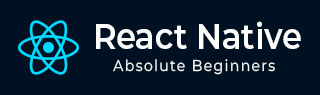
- React Native 教程
- React Native - 主页
- 核心概念
- React Native - 概述
- React Native - 环境设置
- React Native - 应用程序
- React Native - 状态
- React Native - 道具
- React Native - 样式
- React Native - Flexbox
- React Native - 列表视图
- React Native - 文本输入
- React Native - 滚动视图
- React Native - 图像
- 反应本机 - HTTP
- React Native - 按钮
- React Native - 动画
- React Native - 调试
- React Native - 路由器
- React Native - 运行 IOS
- React Native - 运行 Android
- 组件和 API
- React Native - 查看
- React Native - WebView
- React Native - 模态
- React Native - 活动指示器
- React Native - 选择器
- React Native - 状态栏
- React Native - 开关
- React Native - 文本
- React Native - 警报
- React Native - 地理定位
- React Native - 异步存储
- React Native 有用资源
- React Native - 快速指南
- React Native - 有用的资源
- React Native - 讨论
React Native - 按钮
在本章中,我们将向您展示 React Native 中的可触摸组件。我们称它们为“可触摸”,因为它们提供内置动画,我们可以使用onPress属性来处理触摸事件。
Facebook 提供了Button组件,它可以用作通用按钮。请考虑以下示例来理解这一点。
应用程序.js
import React, { Component } from 'react' import { Button } from 'react-native' const App = () => { const handlePress = () => false return ( <Button onPress = {handlePress} title = "Red button!" color = "red" /> ) } export default App
如果默认的Button组件不能满足您的需求,您可以使用以下组件之一代替。

可触摸的不透明度
当触摸该元素时,该元素将改变元素的不透明度。
应用程序.js
import React from 'react' import { TouchableOpacity, StyleSheet, View, Text } from 'react-native' const App = () => { return ( <View style = {styles.container}> <TouchableOpacity> <Text style = {styles.text}> Button </Text> </TouchableOpacity> </View> ) } export default App const styles = StyleSheet.create ({ container: { alignItems: 'center', }, text: { borderWidth: 1, padding: 25, borderColor: 'black', backgroundColor: 'red' } })

可触摸亮点
当用户按下该元素时,它会变暗,并且底层颜色将显示出来。
应用程序.js
import React from 'react' import { View, TouchableHighlight, Text, StyleSheet } from 'react-native' const App = (props) => { return ( <View style = {styles.container}> <TouchableHighlight> <Text style = {styles.text}> Button </Text> </TouchableHighlight> </View> ) } export default App const styles = StyleSheet.create ({ container: { alignItems: 'center', }, text: { borderWidth: 1, padding: 25, borderColor: 'black', backgroundColor: 'red' } })
可触摸的原生反馈
这将在按下元素时模拟墨迹动画。
应用程序.js
import React from 'react' import { View, TouchableNativeFeedback, Text, StyleSheet } from 'react-native' const Home = (props) => { return ( <View style = {styles.container}> <TouchableNativeFeedback> <Text style = {styles.text}> Button </Text> </TouchableNativeFeedback> </View> ) } export default Home const styles = StyleSheet.create ({ container: { alignItems: 'center', }, text: { borderWidth: 1, padding: 25, borderColor: 'black', backgroundColor: 'red' } })
无反馈可触摸
当你想要处理没有任何动画的触摸事件时应该使用这个组件。通常,这个组件使用得不多。
<TouchableWithoutFeedback> <Text> Button </Text> </TouchableWithoutFeedback>