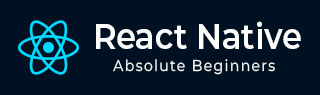
- React Native 教程
- React Native - 主页
- 核心概念
- React Native - 概述
- React Native - 环境设置
- React Native - 应用程序
- React Native - 状态
- React Native - 道具
- React Native - 样式
- React Native - Flexbox
- React Native - 列表视图
- React Native - 文本输入
- React Native - 滚动视图
- React Native - 图像
- 反应本机 - HTTP
- React Native - 按钮
- React Native - 动画
- React Native - 调试
- React Native - 路由器
- React Native - 运行 IOS
- React Native - 运行 Android
- 组件和 API
- React Native - 查看
- React Native - WebView
- React Native - 模态
- React Native - 活动指示器
- React Native - 选择器
- React Native - 状态栏
- React Native - 开关
- React Native - 文本
- React Native - 警报
- React Native - 地理定位
- React Native - 异步存储
- React Native 有用资源
- React Native - 快速指南
- React Native - 有用的资源
- React Native - 讨论
React Native - 动画
在本章中,我们将向您展示如何在 React Native 中使用LayoutAnimation。
动画组件
我们将把myStyle设置为状态的一个属性。此属性用于为PresentationalAnimationComponent内的元素设置样式。
我们还将创建两个函数 - ExpandElement和CollapseElement。这些函数将更新状态值。第一个将使用弹簧预设动画,而第二个将使用线性预设。我们也将把它们作为道具传递。Expand和Collapse按钮调用expandElement()和collapseElement ( )函数。
在此示例中,我们将动态更改框的宽度和高度。由于Home组件是相同的,我们将只更改Animations组件。
应用程序.js
import React, { Component } from 'react' import { View, StyleSheet, Animated, TouchableOpacity } from 'react-native' class Animations extends Component { componentWillMount = () => { this.animatedWidth = new Animated.Value(50) this.animatedHeight = new Animated.Value(100) } animatedBox = () => { Animated.timing(this.animatedWidth, { toValue: 200, duration: 1000 }).start() Animated.timing(this.animatedHeight, { toValue: 500, duration: 500 }).start() } render() { const animatedStyle = { width: this.animatedWidth, height: this.animatedHeight } return ( <TouchableOpacity style = {styles.container} onPress = {this.animatedBox}> <Animated.View style = {[styles.box, animatedStyle]}/> </TouchableOpacity> ) } } export default Animations const styles = StyleSheet.create({ container: { justifyContent: 'center', alignItems: 'center' }, box: { backgroundColor: 'blue', width: 50, height: 100 } })