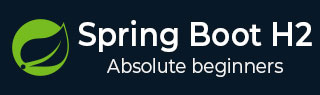
- Spring Boot 和 H2 教程
- Spring Boot 和 H2 - 主页
- Spring Boot 和 H2 - 概述
- Spring Boot & H2 - 环境设置
- Spring Boot 和 H2 - 项目设置
- Spring Boot 和 H2 - REST API
- Spring Boot 和 H2 - H2 控制台
- Spring Boot 和 H2 示例
- Spring Boot & H2 - 添加记录
- Spring Boot & H2 - 获取记录
- Spring Boot & H2 - 获取所有记录
- Spring Boot & H2 - 更新记录
- Spring Boot & H2 - 删除记录
- Spring Boot & H2 - 单元测试控制器
- Spring Boot & H2 - 单元测试服务
- Spring Boot 和 H2 - 单元测试存储库
- Spring Boot 和 H2 有用资源
- Spring Boot 和 H2 - 快速指南
- Spring Boot 和 H2 - 有用的资源
- Spring Boot 和 H2 - 讨论
Spring Boot 和 H2 - 项目设置
与上一章环境设置一样,我们在 eclipse 中导入了生成的 spring boot 项目。现在让我们在src/main/java文件夹中创建以下结构。

com.tutorialspoint.controller.EmployeeController - 基于 REST 的控制器,用于实现基于 REST 的 API。
com.tutorialspoint.entity.Employee - 表示数据库中相应表的实体类。
com.tutorialspoint.repository.EmployeeRepository - 用于在数据库上实现 CRUD 操作的存储库接口。
com.tutorialspoint.service.EmployeeService - 通过存储库功能实现业务操作的服务类。
com.tutorialspoint.springbooth2.SprintBootH2Application - Spring Boot 应用程序类。
SprintBootH2Application 类已经存在。我们需要创建上述包以及相关的类和接口,如下所示 -
实体-Entity.java
以下是 Employee 的默认代码。它代表一个包含 id、name、age 和 email 列的 Employee 表。
package com.tutorialspoint.entity; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table public class Employee { @Id @Column private int id; @Column private String name; @Column private int age; @Column private String email; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
存储库-EmployeeRepository.java
以下是 Repository 对上述实体 Employee 实现 CRUD 操作的默认代码。
package com.tutorialspoint.repository; import org.springframework.data.repository.CrudRepository; import org.springframework.stereotype.Repository; import com.tutorialspoint.entity.Employee; @Repository public interface EmployeeRepository extends CrudRepository<Employee, Integer> { }
服务-EmployeeService.java
以下是Service默认实现对repository函数操作的代码。
package com.tutorialspoint.service; import java.util.ArrayList; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import com.tutorialspoint.entity.Employee; import com.tutorialspoint.repository.EmployeeRepository; @Service public class EmployeeService { @Autowired EmployeeRepository repository; public Employee getEmployeeById(int id) { return null; } public List<Employee> getAllEmployees(){ return null; } public void saveOrUpdate(Employee employee) { } public void deleteEmployeeById(int id) { } }
控制器 - EmployeeController.java
以下是Controller实现REST API的默认代码。
package com.tutorialspoint.controller; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.DeleteMapping; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.PutMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import com.tutorialspoint.entity.Employee; import com.tutorialspoint.service.EmployeeService; @RestController @RequestMapping(path = "/emp") public class EmployeeController { @Autowired EmployeeService employeeService; @GetMapping("/employees") public List<Employee> getAllEmployees(){ return null; } @GetMapping("/employee/{id}") public Employee getEmployee(@PathVariable("id") int id) { return null;; } @DeleteMapping("/employee/{id}") public void deleteEmployee(@PathVariable("id") int id) { } @PostMapping("/employee") public void addEmployee(@RequestBody Employee employee) { } @PutMapping("/employee") public void updateEmployee(@RequestBody Employee employee) { } }
应用程序 - SprintBootH2Application.java
以下是应用程序使用上述类的更新代码。
package com.tutorialspoint.sprintbooth2; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.autoconfigure.domain.EntityScan; import org.springframework.context.annotation.ComponentScan; import org.springframework.data.jpa.repository.config.EnableJpaRepositories; @ComponentScan({"com.tutorialspoint.controller","com.tutorialspoint.service"}) @EntityScan("com.tutorialspoint.entity") @EnableJpaRepositories("com.tutorialspoint.repository") @SpringBootApplication public class SprintBootH2Application { public static void main(String[] args) { SpringApplication.run(SprintBootH2Application.class, args); } }
运行/调试配置
在 Eclipse 中创建以下Maven 配置,以运行目标为spring-boot:run 的springboot 应用程序。此配置将有助于运行 REST API,我们可以使用 POSTMAN 测试它们。
