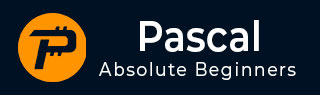
- 帕斯卡教程
- 帕斯卡 - 主页
- 帕斯卡 - 概述
- Pascal - 环境设置
- 帕斯卡 - 程序结构
- Pascal - 基本语法
- Pascal - 数据类型
- Pascal - 变量类型
- 帕斯卡 - 常数
- 帕斯卡 - 运算符
- 帕斯卡 - 决策
- 帕斯卡 - 循环
- 帕斯卡 - 函数
- 帕斯卡 - 程序
- Pascal - 变量作用域
- 帕斯卡 - 弦乐
- 帕斯卡 - 布尔
- 帕斯卡 - 数组
- 帕斯卡 - 指针
- 帕斯卡 - 记录
- 帕斯卡 - 变体
- 帕斯卡 - 集合
- 帕斯卡 - 文件处理
- 帕斯卡 - 记忆
- 帕斯卡 - 单位
- 帕斯卡 - 日期和时间
- 帕斯卡 - 对象
- 帕斯卡 - 类
- 帕斯卡有用资源
- 帕斯卡 - 快速指南
- 帕斯卡 - 有用的资源
- 帕斯卡 - 讨论
Pascal - if then else 语句
if -then语句后面可以跟一个可选的else语句,该语句在布尔表达式为false时执行。
句法
if-then-else 语句的语法是 -
if condition then S1 else S2;
其中,S1和S2是不同的语句。请注意,语句 S1 后面没有分号。if-then-else语句中,当测试条件为真时,执行语句S1,跳过S2;当测试条件为假时,则绕过 S1 并执行语句 S2。
例如,
if color = red then writeln('You have chosen a red car') else writeln('Please choose a color for your car');
如果布尔表达式条件的计算结果为 true,则将执行 if-then 代码块,否则将执行 else 代码块。
Pascal 假定任何非零和非零值都为 true,如果为零或 nil,则假定为 false 值。
流程图

例子
让我们尝试一个完整的例子来说明这个概念 -
program ifelseChecking; var { local variable definition } a : integer; begin a := 100; (* check the boolean condition *) if( a < 20 ) then (* if condition is true then print the following *) writeln('a is less than 20' ) else (* if condition is false then print the following *) writeln('a is not less than 20' ); writeln('value of a is : ', a); end.
当上面的代码被编译并执行时,它会产生以下结果 -
a is not less than 20 value of a is : 100
if-then-else if-then-else 语句
if-then 语句后面可以跟一个可选的 else if-then-else 语句,这对于使用单个 if-then-else if 语句测试各种条件非常有用。
使用 if-then 、 else if-then 、 else 语句时,有几点需要记住。
if-then 语句可以有零个或一个 else,并且它必须位于任何 else if 之后。
if-then 语句可以有零到多个 else if,并且它们必须位于 else 之前。
一旦 else if 成功,则不会测试其余的 else if 或 else。
最后一个 else 关键字之前没有给出分号 (;),但所有语句都可以是复合语句。
句法
Pascal 编程语言中 if-then-else if-then-else 语句的语法为 -
if(boolean_expression 1)then S1 (* Executes when the boolean expression 1 is true *) else if( boolean_expression 2) then S2 (* Executes when the boolean expression 2 is true *) else if( boolean_expression 3) then S3 (* Executes when the boolean expression 3 is true *) else S4; ( * executes when the none of the above condition is true *)
例子
下面的例子说明了这个概念 -
program ifelse_ifelseChecking; var { local variable definition } a : integer; begin a := 100; (* check the boolean condition *) if (a = 10) then (* if condition is true then print the following *) writeln('Value of a is 10' ) else if ( a = 20 ) then (* if else if condition is true *) writeln('Value of a is 20' ) else if( a = 30 ) then (* if else if condition is true *) writeln('Value of a is 30' ) else (* if none of the conditions is true *) writeln('None of the values is matching' ); writeln('Exact value of a is: ', a ); end.
当上面的代码被编译并执行时,它会产生以下结果 -
None of the values is matching Exact value of a is: 100
pascal_decision_making.htm