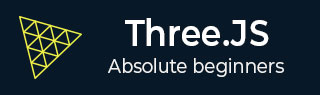
- 三.js教程
- Three.js - 主页
- Three.js - 简介
- Three.js - 安装
- Three.js - Hello Cube 应用程序
- Three.js - 渲染器和响应能力
- Three.js - 响应式设计
- Three.js - 调试和统计
- Three.js - 相机
- Three.js - 控件
- Three.js - 光与影
- Three.js - 几何
- Three.js - 材料
- Three.js - 纹理
- Three.js - 画线
- Three.js - 动画
- Three.js - 创建文本
- Three.js - 加载 3D 模型
- Three.js - 库和插件
- Three.js 有用资源
- Three.js - 快速指南
- Three.js - 有用的资源
- Three.js - 讨论
Three.js - 画线
你已经在 Three.js 中学到了相当多的资料。现在让我们看看一些用于绘制线条的独特材料。我们可以用线条画出各种形状和图案。
使用BufferGeometry
THREE.BufferGeometry 是 Three.js 中所有内置几何图形的基类。您可以通过传递几何图形的顶点数组来创建几何图形。
在此处了解有关 BufferGeometry 的更多信息。
const points = [] points.push(new THREE.Vector3(-10, 0, 0)) points.push(new THREE.Vector3(0, -10, 0)) points.push(new THREE.Vector3(10, 0, 0))
这些是 Three.js 为我们提供的一些附加元素,用于创建几何图形。THREE.Vector3(x, y, z) - 它在 3D 空间中生成一个点。在上面的代码中,我们向点数组添加 3 个点。
const geometry = new THREE.BufferGeometry().setFromPoints(points)
THREE.BufferGeometry(),如前所述,它创建了我们的几何图形。我们使用 setFromPoints 方法通过点数组设置几何图形。
注意- 在每对连续的顶点之间绘制线条,但不在第一个和最后一个顶点之间绘制线条(线条不是闭合的。)
const material = new THREE.LineBasicMaterial({ // for normal lines color: 0xffffff, linewidth: 1, linecap: 'round', //ignored by WebGLRenderer linejoin: 'round', //ignored by WebGLRenderer }) // or const material = new THREE.LineDashedMaterial({ // for dashed lines color: 0xffffff, linewidth: 1,scale: 1, dashSize: 3, gapSize: 1, })
这些是线条的独特材料。您可以使用 THREE.LineBasicMaterial 或 THREE.LineDashedMaterial 中的任何一种。
const line = new THREE.Line(geometry, material)
例子
现在,我们不再使用 THREE.Mesh,而是使用 THREE.Line 来绘制线条。现在,您会在屏幕上看到使用线条绘制的“V”形状。
linebasic.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Three.js - Line basic</title> <style> * { margin: 0; padding: 0; box-sizing: border-box; font-family: -applesystem, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif; } html, body { height: 100vh; width: 100vw; } #threejs-container { position: block; width: 100%; height: 100%; } </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.7/dat.gui.js"></script> </head> <body> <div id="threejs-container"></div> <script type="module"> // Creating a line using LineBasicMaterial // GUI const gui = new dat.GUI() // sizes let width = window.innerWidth let height = window.innerHeight // scene const scene = new THREE.Scene() scene.background = new THREE.Color(0x262626) // camera const camera = new THREE.PerspectiveCamera(45, width / height, 0.1, 100) camera.position.set(0, 0, 50) camera.lookAt(0, 0, 0) const camFolder = gui.addFolder('Camera') camFolder.add(camera.position, 'z', 10, 100) camFolder.open() // Line const points = [] points.push(new THREE.Vector3(-10, 0, 0)) points.push(new THREE.Vector3(0, -20, 0)) points.push(new THREE.Vector3(10, 0, 0)) const folders = [gui.addFolder('Poin 1'), gui.addFolder('Poin 2'), gui.addFolder('Poin 3')] folders.forEach((folder, i) => { folder.add(points[i], 'x', -30, 30, 1).onChange(redraw) folder.add(points[i], 'y', -30, 30, 1).onChange(redraw) folder.add(points[i], 'z', -30, 30, 1).onChange(redraw) folder.open() }) const geometry = new THREE.BufferGeometry().setFromPoints(points) const material = new THREE.LineBasicMaterial({ color: 0xffffff, linewidth: 2 }) const line = new THREE.Line(geometry, material) line.position.set(0, 10, 0) scene.add(line) function redraw() { let newGeometry = new THREE.BufferGeometry().setFromPoints(points) line.geometry.dispose() line.geometry = newGeometry } // responsiveness window.addEventListener('resize', () => { width = window.innerWidth height = window.innerHeight camera.aspect = width / height camera.updateProjectionMatrix() renderer.setSize(window.innerWidth, window.innerHeight) renderer.render(scene, camera) }) // renderer const renderer = new THREE.WebGL1Renderer() renderer.setSize(width, height) renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2)) // animation function animate() { requestAnimationFrame(animate) renderer.render(scene, camera) } // rendering the scene const container = document.querySelector('#threejs-container') container.append(renderer.domElement) renderer.render(scene, camera) animate() </script> </body> </html>
输出
例子
您可以通过指定顶点使用线创建任何类型的几何线框。查看下面我们绘制虚线的示例。
虚线.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Three.js - Dashed line</title> <style> * { margin: 0; padding: 0; box-sizing: border-box; font-family: -applesystem, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif; } html, body { height: 100vh; width: 100vw; } #threejs-container { position: block; width: 100%; height: 100%; } </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.7/dat.gui.js"></script> </head> <body> <div id="threejs-container"></div> <script type="module"> // Creating dashed line using LineDashedMaterial // GUI const gui = new dat.GUI() // sizes let width = window.innerWidth let height = window.innerHeight // scene const scene = new THREE.Scene() scene.background = new THREE.Color(0x262626) // camera const camera = new THREE.PerspectiveCamera(45, width / height, 0.1, 100) camera.position.set(0, 0, 50) camera.lookAt(0, 0, 0) const camFolder = gui.addFolder('Camera') camFolder.add(camera.position, 'z', 10, 100) camFolder.open() // Line const points = [] points.push(new THREE.Vector3(-10, 0, 0)) points.push(new THREE.Vector3(0, -20, 0)) points.push(new THREE.Vector3(10, 0, 0)) const folders = [gui.addFolder('Poin 1'), gui.addFolder('Poin 2'), gui.addFolder('Poin 3')] folders.forEach((folder, i) => { folder.add(points[i], 'x', -30, 30, 1).onChange(redraw) folder.add(points[i], 'y', -30, 30, 1).onChange(redraw) folder.add(points[i], 'z', -30, 30, 1).onChange(redraw) folder.open() }) const geometry = new THREE.BufferGeometry().setFromPoints(points) const material = new THREE.LineDashedMaterial({ color: 0xffffff, linewidth: 2, scale: 1, dashSize: 3, gapSize: 2 }) const line = new THREE.Line(geometry, material) line.computeLineDistances() line.position.set(0, 10, 0) scene.add(line) console.log(line) function redraw() { let newGeometry = new THREE.BufferGeometry().setFromPoints(points) line.geometry.dispose() line.geometry = newGeometry } // responsiveness window.addEventListener('resize', () => { width = window.innerWidth height = window.innerHeight camera.aspect = width / height camera.updateProjectionMatrix() renderer.setSize(window.innerWidth, window.innerHeight) renderer.render(scene, camera) }) // renderer const renderer = new THREE.WebGL1Renderer() renderer.setSize(width, height) renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2)) // animation function animate() { requestAnimationFrame(animate) renderer.render(scene, camera) } // rendering the scene const container = document.querySelector('#threejs-container') container.append(renderer.domElement) renderer.render(scene, camera) animate() </script> </body> </html>