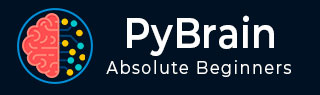
- PyBrain 教程
- PyBrain - 主页
- PyBrain - 概述
- PyBrain - 环境设置
- PyBrain - PyBrain 网络简介
- PyBrain - 使用网络
- PyBrain - 使用数据集
- PyBrain - 数据集类型
- PyBrain - 导入数据集的数据
- PyBrain - 网络训练数据集
- PyBrain - 测试网络
- 使用前馈网络
- PyBrain - 使用循环网络
- 使用优化算法训练网络
- PyBrain - 层
- PyBrain - 连接
- PyBrain - 强化学习模块
- PyBrain - API 和工具
- PyBrain - 示例
- PyBrain 有用资源
- PyBrain - 快速指南
- PyBrain - 有用的资源
- PyBrain - 讨论
PyBrain - 层
层基本上是一组用于网络隐藏层的函数。
我们将在本章中详细介绍有关层的以下详细信息 -
- 理解层
- 使用 Pybrain 创建图层
了解图层
我们之前已经看到过使用图层的示例,如下所示 -
- Tanh层
- Softmax层
使用 TanhLayer 的示例
下面是我们使用 TanhLayer 构建网络的一个示例 -
测试网络.py
from pybrain.tools.shortcuts import buildNetwork from pybrain.structure import TanhLayer from pybrain.datasets import SupervisedDataSet from pybrain.supervised.trainers import BackpropTrainer # Create a network with two inputs, three hidden, and one output nn = buildNetwork(2, 3, 1, bias=True, hiddenclass=TanhLayer) # Create a dataset that matches network input and output sizes: norgate = SupervisedDataSet(2, 1) # Create a dataset to be used for testing. nortrain = SupervisedDataSet(2, 1) # Add input and target values to dataset # Values for NOR truth table norgate.addSample((0, 0), (1,)) norgate.addSample((0, 1), (0,)) norgate.addSample((1, 0), (0,)) norgate.addSample((1, 1), (0,)) # Add input and target values to dataset # Values for NOR truth table nortrain.addSample((0, 0), (1,)) nortrain.addSample((0, 1), (0,)) nortrain.addSample((1, 0), (0,)) nortrain.addSample((1, 1), (0,)) #Training the network with dataset norgate. trainer = BackpropTrainer(nn, norgate) # will run the loop 1000 times to train it. for epoch in range(1000): trainer.train() trainer.testOnData(dataset=nortrain, verbose = True)
输出
上述代码的输出如下 -
python 测试网络.py
C:\pybrain\pybrain\src>python testnetwork.py Testing on data: ('out: ', '[0.887 ]') ('correct:', '[1 ]') error: 0.00637334 ('out: ', '[0.149 ]') ('correct:', '[0 ]') error: 0.01110338 ('out: ', '[0.102 ]') ('correct:', '[0 ]') error: 0.00522736 ('out: ', '[-0.163]') ('correct:', '[0 ]') error: 0.01328650 ('All errors:', [0.006373344564625953, 0.01110338071737218, 0.005227359234093431, 0.01328649974219942]) ('Average error:', 0.008997646064572746) ('Max error:', 0.01328649974219942, 'Median error:', 0.01110338071737218)
使用 SoftMaxLayer 的示例
下面是我们使用 SoftmaxLayer 构建网络的一个示例 -
from pybrain.tools.shortcuts import buildNetwork from pybrain.structure.modules import SoftmaxLayer from pybrain.datasets import SupervisedDataSet from pybrain.supervised.trainers import BackpropTrainer # Create a network with two inputs, three hidden, and one output nn = buildNetwork(2, 3, 1, bias=True, hiddenclass=SoftmaxLayer) # Create a dataset that matches network input and output sizes: norgate = SupervisedDataSet(2, 1) # Create a dataset to be used for testing. nortrain = SupervisedDataSet(2, 1) # Add input and target values to dataset # Values for NOR truth table norgate.addSample((0, 0), (1,)) norgate.addSample((0, 1), (0,)) norgate.addSample((1, 0), (0,)) norgate.addSample((1, 1), (0,)) # Add input and target values to dataset # Values for NOR truth table nortrain.addSample((0, 0), (1,)) nortrain.addSample((0, 1), (0,)) nortrain.addSample((1, 0), (0,)) nortrain.addSample((1, 1), (0,)) #Training the network with dataset norgate. trainer = BackpropTrainer(nn, norgate) # will run the loop 1000 times to train it. for epoch in range(1000): trainer.train() trainer.testOnData(dataset=nortrain, verbose = True)
输出
输出如下 -
C:\pybrain\pybrain\src>python example16.py Testing on data: ('out: ', '[0.918 ]') ('correct:', '[1 ]') error: 0.00333524 ('out: ', '[0.082 ]') ('correct:', '[0 ]') error: 0.00333484 ('out: ', '[0.078 ]') ('correct:', '[0 ]') error: 0.00303433 ('out: ', '[-0.082]') ('correct:', '[0 ]') error: 0.00340005 ('All errors:', [0.0033352368788838365, 0.003334842961037291, 0.003034328685718761, 0.0034000458892589056]) ('Average error:', 0.0032761136037246985) ('Max error:', 0.0034000458892589056, 'Median error:', 0.0033352368788838365)
在 Pybrain 中创建层
在 Pybrain 中,您可以创建自己的层,如下所示 -
要创建图层,您需要使用NeuronLayer 类作为基类来创建所有类型的图层。
例子
from pybrain.structure.modules.neuronlayer import NeuronLayer class LinearLayer(NeuronLayer): def _forwardImplementation(self, inbuf, outbuf): outbuf[:] = inbuf def _backwardImplementation(self, outerr, inerr, outbuf, inbuf): inerr[:] = outer
要创建一个 Layer,我们需要实现两个方法:_forwardImplementation()和_backwardImplementation()。
_forwardImplementation() 接受 2 个参数 inbuf和 outbuf,它们是 Scipy 数组。它的大小取决于层的输入和输出尺寸。
_backwardImplementation ()用于计算输出相对于给定输入的导数。
因此,要在 Pybrain 中实现图层,这是图层类的骨架 -
from pybrain.structure.modules.neuronlayer import NeuronLayer class NewLayer(NeuronLayer): def _forwardImplementation(self, inbuf, outbuf): pass def _backwardImplementation(self, outerr, inerr, outbuf, inbuf): pass
如果您想将二次多项式函数实现为一个层,我们可以这样做 -
考虑我们有一个多项式函数 -
f(x) = 3x2
上述多项式函数的导数如下 -
f(x) = 6 x
上述多项式函数的最终层类如下 -
测试层.py
from pybrain.structure.modules.neuronlayer import NeuronLayer class PolynomialLayer(NeuronLayer): def _forwardImplementation(self, inbuf, outbuf): outbuf[:] = 3*inbuf**2 def _backwardImplementation(self, outerr, inerr, outbuf, inbuf): inerr[:] = 6*inbuf*outerr
现在让我们利用创建的图层,如下所示 -
测试层1.py
from testlayer import PolynomialLayer from pybrain.tools.shortcuts import buildNetwork from pybrain.tests.helpers import gradientCheck n = buildNetwork(2, 3, 1, hiddenclass=PolynomialLayer) n.randomize() gradientCheck(n)
GradientCheck()将测试该层是否工作正常。我们需要将使用该层的网络传递给gradientCheck(n)。如果该层工作正常,它将给出“完美梯度”的输出。
输出
C:\pybrain\pybrain\src>python testlayer1.py Perfect gradient