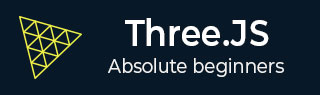
- 三.js教程
- Three.js - 主页
- Three.js - 简介
- Three.js - 安装
- Three.js - Hello Cube 应用程序
- Three.js - 渲染器和响应能力
- Three.js - 响应式设计
- Three.js - 调试和统计
- Three.js - 相机
- Three.js - 控件
- Three.js - 光与影
- Three.js - 几何
- Three.js - 材料
- Three.js - 纹理
- Three.js - 画线
- Three.js - 动画
- Three.js - 创建文本
- Three.js - 加载 3D 模型
- Three.js - 库和插件
- Three.js 有用资源
- Three.js - 快速指南
- Three.js - 有用的资源
- Three.js - 讨论
Three.js - 调试和统计
使用Dat.GUI
不断尝试变量的值(例如立方体的位置)是很困难的。在这种情况下,假设直到你得到你喜欢的东西。这是一个缓慢而艰巨的过程。幸运的是,已经有一个很好的解决方案可以与 Three.js、dat.GUI 完美集成。它允许您创建一个可以更改代码中变量的基本用户界面组件。
安装
要在您的项目中使用 dat.GUI,请在此处下载并将 <script> 标记添加到 HTML 文件中。
<script type='text/javascript' src='path/to/dat.gui.min.js'></script>
或者您可以使用 CDN,在 HTML 中添加以下 <script> 标记。
<script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.7/dat.gui.js"></script>
如果您在节点应用程序中使用 Three.js,请安装 npm 包 - dat.GUI 并将其导入您的 JavaScript 文件中。
npm install dat.gui
或者
yarn add dat.gui import * as dat from 'dat.gui'
用法
首先,您应该初始化对象本身。它创建一个小部件并将其显示在屏幕右上角。
const gui = new dat.GUI()
然后,您可以添加要控制的参数和变量。例如,下面的代码是控制立方体的y位置。
gui.add(cube.position, 'y')
例子
尝试添加其他位置变量。请参阅此工作代码示例。
立方体.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Three.js - Position GUI</title> <style> * { margin: 0; padding: 0; box-sizing: border-box; font-family: -applesystem, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif; } html, body { height: 100vh; width: 100vw; } #threejs-container { position: block; width: 100%; height: 100%; } </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.7/dat.gui.js"></script> </head> <body> <div id="threejs-container"></div> <script type="module"> // Adding UI to debug and experimenting different values // UI const gui = new dat.GUI() // sizes let width = window.innerWidth let height = window.innerHeight // scene const scene = new THREE.Scene() scene.background = new THREE.Color(0x262626) // camera const camera = new THREE.PerspectiveCamera(45, width / height, 0.1, 100) camera.position.set(0, 0, 10) // cube const geometry = new THREE.BoxGeometry(2, 2, 2) const material = new THREE.MeshBasicMaterial({ color: 0xffffff, wireframe: true }) gui.add(material, 'wireframe') const cube = new THREE.Mesh(geometry, material) scene.add(cube) gui.add(cube.position, 'x') gui.add(cube.position, 'y') gui.add(cube.position, 'z') // responsiveness window.addEventListener('resize', () => { width = window.innerWidth height = window.innerHeight camera.aspect = width / height camera.updateProjectionMatrix() renderer.setSize(window.innerWidth, window.innerHeight) renderer.render(scene, camera) }) // renderer const renderer = new THREE.WebGL1Renderer() renderer.setSize(width, height) renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2)) // animation function animate() { requestAnimationFrame(animate) cube.rotation.x += 0.005 cube.rotation.y += 0.01 renderer.render(scene, camera) } // rendering the scene const container = document.querySelector('#threejs-container') container.append(renderer.domElement) renderer.render(scene, camera) animate() </script> </body> </html>
输出
您可以使用 name 属性自定义显示的标签。要更改变量行上的标签,请使用 .name("your label")。
gui.add(cube.position, 'y').name('cube-y')
您可以设置最小/最大限制以及获取滑块的步骤。以下行允许值从 1 到 10,每次将值增加 1。
gui.add(cube.position, 'y').min(1).max(10).step(1) // or gui.add(cube.position, 'y', 1, 10, 1)
如果有许多同名的变量,您可能会发现很难区分它们。在这种情况下,您可以为每个对象添加文件夹。与对象相关的所有变量都位于一个文件夹中。
// creating a folder const cube1 = gui.addFolder('Cube 1') cube1.add(redCube.position, 'y').min(1).max(10).step(1) cube1.add(redCube.position, 'x').min(1).max(10).step(1) cube1.add(redCube.position, 'z').min(1).max(10).step(1) // another folder const cube2 = gui.addFolder('Cube 2') cube2.add(greenCube.position, 'y').min(1).max(10).step(1) cube2.add(greenCube.position, 'x').min(1).max(10).step(1) cube2.add(greenCube.position, 'z').min(1).max(10).step(1)
例子
现在,检查以下示例。
gui-folders.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Three.js - More variables</title> <style> * { margin: 0; padding: 0; box-sizing: border-box; font-family: -applesystem, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif; } html, body { height: 100vh; width: 100vw; } #threejs-container { position: block; width: 100%; height: 100%; } </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.7/dat.gui.js"></script> </head> <body> <div id="threejs-container"></div> <script type="module"> // Adding folders to distinguish between variables // controls const gui = new dat.GUI() // sizes let width = window.innerWidth let height = window.innerHeight // scene const scene = new THREE.Scene() scene.background = new THREE.Color(0x262626) // camera const camera = new THREE.PerspectiveCamera(45, width / height, 0.1, 100) camera.position.set(0, 0, 10) const camFolder = gui.addFolder('Camera') camFolder.add(camera.position, 'z').min(10).max(60).step(10) // cube const geometry = new THREE.BoxGeometry(2, 2, 2) const material = new THREE.MeshBasicMaterial({ color: 0xffffff, wireframe: true }) const cubeColor = { color: 0xffffff } const materialFolder = gui.addFolder('Material') materialFolder.add(material, 'wireframe') materialFolder.addColor(cubeColor, 'color').onChange(() => { // callback material.color.set(cubeColor.color) }) materialFolder.open() const cube = new THREE.Mesh(geometry, material) scene.add(cube) const cubeFolder = gui.addFolder('Cube') // for position const posFolder = cubeFolder.addFolder('position') posFolder.add(cube.position, 'x', 0, 5, 0.1) posFolder.add(cube.position, 'y', 0, 5, 0.1) posFolder.add(cube.position, 'z', 0, 5, 0.1) posFolder.open() // for scale const scaleFolder = cubeFolder.addFolder('Scale') scaleFolder.add(cube.scale, 'x', 0, 5, 0.1).name('Width') scaleFolder.add(cube.scale, 'y', 0, 5, 0.1).name('Height') scaleFolder.add(cube.scale, 'z', 0, 5, 0.1).name('Depth') scaleFolder.open() cubeFolder.open() // responsiveness window.addEventListener('resize', () => { width = window.innerWidth height = window.innerHeight camera.aspect = width / height camera.updateProjectionMatrix() renderer.setSize(window.innerWidth, window.innerHeight) renderer.render(scene, camera) }) // renderer const renderer = new THREE.WebGL1Renderer() renderer.setSize(width, height) renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2)) // animation function animate() { requestAnimationFrame(animate) cube.rotation.x += 0.005 cube.rotation.y += 0.01 renderer.render(scene, camera) } // rendering the scene const container = document.querySelector('#threejs-container') container.append(renderer.domElement) renderer.render(scene, camera) animate() </script> </body> </html>
输出
您还可以添加一些回调函数。一旦值改变就会触发onChange。
gui.add(cube.position, 'y').onChange(function () { // refresh based on the new value of y console.log(cube.position.y) })
让我们看一下使用 dat.gui 和回调更改颜色的另一个示例。
// parameter const cubeColor = { color: 0xff0000, } gui.addColor(cubeColor, 'color').onChange(() => { // callback cube.color.set(cubeColor.color) })
当cubeColor的颜色改变时,上面的回调onChange通知Three.js改变立方体的颜色。
从现在开始我们将经常使用这个 dat.gui。通过尝试“Hello Cube!”确保您习惯了它。应用程序。
统计- 统计在大规模应用中发挥着重要作用。