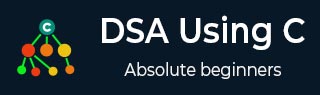
- DSA 使用 C 教程
- 使用 C 的 DSA - 主页
- 使用 C 语言的 DSA - 概述
- 使用 C 语言的 DSA - 环境
- 使用 C 算法的 DSA
- 使用 C 的 DSA - 概念
- 使用 C 数组的 DSA
- 使用 C 链表的 DSA
- 使用 C 的 DSA - 双向链表
- 使用 C 的 DSA - 循环链表
- 使用 C 的 DSA - 堆栈内存溢出
- 使用 C 的 DSA - 解析表达式
- 使用 C 队列的 DSA
- 使用 C 的 DSA - 优先级队列
- 使用 C 树的 DSA
- 使用 C 哈希表的 DSA
- 使用 C 堆的 DSA
- 使用 C - Graph 的 DSA
- 使用 C 搜索技术的 DSA
- 使用 C 排序技术的 DSA
- 使用 C 的 DSA - 递归
- 使用 C 语言的 DSA 有用资源
- 使用 C 的 DSA - 快速指南
- 使用 C 的 DSA - 有用资源
- 使用 C 的 DSA - 讨论
使用 C 选择排序的 DSA
概述
选择排序是一种简单的排序算法。该排序算法是一种基于就地比较的算法,其中列表分为两部分,已排序部分位于左端,未排序部分位于右端。最初排序的部分是空的,未排序的部分是整个列表。
从未排序的数组中选择最小的元素并与最左边的元素交换,该元素成为排序数组的一部分。此过程继续将未排序的数组边界向右移动一个元素。
该算法不适合大型数据集,因为其平均和最坏情况复杂度为 O(n 2 ),其中 n 为否。的项目。
伪代码
Selection Sort ( A: array of item) procedure selectionSort( A : array of items ) int indexMin for i = 1 to length(A) - 1 inclusive do: /* set current element as minimum*/ indexMin = i /* check the element to be minimum */ for j = i+1 to length(A) - 1 inclusive do: if(intArray[j] < intArray[indexMin]){ indexMin = j; } end for /* swap the minimum element with the current element*/ if(indexMin != i) then swap(A[indexMin],A[i]) end if end for end procedure
例子
#include <stdio.h> #include <stdbool.h> #define MAX 7 int intArray[MAX] = {4,6,3,2,1,9,7}; void printline(int count){ int i; for(i=0;i <count-1;i++){ printf("="); } printf("=\n"); } void display(){ int i; printf("["); // navigate through all items for(i=0;i<MAX;i++){ printf("%d ",intArray[i]); } printf("]\n"); } void selectionSort(){ int indexMin,i,j; // loop through all numbers for(i=0; i < MAX-1; i++){ // set current element as minimum indexMin = i; // check the element to be minimum for(j=i+1;j<MAX;j++){ if(intArray[j] < intArray[indexMin]){ indexMin = j; } } if(indexMin != i){ printf("Items swapped: [ %d, %d ]\n" ,intArray[i],intArray[indexMin]); // swap the numbers int temp=intArray[indexMin]; intArray[indexMin] = intArray[i]; intArray[i] = temp; } printf("Iteration %d#:",(i+1)); display(); } } main(){ printf("Input Array: "); display(); printline(50); selectionSort(); printf("Output Array: "); display(); printline(50); }
输出
如果我们编译并运行上面的程序,那么它将产生以下输出 -
Input Array: [4, 6, 3, 2, 1, 9, 7] ================================================== Items swapped: [ 4, 1 ] iteration 1#: [1, 6, 3, 2, 4, 9, 7] Items swapped: [ 6, 2 ] iteration 2#: [1, 2, 3, 6, 4, 9, 7] iteration 3#: [1, 2, 3, 6, 4, 9, 7] Items swapped: [ 6, 4 ] iteration 4#: [1, 2, 3, 4, 6, 9, 7] iteration 5#: [1, 2, 3, 4, 6, 9, 7] Items swapped: [ 9, 7 ] iteration 6#: [1, 2, 3, 4, 6, 7, 9] Output Array: [1, 2, 3, 4, 6, 7, 9] ==================================================
dsa_using_c_sorting_techniques.htm