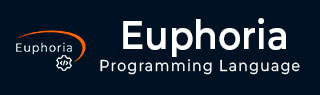
- Euphoria Tutorial
- Euphoria - Home
- Euphoria - Overview
- Euphoria - Environment
- Euphoria - Basic Syntax
- Euphoria - Variables
- Euphoria - Constants
- Euphoria - Data Types
- Euphoria - Operators
- Euphoria - Branching
- Euphoria - Loop Types
- Euphoria - Flow Control
- Euphoria - Short Circuit
- Euphoria - Sequences
- Euphoria - Date & Time
- Euphoria - Procedures
- Euphoria - Functions
- Euphoria - Files I/O
- Euphoria Useful Resources
- Euphoria - Quick Guide
- Euphoria - Library Routines
- Euphoria - Useful Resources
- Euphoria - Discussion
Euphoria - while 语句
while 循环是一种控制结构,允许您重复任务一定次数。
句法
while 循环的语法如下 -
while expression do -- Statements executed if expression returns true end while
执行时,如果表达式结果为 true,则执行循环内的操作。只要表达式结果为真,这种情况就会持续下去。
while循环的关键点是,循环可能永远不会运行。当测试表达式且结果为 false 时,将跳过循环体并执行 while 循环后的第一条语句。
例子
#!/home/euphoria-4.0b2/bin/eui integer a = 10 while a < 20 do printf(1, "value of a : %d\n", a) a = a + 1 end while
这会产生以下结果 -
value of a : 10 value of a : 11 value of a : 12 value of a : 13 value of a : 14 value of a : 15 value of a : 16 value of a : 17 value of a : 18 value of a : 19
while ....带有入口语句
通常情况下,循环的第一次迭代有些特殊。在循环开始之前必须完成一些事情。它们在开始循环的语句之前完成。
with条目语句很好地达到了目的。您需要将此语句与 while 循环一起使用,并在您希望第一次迭代开始的位置添加Entry关键字。
句法
带条目的 while 循环的语法如下 -
while expression with entry do -- Statements executed if expression returns true entry -- Initialisation statements. end while
在执行表达式之前,它会执行初始化语句,然后作为普通的 while 循环启动。随后,这些初始化语句成为循环体的一部分。
例子
#!/home/euphoria-4.0b2/bin/eui integer a = 10 while a < 20 with entry do printf(1, "value of a : %d\n", a) a = a + 1 entry a = a + 2 end while
这会产生以下结果 -
value of a : 12 value of a : 15 value of a : 18
while ....标签声明
while循环可以在第一个do关键字之前有一个标签子句。您可以在 Enter子句之前或之后保留标签子句。
while 循环标签仅用于命名循环块,标签名称必须是具有单个或多个单词的双引号常量字符串。label 关键字区分大小写,应写为label。
句法
带标签子句的 while 循环的语法如下 -
while expression label "Label Name" do -- Statements executed if expression returns true end while
当您使用嵌套 while 循环时,标签非常有用。您可以使用带有标签名称的continue或exit循环控制语句来控制循环流程。
例子
#!/home/euphoria-4.0b2/bin/eui integer a = 10 integer b = 20 while a < 20 label "OUTER" do printf(1, "value of a : %d\n", a) a = a + 1 while b < 30 label "INNER" do printf(1, "value of b : %d\n", b) b = b + 1 if b > 25 then continue "OUTER" -- go to start of OUTER loop end if end while end while
这会产生以下结果 -
value of a : 10 value of b : 20 value of b : 21 value of b : 22 value of b : 23 value of b : 24 value of b : 25 value of a : 11 value of b : 26 value of a : 12 value of b : 27 value of a : 13 value of b : 28 value of a : 14 value of b : 29 value of a : 15 value of a : 16 value of a : 17 value of a : 18 value of a : 19
euphoria_loop_types.htm