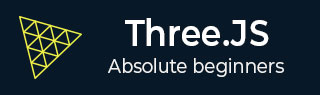
- 三.js教程
- Three.js - 主页
- Three.js - 简介
- Three.js - 安装
- Three.js - Hello Cube 应用程序
- Three.js - 渲染器和响应能力
- Three.js - 响应式设计
- Three.js - 调试和统计
- Three.js - 相机
- Three.js - 控件
- Three.js - 光与影
- Three.js - 几何
- Three.js - 材料
- Three.js - 纹理
- Three.js - 画线
- Three.js - 动画
- Three.js - 创建文本
- Three.js - 加载 3D 模型
- Three.js - 库和插件
- Three.js 有用资源
- Three.js - 快速指南
- Three.js - 有用的资源
- Three.js - 讨论
Three.js - 响应式设计
在调整屏幕大小时,您可以观察到场景没有响应。使网页响应通常是指页面在不同尺寸的显示器(从台式机、平板电脑到手机)上显示良好。在本章中,您可以了解如何解决 Three.js 应用程序的一些基本问题。
当浏览器尺寸改变时自动调整输出大小
当您调整浏览器大小时,我们必须通知 Three.js 以了解 <canvas> 元素应该有多宽。对于相机,我们需要更新纵横比属性,该属性保存屏幕的纵横比,对于渲染器,我们需要更改其大小。
window.addEventListener('resize', () => { // update display width and height width = window.innerWidth height = window.innerHeight // update camera aspect camera.aspect = width / height camera.updateProjectionMatrix() // update renderer renderer.setSize(width, height) renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2)) renderer.render(scene, camera) })
例子
上面的代码为您的 Three.js 项目提供了响应能力。
调整浏览器大小.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Three.js – Resizing browser</title> <style> * { margin: 0; padding: 0; box-sizing: border-box; font-family: -applesystem, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif; } html, body { height: 100vh; width: 100vw; } #threejs-container { position: block; width: 100%; height: 100%; } </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.7/dat.gui.js"></script> </head> <body> <div id="threejs-container"></div> <script type="module"> // Adding responsiveness for Three.js // sizes let width = window.innerWidth let height = window.innerHeight const gui = new dat.GUI() // scene const scene = new THREE.Scene() scene.background = new THREE.Color(0x262626) // camera const camera = new THREE.PerspectiveCamera(45, width / height, 0.1, 100) camera.position.set(0, 0, 10) // cube const geometry = new THREE.BoxGeometry(2, 2, 2) const material = new THREE.MeshBasicMaterial({ color: 0xffffff, wireframe: true }) const cube = new THREE.Mesh(geometry, material) scene.add(cube) // responsiveness window.addEventListener('resize', () => { width = window.innerWidth height = window.innerHeight camera.aspect = width / height camera.updateProjectionMatrix() renderer.setSize(window.innerWidth, window.innerHeight) renderer.render(scene, camera) }) // renderer const renderer = new THREE.WebGL1Renderer() renderer.setSize(width, height) renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2)) // animation function animate() { requestAnimationFrame(animate) cube.rotation.x += 0.005 cube.rotation.y += 0.01 renderer.render(scene, camera) } // rendering the scene const container = document.querySelector('#threejs-container') container.append(renderer.domElement) renderer.render(scene, camera) animate() </script> </body> </html>
输出
当您执行代码时,它将产生以下输出 -
现在,调整浏览器的大小。由于响应式设计,该对象始终会重新定位在浏览器的中心。
抗锯齿
锯齿效应是指边缘和对象(使用像素渲染)上出现锯齿状边缘或“锯齿”(也称为阶梯线)。

例子
抗锯齿.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Three.js - Anti-aliasing</title> <style> * { margin: 0; padding: 0; box-sizing: border-box; font-family: -applesystem, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif; } html, body { height: 100vh; width: 100vw; } #threejs-container { position: block; width: 100%; height: 100%; } </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.7/dat.gui.js"></script> </head> <body> <div id="threejs-container"></div> <script type="module"> // Adding anti-aliasing to Three.js app for removing jaggies // sizes let width = window.innerWidth let height = window.innerHeight const gui = new dat.GUI() // scene const scene = new THREE.Scene() scene.background = new THREE.Color(0x262626) // camera const camera = new THREE.PerspectiveCamera(45, width / height, 0.1, 100) camera.position.set(0, 0, 10) // cube const geometry = new THREE.BoxGeometry(2, 2, 2) const material = new THREE.MeshBasicMaterial({ color: 0xffffff, wireframe: true }) const cube = new THREE.Mesh(geometry, material) scene.add(cube) // responsiveness window.addEventListener('resize', () => { width = window.innerWidth height = window.innerHeight camera.aspect = width / height camera.updateProjectionMatrix() renderer.setSize(window.innerWidth, window.innerHeight) renderer.render(scene, camera) }) // renderer - anti-aliasing const renderer = new THREE.WebGLRenderer({ antialias: true }) renderer.physicallyCorrectLights = true renderer.setSize(width, height) renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2)) // animation function animate() { requestAnimationFrame(animate) cube.rotation.x += 0.005 cube.rotation.y += 0.01 renderer.render(scene, camera) } // rendering the scene const container = document.querySelector('#threejs-container') container.append(renderer.domElement) renderer.render(scene, camera) animate() </script> </body> </html>
输出
我们的 Hello 立方体应用程序中的别名如下所示。

我们可以通过将 WebGLRenderer 的 antialias 属性设置为 true 来打开抗锯齿功能。默认情况下,它是错误的。在这里,我们将抗锯齿参数设置为 true -
const renderer = new WebGLRenderer({ antialias: true }) renderer.physicallyCorrectLights = true
抗锯齿后,它看起来很平滑,没有锯齿,如下图所示。

physicalCorrectLights 属性告诉 Three.js 是否使用物理上正确的光照模式。默认为 false。将其设置为 true 有助于增加对象的细节。