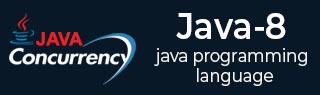
- Java并发教程
- 并发 - 主页
- 并发 - 概述
- 并发 - 环境设置
- 并发-主要操作
- 线程间通信
- 并发-同步
- 并发-死锁
- 实用程序类示例
- 并发-ThreadLocal
- 并发 - ThreadLocalRandom
- 锁示例
- 并发-锁
- 并发-ReadWriteLock
- 并发-条件
- 原子变量示例
- 并发-AtomicInteger
- 并发-AtomicLong
- 并发 - AtomicBoolean
- 并发 - AtomicReference
- 并发 - AtomicIntegerArray
- 并发-AtomicLongArray
- 并发 - AtomicReferenceArray
- 执行器示例
- 并发-执行器
- 并发-ExecutorService
- 预定执行服务
- 线程池示例
- 并发-newFixedThreadPool
- 并发-newCachedThreadPool
- 新的调度线程池
- 新的单线程执行器
- 并发-ThreadPoolExecutor
- 调度线程池执行器
- 高级示例
- 并发 - Futures 和 Callables
- 并发 - Fork-Join 框架
- 并发集合
- 并发-BlockingQueue
- 并发 - ConcurrentMap
- 并发导航地图
- 并发有用的资源
- 并发 - 快速指南
- 并发 - 有用的资源
- 并发 - 讨论
Java并发-锁接口
java.util.concurrent.locks.Lock 接口用作类似于同步块的线程同步机制。新的锁定机制比同步块更灵活,并提供更多选项。锁和同步块之间的主要区别如下:
顺序保证- 同步块不提供任何等待线程访问顺序的保证。锁接口处理它。
无超时- 如果未授予锁定,则同步块没有超时选项。锁定界面提供了这样的选项。
单一方法- 同步块必须完全包含在单一方法中,而锁接口的方法lock()和unlock()可以在不同的方法中调用。
锁定方法
以下是 Lock 类中可用的重要方法的列表。
先生。 | 方法及说明 |
---|---|
1 | 公共无效锁() 获取锁。 |
2 | 公共无效锁可中断() 除非当前线程被中断,否则获取锁。 |
3 | 公共条件 newCondition() 返回绑定到此 Lock 实例的新 Condition 实例。 |
4 | 公共布尔tryLock() 仅当调用时锁空闲时才获取锁。 |
5 | public boolean tryLock(长时间, TimeUnit 单位) 如果在给定的等待时间内锁是空闲的并且当前线程没有被中断,则获取锁。 |
6 | 公共无效解锁() 释放锁。 |
例子
下面的 TestThread 程序演示了 Lock 接口的一些方法。这里我们使用lock()来获取锁,使用unlock()来释放锁。
现场演示import java.util.concurrent.locks.Lock; import java.util.concurrent.locks.ReentrantLock; class PrintDemo { private final Lock queueLock = new ReentrantLock(); public void print() { queueLock.lock(); try { Long duration = (long) (Math.random() * 10000); System.out.println(Thread.currentThread().getName() + " Time Taken " + (duration / 1000) + " seconds."); Thread.sleep(duration); } catch (InterruptedException e) { e.printStackTrace(); } finally { System.out.printf( "%s printed the document successfully.\n", Thread.currentThread().getName()); queueLock.unlock(); } } } class ThreadDemo extends Thread { PrintDemo printDemo; ThreadDemo(String name, PrintDemo printDemo) { super(name); this.printDemo = printDemo; } @Override public void run() { System.out.printf( "%s starts printing a document\n", Thread.currentThread().getName()); printDemo.print(); } } public class TestThread { public static void main(String args[]) { PrintDemo PD = new PrintDemo(); ThreadDemo t1 = new ThreadDemo("Thread - 1 ", PD); ThreadDemo t2 = new ThreadDemo("Thread - 2 ", PD); ThreadDemo t3 = new ThreadDemo("Thread - 3 ", PD); ThreadDemo t4 = new ThreadDemo("Thread - 4 ", PD); t1.start(); t2.start(); t3.start(); t4.start(); } }
这将产生以下结果。
输出
Thread - 1 starts printing a document Thread - 4 starts printing a document Thread - 3 starts printing a document Thread - 2 starts printing a document Thread - 1 Time Taken 4 seconds. Thread - 1 printed the document successfully. Thread - 4 Time Taken 3 seconds. Thread - 4 printed the document successfully. Thread - 3 Time Taken 5 seconds. Thread - 3 printed the document successfully. Thread - 2 Time Taken 4 seconds. Thread - 2 printed the document successfully.
我们在这里使用 ReentrantLock 类作为 Lock 接口的实现。ReentrantLock 类允许线程锁定一个方法,即使它已经拥有其他方法的锁定。