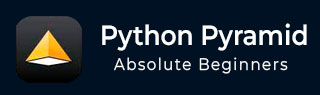
- Python Pyramid教程
- Python Pyramid - 主页
- Python Pyramid - 概述
- Pyramid - 环境设置
- Python Pyramid - Hello World
- Pyramid - 应用程序配置
- Python Pyramid - URL 路由
- Python Pyramid - 查看配置
- Python Pyramid - 路由前缀
- Python Pyramid - 模板
- Pyramid - HTML 表单模板
- Python Pyramid - 静态资源
- Python Pyramid - 请求对象
- Python Pyramid - 响应对象
- Python Pyramid - 会话
- Python Pyramid - 事件
- Python Pyramid - 消息闪烁
- Pyramid - 使用 SQLAlchemy
- Python Pyramid - Cookiecutter
- Python Pyramid - 创建项目
- Python Pyramid - 项目结构
- Python Pyramid - 包结构
- 手动创建项目
- 命令行Pyramid
- Python Pyramid - 测试
- Python Pyramid - 日志记录
- Python Pyramid - 安全
- Python Pyramid - 部署
- Python Pyramid有用资源
- Python Pyramid - 快速指南
- Python Pyramid - 有用的资源
- Python Pyramid - 讨论
Python Pyramid - 路由前缀
很多时候,相似的 URL 模式会在多个 Python 代码模块中注册不同的路由。例如,我们有一个Student_routes.py,其中 /list 和 /add URL 模式注册为“list”和“add”路由。与这些路由关联的视图函数分别是list()和add()。
#student_routes.py from pyramid.config import Configurator from pyramid.response import Response from pyramid.view import view_config @view_config( route_name='add') def add(request): return Response('add student') @view_config(route_name='list') def list(request): return Response('Student list') def students(config): config.add_route('list', '/list') config.add_route('add', '/add') config.scan()
这些路由最终将在调用Students()函数时注册。
同时,还有 book_routes.py,其中相同的 URL /list和add/被注册到 'show' 和 'new' 路由。它们的关联视图分别是list()和add()。该模块有 books() 函数,可以添加路线。
#book_routes.py from pyramid.config import Configurator from pyramid.response import Response from pyramid.view import view_config @view_config( route_name='new') def add(request): return Response('add book') @view_config(route_name='show') def list(request): return Response('Book list') def books(config): config.add_route('show', '/list') config.add_route('new', '/add') config.scan()
显然,URL 模式之间存在冲突,因为“/list”和“/add”分别指向两个路由,必须解决此冲突。这是通过使用config.include()方法的route_prefix参数来完成的。
config.include() 的第一个参数是添加路由的函数,第二个参数是route_prefix 字符串,它将被添加到包含函数中使用的 URL 模式之前。
因此,声明
config.include(students, route_prefix='/student')
将导致“/list”URL 模式更改为“/student/list”,“/add”变为“student/add”。同样,我们可以在 books() 函数中为这些 URL 模式添加前缀。
config.include(books, route_prefix='/books')
例子
启动服务器的代码如下 -
from wsgiref.simple_server import make_server from pyramid.config import Configurator from pyramid.response import Response from student_routes import students from book_routes import books if __name__ == '__main__': with Configurator() as config: config.include(students, route_prefix='/student') config.include(books, route_prefix='/book') app = config.make_wsgi_app() server = make_server('0.0.0.0', 6543, app) server.serve_forever()
输出
让我们运行上面的代码并通过以下 CURL 命令测试路由。
C:\Users\Acer>curl localhost:6543/student/list Student list C:\Users\Acer>curl localhost:6543/student/add add student C:\Users\Acer>curl localhost:6543/book/add add book C:\Users\Acer>curl localhost:6543/book/list Book list