); ctx.refresh(); MyService myService = ctx.getBean(MyService.class); myService.doStuff(); }
例子
让我们准备好一个可用的 Eclipse IDE,并按照以下步骤创建一个 Spring 应用程序 -
脚步 | 描述 |
---|---|
1 | 创建一个名为SpringExample的项目,并在创建的项目中的src文件夹下创建一个包com.tutorialspoint 。 |
2 | 使用“添加外部 JAR”选项添加所需的 Spring 库,如Spring Hello World 示例一章中所述。 |
3 | 因为您使用的是基于 Java 的注释,所以您还需要添加Java 安装目录中的CGLIB.jar和ASM.jar库,该库可以从asm.ow2.org下载。 |
4 | 在com.tutorialspoint包下创建 Java 类HelloWorldConfig、HelloWorld和MainApp。 |
5 | 最后一步是创建所有 Java 文件和 Bean 配置文件的内容并运行应用程序,如下所述。 |
这是HelloWorldConfig.java文件的内容
package com.tutorialspoint; import org.springframework.context.annotation.*; @Configuration public class HelloWorldConfig { @Bean public HelloWorld helloWorld(){ return new HelloWorld(); } }
这是HelloWorld.java文件的内容
package com.tutorialspoint; public class HelloWorld { private String message; public void setMessage(String message){ this.message = message; } public void getMessage(){ System.out.println("Your Message : " + message); } }
以下是MainApp.java文件的内容
package com.tutorialspoint; import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.*; public class MainApp { public static void main(String[] args) { ApplicationContext ctx = new AnnotationConfigApplicationContext(HelloWorldConfig.class); HelloWorld helloWorld = ctx.getBean(HelloWorld.class); helloWorld.setMessage("Hello World!"); helloWorld.getMessage(); } }
创建完所有源文件并添加所需的附加库后,让我们运行该应用程序。您应该注意,不需要配置文件。如果您的应用程序一切正常,它将打印以下消息 -
Your Message : Hello World!
注入 Bean 依赖项
当 @Beans 相互依赖时,表达依赖关系就像让一个 bean 方法调用另一个 bean 方法一样简单,如下所示 -
package com.tutorialspoint; import org.springframework.context.annotation.*; @Configuration public class AppConfig { @Bean public Foo foo() { return new Foo(bar()); } @Bean public Bar bar() { return new Bar(); } }
在这里,foo bean 通过构造函数注入接收对 bar 的引用。现在让我们看另一个工作示例。
例子
让我们准备好一个可用的 Eclipse IDE,并按照以下步骤创建一个 Spring 应用程序 -
脚步 | 描述 |
---|---|
1 | 创建一个名为SpringExample的项目,并在创建的项目中的src文件夹下创建一个包com.tutorialspoint 。 |
2 | 使用“添加外部 JAR”选项添加所需的 Spring 库,如Spring Hello World 示例一章中所述。 |
3 | 因为您使用的是基于 Java 的注释,所以您还需要添加Java 安装目录中的CGLIB.jar和ASM.jar库,该库可以从asm.ow2.org下载。 |
4 | 在com.tutorialspoint包下创建 Java 类TextEditorConfig、TextEditor、SpellChecker和MainApp。 |
5 | 最后一步是创建所有 Java 文件和 Bean 配置文件的内容并运行应用程序,如下所述。 |
这是TextEditorConfig.java文件的内容
package com.tutorialspoint; import org.springframework.context.annotation.*; @Configuration public class TextEditorConfig { @Bean public TextEditor textEditor(){ return new TextEditor( spellChecker() ); } @Bean public SpellChecker spellChecker(){ return new SpellChecker( ); } }
这是TextEditor.java文件的内容
package com.tutorialspoint; public class TextEditor { private SpellChecker spellChecker; public TextEditor(SpellChecker spellChecker){ System.out.println("Inside TextEditor constructor." ); this.spellChecker = spellChecker; } public void spellCheck(){ spellChecker.checkSpelling(); } }
以下是另一个依赖类文件SpellChecker.java的内容
package com.tutorialspoint; public class SpellChecker { public SpellChecker(){ System.out.println("Inside SpellChecker constructor." ); } public void checkSpelling(){ System.out.println("Inside checkSpelling." ); } }
以下是MainApp.java文件的内容
package com.tutorialspoint; import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.*; public class MainApp { public static void main(String[] args) { ApplicationContext ctx = new AnnotationConfigApplicationContext(TextEditorConfig.class); TextEditor te = ctx.getBean(TextEditor.class); te.spellCheck(); } }
创建完所有源文件并添加所需的附加库后,让我们运行该应用程序。您应该注意,不需要配置文件。如果您的应用程序一切正常,它将打印以下消息 -
Inside SpellChecker constructor. Inside TextEditor constructor. Inside checkSpelling.
@Import 注解
@Import注释允许从另一个配置类加载 @Bean 定义。考虑 ConfigA 类如下 -
@Configuration public class ConfigA { @Bean public A a() { return new A(); } }
您可以将上面的 Bean 声明导入到另一个 Bean 声明中,如下所示 -
@Configuration @Import(ConfigA.class) public class ConfigB { @Bean public B b() { return new B(); } }
现在,在实例化上下文时不需要同时指定 ConfigA.class 和 ConfigB.class,只需提供 ConfigB,如下所示 -
public static void main(String[] args) { ApplicationContext ctx = new AnnotationConfigApplicationContext(ConfigB.class); // now both beans A and B will be available... A a = ctx.getBean(A.class); B b = ctx.getBean(B.class); }
生命周期回调
@Bean 注释支持指定任意初始化和销毁回调方法,很像 Spring XML 的 bean 元素上的 init-method 和 destroy-method 属性 -
public class Foo { public void init() { // initialization logic } public void cleanup() { // destruction logic } } @Configuration public class AppConfig { @Bean(initMethod = "init", destroyMethod = "cleanup" ) public Foo foo() { return new Foo(); } }
指定 Bean 范围
默认范围是单例,但您可以使用 @Scope 注释覆盖它,如下所示 -
@Configuration public class AppConfig { @Bean @Scope("prototype") public Foo foo() { return new Foo(); } }