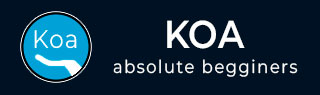
- Koa.js 教程
- Koa.js - 主页
- Koa.js - 概述
- Koa.js - 环境
- Koa.js - 你好世界
- Koa.js - 生成器
- Koa.js - 路由
- Koa.js - URL 构建
- Koa.js - HTTP 方法
- Koa.js - 请求对象
- Koa.js - 响应对象
- Koa.js - 重定向
- Koa.js - 错误处理
- Koa.js - 级联
- Koa.js - 模板
- Koa.js - 表单数据
- Koa.js - 文件上传
- Koa.js - 静态文件
- Koa.js - Cookie
- Koa.js - 会话
- Koa.js - 身份验证
- Koa.js - 压缩
- Koa.js - 缓存
- Koa.js - 数据库
- Koa.js - RESTful API
- Koa.js - 日志记录
- Koa.js - 脚手架
- Koa.js - 资源
- Koa.js 有用资源
- Koa.js - 快速指南
- Koa.js - 有用的资源
- Koa.js - 讨论
Koa.js - 身份验证
身份验证是一个过程,其中将所提供的凭据与本地操作系统或身份验证服务器内的授权用户信息数据库中的文件进行比较。如果凭据匹配,则该过程完成,并且用户被授予访问授权。
我们将创建一个非常基本的身份验证系统,该系统将使用基本 HTTP 身份验证。这是实施访问控制的最简单的方法,因为它不需要 cookie、会话或其他任何东西。要使用此功能,客户端必须将授权标头与其发出的每个请求一起发送。用户名和密码未加密,而是连接在单个字符串中,如下所示。
username:password
该字符串使用 Base64 编码,并且单词 Basic 放在该值之前。例如,如果您的用户名是 Ayush,密码 India,则字符串“Ayush:India”将按照授权标头中的编码形式发送。
Authorization: Basic QXl1c2g6SW5kaWE=
要在您的 koa 应用程序中实现此功能,您需要 koa-basic-auth 中间件。使用以下命令安装它 -
$ npm install --save koa-basic-auth
现在打开您的 app.js 文件并在其中输入以下代码。
//This is what the authentication would be checked against var credentials = { name: 'Ayush', pass: 'India' } var koa = require('koa'); var auth = require('koa-basic-auth'); var _ = require('koa-router')(); var app = koa(); //Error handling middleware app.use(function *(next){ try { yield next; } catch (err) { if (401 == err.status) { this.status = 401; this.set('WWW-Authenticate', 'Basic'); this.body = 'You have no access here'; } else { throw err; } } }); // Set up authentication here as first middleware. // This returns an error if user is not authenticated. _.get('/protected', auth(credentials), function *(){ this.body = 'You have access to the protected area.'; yield next; }); // No authentication middleware present here. _.get('/unprotected', function*(next){ this.body = "Anyone can access this area"; yield next; }); app.use(_.routes()); app.listen(3000);
我们创建了一个错误处理中间件来处理所有与身份验证相关的错误。然后,我们创建了 2 条路线 -
/protected - 仅当用户发送正确的身份验证标头时才能访问此路由。对于所有其他人,它会给出错误。
/unprotected - 任何人都可以访问此路由,无论是否经过身份验证。
现在,如果您在没有身份验证标头或使用错误凭据的情况下向 /protected 发送请求,您将收到错误。例如,
$ curl https://localhost:3000/protected
您将收到如下响应:
HTTP/1.1 401 Unauthorized WWW-Authenticate: Basic Content-Type: text/plain; charset=utf-8 Content-Length: 28 Date: Sat, 17 Sep 2016 19:05:56 GMT Connection: keep-alive Please authenticate yourself
但是,只要有正确的凭据,您就会得到预期的响应。例如,
$ curl -H "Authorization: basic QXl1c2g6SW5kaWE=" https://localhost:3000/protected -i
您将得到如下响应:
HTTP/1.1 200 OK Content-Type: text/plain; charset=utf-8 Content-Length: 38 Date: Sat, 17 Sep 2016 19:07:33 GMT Connection: keep-alive You have access to the protected area.
/unprotected 路由仍然可供所有人访问。