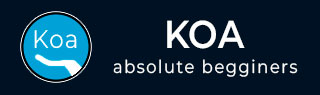
- Koa.js 教程
- Koa.js - 主页
- Koa.js - 概述
- Koa.js - 环境
- Koa.js - 你好世界
- Koa.js - 生成器
- Koa.js - 路由
- Koa.js - URL 构建
- Koa.js - HTTP 方法
- Koa.js - 请求对象
- Koa.js - 响应对象
- Koa.js - 重定向
- Koa.js - 错误处理
- Koa.js - 级联
- Koa.js - 模板
- Koa.js - 表单数据
- Koa.js - 文件上传
- Koa.js - 静态文件
- Koa.js - Cookie
- Koa.js - 会话
- Koa.js - 身份验证
- Koa.js - 压缩
- Koa.js - 缓存
- Koa.js - 数据库
- Koa.js - RESTful API
- Koa.js - 日志记录
- Koa.js - 脚手架
- Koa.js - 资源
- Koa.js 有用资源
- Koa.js - 快速指南
- Koa.js - 有用的资源
- Koa.js - 讨论
Koa.js - 路由
Web 框架以不同的路径提供 HTML 页面、脚本、图像等资源。Koa 核心模块不支持路由。我们需要使用 Koa-router 模块来轻松地在 Koa 中创建路由。使用以下命令安装此模块。
npm install --save koa-router
现在我们已经安装了 Koa-router,让我们看一个简单的 GET 路由示例。
var koa = require('koa'); var router = require('koa-router'); var app = koa(); var _ = router(); //Instantiate the router _.get('/hello', getMessage); // Define routes function *getMessage() { this.body = "Hello world!"; }; app.use(_.routes()); //Use the routes defined using the router app.listen(3000);
如果我们运行应用程序并转到 localhost:3000/hello,服务器会在路由“/hello”处收到一个 get 请求。我们的 Koa 应用程序执行附加到该路由的回调函数并发送“Hello World!” 作为回应。

我们还可以在同一条路线上有多种不同的方法。例如,
var koa = require('koa'); var router = require('koa-router'); var app = koa(); var _ = router(); //Instantiate the router _.get('/hello', getMessage); _.post('/hello', postMessage); function *getMessage() { this.body = "Hello world!"; }; function *postMessage() { this.body = "You just called the post method at '/hello'!\n"; }; app.use(_.routes()); //Use the routes defined using the router app.listen(3000);
要测试此请求,请打开终端并使用 cURL 执行以下请求
curl -X POST "https://localhost:3000/hello"

Express 提供了一种特殊方法all ,用于使用相同的函数处理特定路由上的所有类型的 http 方法。要使用此方法,请尝试以下操作 -
_.all('/test', allMessage); function *allMessage(){ this.body = "All HTTP calls regardless of the verb will get this response"; };