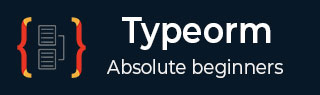
- TypeORM教程
- TypeORM - 主页
- TypeORM - 简介
- TypeORM - 安装
- TypeORM - 创建一个简单的项目
- TypeORM - 连接 API
- TypeORM - 实体
- TypeORM - 关系
- TypeORM - 使用存储库
- TypeORM - 使用实体管理器
- TypeORM - 查询生成器
- TypeORM - 查询操作
- TypeORM - 交易
- TypeORM - 索引
- TypeORM - 实体监听器和日志记录
- 使用 JavaScript 进行 TypeORM
- TypeORM - 使用 MongoDB
- 使用 Express 进行 TypeORM
- TypeORM - 迁移
- TypeORM - 使用 CLI
- TypeORM 有用资源
- TypeORM - 快速指南
- TypeORM - 有用的资源
- TypeORM - 讨论
TypeORM - 使用 MongoDB
本章解释了 TypeORM 提供的广泛的 MongoDB 数据库支持。希望我们已经使用 npm 安装了 mongodb。如果未安装,请使用以下命令安装 MongoDB 驱动程序,
npm install mongodb --save
创建项目
让我们使用 MongoDB 创建一个新项目,如下所示 -
typeorm init --name MyProject --database mongodb
配置ormconfig.json
让我们在 ormconfig.json 文件中配置 MongoDB 主机、端口和数据库选项,如下所示 -
ormconfig.json
{ "type": "mongodb", "host": "localhost", "port": 27017, "database": "test", "synchronize": true, "logging": false, "entities": [ "src/entity/**/*.ts" ], "migrations": [ "src/migration/**/*.ts" ], "subscribers": [ "src/subscriber/**/*.ts" ], "cli": { "entitiesDir": "src/entity", "migrationsDir": "src/migration", "subscribersDir": "src/subscriber" } }
定义实体和列
让我们在 src 目录中创建一个名为 Student 的新实体。实体和列是相同的。要生成主键列,我们使用@PrimaryColumn或
@PrimaryGenerateColumn。这可以定义为@ObjectIdColumn。简单的例子如下所示 -
学生.ts
import {Entity, ObjectID, ObjectIdColumn, Column} from "typeorm"; @Entity() export class Student { @ObjectIdColumn() id: ObjectID; @Column() Name: string; @Column() Country: string; }
要保存此实体,请打开 index.ts 文件并添加以下更改 -
索引.ts
import "reflect-metadata"; import {createConnection} from "typeorm"; import {Student} from "./entity/Student"; createConnection().then(async connection => { console.log("Inserting a new Student into the database..."); const std = new Student(); std.Name = "Student1"; std.Country = "India"; await connection.manager.save(std); console.log("Saved a new user with id: " + std.id); console.log("Loading users from the database..."); const stds = await connection.manager.find(Student); console.log("Loaded users: ", stds); console.log("TypeORM with MongoDB"); }).catch(error => console.log(error));
现在,启动您的服务器,您将得到以下响应 -
npm start

MongoDB 实体管理器
我们还可以使用EntityManager来获取数据。简单的例子如下所示 -
import {getManager} from "typeorm"; const manager = getManager(); const result = await manager.findOne(Student, { id:1 });
同样,我们也可以使用存储库来访问数据。
import {getMongoRepository} from "typeorm"; const studentRepository = getMongoRepository(Student); const result = await studentRepository.findOne({ id:1 });
如果您想使用 equal 选项过滤数据,如下所示 -
import {getMongoRepository} from "typeorm"; const studentRepository = getMongoRepository(Student); const result = await studentRepository.find({ where: { Name: {$eq: "Student1"}, } });
正如我们在本章中看到的,TypeORM 使得使用 MongoDB 数据库引擎变得很容易。