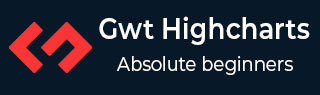
- GWT Highcharts 教程
- GWT Highcharts - 主页
- GWT Highcharts - 概述
- 环境设置
- 配置语法
- GWT Highcharts - 折线图
- GWT Highcharts - 面积图
- GWT Highcharts - 条形图
- GWT Highcharts - 柱形图
- GWT Highcharts - 饼图
- GWT Highcharts - 散点图
- GWT Highcharts - 动态图表
- GWT Highcharts - 组合
- GWT Highcharts - 3D 图表
- GWT Highcharts - 地图图表
- GWT Highcharts 有用资源
- GWT Highcharts - 快速指南
- GWT Highcharts - 有用的资源
- GWT Highcharts - 讨论
GWT Highcharts - 配置语法
在本章中,我们将展示使用 GWT 中的 Highcharts API 绘制图表所需的配置。
第 1 步:创建 GWT 应用程序
按照以下步骤更新我们在GWT - 创建应用程序章节中创建的 GWT 应用程序 -
步 | 描述 |
---|---|
1 | 按照GWT - 创建应用程序章节中的说明,在com.tutorialspoint包下创建一个名为HelloWorld的项目。 |
2 | 如下所述修改HelloWorld.gwt.xml、HelloWorld.html和HelloWorld.java 。保持其余文件不变。 |
3 | 编译并运行应用程序以验证实现逻辑的结果。 |
以下是修改后的模块描述符src/com.tutorialspoint/HelloWorld.gwt.xml的内容。
<?xml version = "1.0" encoding = "UTF-8"?> <module rename-to = 'helloworld'> <inherits name = 'com.google.gwt.user.User'/> <inherits name = 'com.google.gwt.user.theme.clean.Clean'/> <entry-point class = 'com.tutorialspoint.client.HelloWorld'/> <inherits name="org.moxieapps.gwt.highcharts.Highcharts"/> <source path = 'client'/> <source path = 'shared'/> </module>
以下是修改后的 HTML 主机文件war/HelloWorld.html的内容。
<html> <head> <title>GWT Highcharts Showcase</title> <link rel = "stylesheet" href = "HelloWorld.css"/> <script language = "javascript" src = "helloworld/helloworld.nocache.js"> <script src = "https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js" /> <script src = "https://code.highcharts.com/highcharts.js" /> </script> </head> <body> </body> </html>
了解配置后,我们最终会看到更新后的HelloWorld.java。
第 2 步:创建配置
创建图表
配置图表的类型、标题和副标题。
Chart chart = new Chart() .setType(Type.SPLINE) .setChartTitleText("Monthly Average Temperature") .setChartSubtitleText("Source: WorldClimate.com");
x轴
配置要在 X 轴上显示的股票代码。
XAxis xAxis = chart.getXAxis(); xAxis.setCategories("Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec");
y轴
配置要在 Y 轴上显示的标题、绘图线。
YAxis yAxis = chart.getYAxis(); yAxis.setAxisTitleText("Temperature °C"); yAxis.createPlotLine() .setValue(0) .setWidth(1) .setColor("#808080");
工具提示
配置工具提示。将后缀添加到值(y 轴)之后。
ToolTip toolTip = new ToolTip(); toolTip.setValueSuffix("°C"); chart.setToolTip(toolTip);
传奇
配置图例与其他属性一起显示在图表的右侧。
legend.setLayout(Legend.Layout.VERTICAL) .setAlign(Legend.Align.RIGHT) .setVerticalAlign(Legend.VerticalAlign.TOP) .setX(-10) .setY(100) .setBorderWidth(0); chart.setLegend(legend);
系列
配置要在图表上显示的数据。Series 是一个数组,其中该数组的每个元素代表图表上的一条线。
chart.addSeries(chart.createSeries() .setName("Tokyo") .setPoints(new Number[] { 7.0, 6.9, 9.5, 14.5, 18.2, 21.5, 25.2, 26.5, 23.3, 18.3, 13.9, 9.6 }) ); chart.addSeries(chart.createSeries() .setName("New York") .setPoints(new Number[] { -0.2, 0.8, 5.7, 11.3, 17.0, 22.0, 24.8, 24.1, 20.1, 14.1, 8.6, 2.5 }) ); chart.addSeries(chart.createSeries() .setName("Berlin") .setPoints(new Number[] { -0.9, 0.6, 3.5, 8.4, 13.5, 17.0, 18.6, 17.9, 14.3, 9.0, 3.9, 1.0 }) ); chart.addSeries(chart.createSeries() .setName("London") .setPoints(new Number[] { 3.9, 4.2, 5.7, 8.5, 11.9, 15.2, 17.0, 16.6, 14.2, 10.3, 6.6, 4.8 }) );
步骤 3:将图表添加到父面板。
我们将图表添加到根面板。
RootPanel.get().add(chart);
例子
考虑以下示例以进一步理解配置语法 -
HelloWorld.java
package com.tutorialspoint.client; import org.moxieapps.gwt.highcharts.client.Chart; import org.moxieapps.gwt.highcharts.client.Legend; import org.moxieapps.gwt.highcharts.client.Series.Type; import org.moxieapps.gwt.highcharts.client.ToolTip; import org.moxieapps.gwt.highcharts.client.XAxis; import org.moxieapps.gwt.highcharts.client.YAxis; import com.google.gwt.core.client.EntryPoint; import com.google.gwt.user.client.ui.RootPanel; public class HelloWorld implements EntryPoint { public void onModuleLoad() { Chart chart = new Chart() .setType(Type.SPLINE) .setChartTitleText("Monthly Average Temperature") .setChartSubtitleText("Source: WorldClimate.com"); XAxis xAxis = chart.getXAxis(); xAxis.setCategories("Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"); YAxis yAxis = chart.getYAxis(); yAxis.setAxisTitleText("Temperature °C"); yAxis.createPlotLine() .setValue(0) .setWidth(1) .setColor("#808080"); ToolTip toolTip = new ToolTip(); toolTip.setValueSuffix("°C"); chart.setToolTip(toolTip); Legend legend = new Legend(); legend.setLayout(Legend.Layout.VERTICAL) .setAlign(Legend.Align.RIGHT) .setVerticalAlign(Legend.VerticalAlign.TOP) .setX(-10) .setY(100) .setBorderWidth(0); chart.setLegend(legend); chart.addSeries(chart.createSeries() .setName("Tokyo") .setPoints(new Number[] { 7.0, 6.9, 9.5, 14.5, 18.2, 21.5, 25.2, 26.5, 23.3, 18.3, 13.9, 9.6 }) ); chart.addSeries(chart.createSeries() .setName("New York") .setPoints(new Number[] { -0.2, 0.8, 5.7, 11.3, 17.0, 22.0, 24.8, 24.1, 20.1, 14.1, 8.6, 2.5 }) ); chart.addSeries(chart.createSeries() .setName("Berlin") .setPoints(new Number[] { -0.9, 0.6, 3.5, 8.4, 13.5, 17.0, 18.6, 17.9, 14.3, 9.0, 3.9, 1.0 }) ); chart.addSeries(chart.createSeries() .setName("London") .setPoints(new Number[] { 3.9, 4.2, 5.7, 8.5, 11.9, 15.2, 17.0, 16.6, 14.2, 10.3, 6.6, 4.8 }) ); RootPanel.get().add(chart); } }
结果
验证结果。
