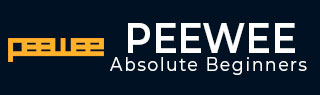
- Peewee教程
- Peewee - 主页
- Peewee - 概述
- Peewee - 数据库类
- Peewee - 模型
- Peewee - 野外课程
- Peewee - 插入新记录
- Peewee - 选择记录
- Peewee - 过滤器
- Peewee - 主键和复合键
- Peewee - 更新现有记录
- Peewee - 删除记录
- Peewee - 创建索引
- Peewee - 约束
- Peewee - 使用 MySQL
- Peewee - 使用 PostgreSQL
- Peewee - 动态定义数据库
- Peewee - 连接管理
- Peewee - 关系与加入
- Peewee - 子查询
- Peewee - 排序
- Peewee - 计数和聚合
- Peewee - SQL 函数
- Peewee - 检索行元组/字典
- Peewee - 用户定义的运算符
- Peewee - 原子事务
- Peewee - 数据库错误
- Peewee - 查询生成器
- Peewee - 与 Web 框架集成
- Peewee - SQLite 扩展
- Peewee - PostgreSQL 和 MySQL 扩展
- Peewee - 使用 CockroachDB
- Peewee有用资源
- Peewee - 快速指南
- Peewee - 有用的资源
- Peewee - 讨论
Peewee - 连接管理
默认情况下,使用设置为 True的自动连接参数创建数据库对象。相反,为了以编程方式管理数据库连接,它最初设置为 False。
db=SqliteDatabase("mydatabase", autoconnect=False)
数据库类具有connect()方法,可与服务器上存在的数据库建立连接。
db.connect()
始终建议在执行的操作结束时关闭连接。
db.close()
如果您尝试打开一个已经打开的连接,Peewee 会引发OperationError。
>>> db.connect() True >>> db.connect() Traceback (most recent call last): File "<stdin>", line 1, in <module> File "c:\peewee\lib\site-packages\peewee.py", line 3031, in connect raise OperationalError('Connection already opened.') peewee.OperationalError: Connection already opened.
为了避免此错误,请使用reuse_if_open=True作为connect()方法的参数。
>>> db.connect(reuse_if_open=True) False
在已经关闭的连接上调用close()不会导致错误。但是,您可以使用is_close()方法检查连接是否已关闭。
>>> if db.is_closed()==True: db.connect() True >>>
也可以使用数据库对象作为context_manager,而不是最终显式调用 db.close() 。
from peewee import * db = SqliteDatabase('mydatabase.db', autoconnect=False) class User (Model): user_id=TextField(primary_key=True) name=TextField() age=IntegerField() class Meta: database=db db_table='User' with db: db.connect() db.create_tables([User])